Multiple forms in forms.py
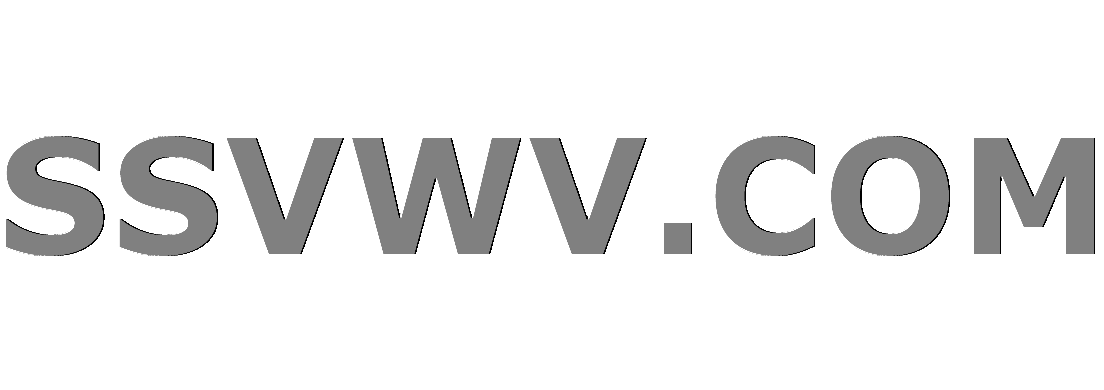
Multi tool use
This is a Django project.
forms.py
class BigForm(forms.Form):
template = forms.CharField(label='Template', widget=forms.Select(choices=CHOICES))
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.helper = FormHelper
self.helper.form_method = 'post'
self.helper.layout = Layout(
Field('template'),
Submit('submit', 'Submit', css_class='btn-success')
)
class DateForm(forms.Form):
start_date = forms.CharField(label='Start date')
end_date = forms.CharField(label='End date')
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.helper = FormHelper
self.helper.form_method = 'post'
self.helper.layout = Layout(
Field('start_date', css_class='form-control'),
Field('end_date', css_class='form-control')
)
views.py
def myForm(request):
main_form = BigForm()
date_form = DateForm()
return render(request, 'polls/main.html', {'main_form': main_form, 'date_form': date_form})
Is there something wrong with this? I keep getting
KeyError: "Key 'end_date' not found in 'BigForm'. Choices are: template."
I just want two separate form classes (for two separate forms)
python django python-3.x
add a comment |
This is a Django project.
forms.py
class BigForm(forms.Form):
template = forms.CharField(label='Template', widget=forms.Select(choices=CHOICES))
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.helper = FormHelper
self.helper.form_method = 'post'
self.helper.layout = Layout(
Field('template'),
Submit('submit', 'Submit', css_class='btn-success')
)
class DateForm(forms.Form):
start_date = forms.CharField(label='Start date')
end_date = forms.CharField(label='End date')
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.helper = FormHelper
self.helper.form_method = 'post'
self.helper.layout = Layout(
Field('start_date', css_class='form-control'),
Field('end_date', css_class='form-control')
)
views.py
def myForm(request):
main_form = BigForm()
date_form = DateForm()
return render(request, 'polls/main.html', {'main_form': main_form, 'date_form': date_form})
Is there something wrong with this? I keep getting
KeyError: "Key 'end_date' not found in 'BigForm'. Choices are: template."
I just want two separate form classes (for two separate forms)
python django python-3.x
1
You are using crisply forms
– Mohit Harshan
Nov 28 '18 at 14:37
Yes I am using crispy forms
– edb500
Nov 28 '18 at 14:39
Does it work? Approve answer
– Mohit Harshan
Nov 28 '18 at 15:27
No it doesn't, I still see the error message. But never mind, I will try and ask a different question. Maybe my code/form design is wrong.
– edb500
Nov 28 '18 at 15:44
1
You should show the entire traceback, because it's not clear on which line this error is produced. I don't believe this error can occur on any of the lines of code you show here.
– dirkgroten
Nov 28 '18 at 16:49
add a comment |
This is a Django project.
forms.py
class BigForm(forms.Form):
template = forms.CharField(label='Template', widget=forms.Select(choices=CHOICES))
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.helper = FormHelper
self.helper.form_method = 'post'
self.helper.layout = Layout(
Field('template'),
Submit('submit', 'Submit', css_class='btn-success')
)
class DateForm(forms.Form):
start_date = forms.CharField(label='Start date')
end_date = forms.CharField(label='End date')
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.helper = FormHelper
self.helper.form_method = 'post'
self.helper.layout = Layout(
Field('start_date', css_class='form-control'),
Field('end_date', css_class='form-control')
)
views.py
def myForm(request):
main_form = BigForm()
date_form = DateForm()
return render(request, 'polls/main.html', {'main_form': main_form, 'date_form': date_form})
Is there something wrong with this? I keep getting
KeyError: "Key 'end_date' not found in 'BigForm'. Choices are: template."
I just want two separate form classes (for two separate forms)
python django python-3.x
This is a Django project.
forms.py
class BigForm(forms.Form):
template = forms.CharField(label='Template', widget=forms.Select(choices=CHOICES))
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.helper = FormHelper
self.helper.form_method = 'post'
self.helper.layout = Layout(
Field('template'),
Submit('submit', 'Submit', css_class='btn-success')
)
class DateForm(forms.Form):
start_date = forms.CharField(label='Start date')
end_date = forms.CharField(label='End date')
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.helper = FormHelper
self.helper.form_method = 'post'
self.helper.layout = Layout(
Field('start_date', css_class='form-control'),
Field('end_date', css_class='form-control')
)
views.py
def myForm(request):
main_form = BigForm()
date_form = DateForm()
return render(request, 'polls/main.html', {'main_form': main_form, 'date_form': date_form})
Is there something wrong with this? I keep getting
KeyError: "Key 'end_date' not found in 'BigForm'. Choices are: template."
I just want two separate form classes (for two separate forms)
python django python-3.x
python django python-3.x
asked Nov 28 '18 at 14:23
edb500edb500
73113
73113
1
You are using crisply forms
– Mohit Harshan
Nov 28 '18 at 14:37
Yes I am using crispy forms
– edb500
Nov 28 '18 at 14:39
Does it work? Approve answer
– Mohit Harshan
Nov 28 '18 at 15:27
No it doesn't, I still see the error message. But never mind, I will try and ask a different question. Maybe my code/form design is wrong.
– edb500
Nov 28 '18 at 15:44
1
You should show the entire traceback, because it's not clear on which line this error is produced. I don't believe this error can occur on any of the lines of code you show here.
– dirkgroten
Nov 28 '18 at 16:49
add a comment |
1
You are using crisply forms
– Mohit Harshan
Nov 28 '18 at 14:37
Yes I am using crispy forms
– edb500
Nov 28 '18 at 14:39
Does it work? Approve answer
– Mohit Harshan
Nov 28 '18 at 15:27
No it doesn't, I still see the error message. But never mind, I will try and ask a different question. Maybe my code/form design is wrong.
– edb500
Nov 28 '18 at 15:44
1
You should show the entire traceback, because it's not clear on which line this error is produced. I don't believe this error can occur on any of the lines of code you show here.
– dirkgroten
Nov 28 '18 at 16:49
1
1
You are using crisply forms
– Mohit Harshan
Nov 28 '18 at 14:37
You are using crisply forms
– Mohit Harshan
Nov 28 '18 at 14:37
Yes I am using crispy forms
– edb500
Nov 28 '18 at 14:39
Yes I am using crispy forms
– edb500
Nov 28 '18 at 14:39
Does it work? Approve answer
– Mohit Harshan
Nov 28 '18 at 15:27
Does it work? Approve answer
– Mohit Harshan
Nov 28 '18 at 15:27
No it doesn't, I still see the error message. But never mind, I will try and ask a different question. Maybe my code/form design is wrong.
– edb500
Nov 28 '18 at 15:44
No it doesn't, I still see the error message. But never mind, I will try and ask a different question. Maybe my code/form design is wrong.
– edb500
Nov 28 '18 at 15:44
1
1
You should show the entire traceback, because it's not clear on which line this error is produced. I don't believe this error can occur on any of the lines of code you show here.
– dirkgroten
Nov 28 '18 at 16:49
You should show the entire traceback, because it's not clear on which line this error is produced. I don't believe this error can occur on any of the lines of code you show here.
– dirkgroten
Nov 28 '18 at 16:49
add a comment |
1 Answer
1
active
oldest
votes
You have not declared end_date
as a field within BigForm
. It exists in DateForm
.
Why would I have to declare end_date in BigForm? I just want to use it in DateForm
– edb500
Nov 28 '18 at 14:39
You have indeed declaredend_date
inDateForm
, but according to the error that you posted in your question, you are getting aKeyError
looking forend_date
inBigForm
. Post the entire traceback of your error message so we can see exactly where the problem is.
– Daniel H.
Nov 29 '18 at 7:00
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53521615%2fmultiple-forms-in-forms-py%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You have not declared end_date
as a field within BigForm
. It exists in DateForm
.
Why would I have to declare end_date in BigForm? I just want to use it in DateForm
– edb500
Nov 28 '18 at 14:39
You have indeed declaredend_date
inDateForm
, but according to the error that you posted in your question, you are getting aKeyError
looking forend_date
inBigForm
. Post the entire traceback of your error message so we can see exactly where the problem is.
– Daniel H.
Nov 29 '18 at 7:00
add a comment |
You have not declared end_date
as a field within BigForm
. It exists in DateForm
.
Why would I have to declare end_date in BigForm? I just want to use it in DateForm
– edb500
Nov 28 '18 at 14:39
You have indeed declaredend_date
inDateForm
, but according to the error that you posted in your question, you are getting aKeyError
looking forend_date
inBigForm
. Post the entire traceback of your error message so we can see exactly where the problem is.
– Daniel H.
Nov 29 '18 at 7:00
add a comment |
You have not declared end_date
as a field within BigForm
. It exists in DateForm
.
You have not declared end_date
as a field within BigForm
. It exists in DateForm
.
answered Nov 28 '18 at 14:28


Daniel H.Daniel H.
420314
420314
Why would I have to declare end_date in BigForm? I just want to use it in DateForm
– edb500
Nov 28 '18 at 14:39
You have indeed declaredend_date
inDateForm
, but according to the error that you posted in your question, you are getting aKeyError
looking forend_date
inBigForm
. Post the entire traceback of your error message so we can see exactly where the problem is.
– Daniel H.
Nov 29 '18 at 7:00
add a comment |
Why would I have to declare end_date in BigForm? I just want to use it in DateForm
– edb500
Nov 28 '18 at 14:39
You have indeed declaredend_date
inDateForm
, but according to the error that you posted in your question, you are getting aKeyError
looking forend_date
inBigForm
. Post the entire traceback of your error message so we can see exactly where the problem is.
– Daniel H.
Nov 29 '18 at 7:00
Why would I have to declare end_date in BigForm? I just want to use it in DateForm
– edb500
Nov 28 '18 at 14:39
Why would I have to declare end_date in BigForm? I just want to use it in DateForm
– edb500
Nov 28 '18 at 14:39
You have indeed declared
end_date
in DateForm
, but according to the error that you posted in your question, you are getting a KeyError
looking for end_date
in BigForm
. Post the entire traceback of your error message so we can see exactly where the problem is.– Daniel H.
Nov 29 '18 at 7:00
You have indeed declared
end_date
in DateForm
, but according to the error that you posted in your question, you are getting a KeyError
looking for end_date
in BigForm
. Post the entire traceback of your error message so we can see exactly where the problem is.– Daniel H.
Nov 29 '18 at 7:00
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53521615%2fmultiple-forms-in-forms-py%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3ohLjoI1GjVd,7XFnI R76Be,G T tcm s,veSyu2ug,EL5qgQ7 pDUDwys d mUXUH26gOYMRksZ t csbFvN82Fb2z,4ZFs5W
1
You are using crisply forms
– Mohit Harshan
Nov 28 '18 at 14:37
Yes I am using crispy forms
– edb500
Nov 28 '18 at 14:39
Does it work? Approve answer
– Mohit Harshan
Nov 28 '18 at 15:27
No it doesn't, I still see the error message. But never mind, I will try and ask a different question. Maybe my code/form design is wrong.
– edb500
Nov 28 '18 at 15:44
1
You should show the entire traceback, because it's not clear on which line this error is produced. I don't believe this error can occur on any of the lines of code you show here.
– dirkgroten
Nov 28 '18 at 16:49