Passing a spinner value from one fragment to the next [duplicate]
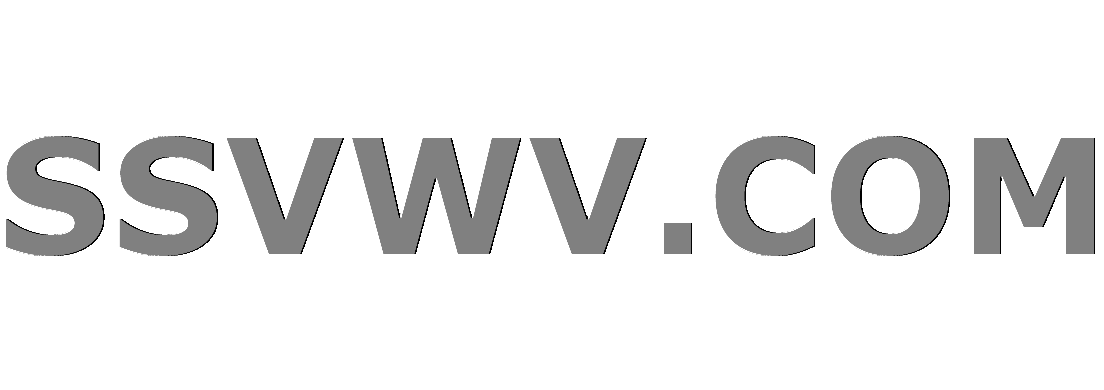
Multi tool use
This question is an exact duplicate of:
Passing a Spinner value from one fragment to another
1 answer
I need to get the value of the spinner set in the first fragment and pass the value along to fragment 2. At the moment I'm just trying to set one text field in fragment 2 to the spinner's value, but later on I'll need to somehow have the fragment 1 spinner value match a unit like meters, and then for fragment 2 to be able to take that unit and a user input to then convert it into a second chosen user input.
In fragment 2 I have just typed out some 'bad' psuedo code to showcase what I'm trying to do:
Main Activity
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
TabLayout tabLayout = (TabLayout) findViewById(R.id.tab_layout);
tabLayout.addTab(tabLayout.newTab().setText(R.string.tab_page1));
tabLayout.addTab(tabLayout.newTab().setText(R.string.tab_page2));
tabLayout.setTabGravity(TabLayout.GRAVITY_FILL);
final ViewPager viewPager = (ViewPager) findViewById(R.id.pager);
final PagerAdapter adapter = new PagerAdapter(getSupportFragmentManager(),
tabLayout.getTabCount());
viewPager.setAdapter(adapter);
viewPager.addOnPageChangeListener(new
TabLayout.TabLayoutOnPageChangeListener(tabLayout));
tabLayout.setOnTabSelectedListener(new TabLayout.OnTabSelectedListener() {
@Override
public void onTabSelected(TabLayout.Tab tab) {
viewPager.setCurrentItem(tab.getPosition());
}
@Override
public void onTabUnselected(TabLayout.Tab tab) {}
@Override
public void onTabReselected(TabLayout.Tab tab) {}
});
}
Fragment 1
public class Page1Fragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle
savedInstanceState) {
View view = inflater.inflate(R.layout.page1_fragment, container, false);
Spinner spinner = (Spinner) view.findViewById(R.id.spinner);
// Create an ArrayAdapter using the string array and a default spinner layout
ArrayAdapter<CharSequence> adapter = ArrayAdapter.createFromResource(getActivity(), R.array.planets_array, android.R.layout.simple_spinner_item);
// Specify the layout to use when the list of choices appears
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
// Apply the adapter to the spinner
spinner.setAdapter(adapter);
return view;
}
Fragment 2
public class Page2Fragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle
savedInstanceState) {
View view = inflater.inflate(R.layout.page2_fragment, container, false);
fragment1Spinner = Page1Fragment.getSpinnerValue
TextView text = (TextView) view.findViewById(R.id.textView2);
text.setText(fragment1Spinner);
return view;
}
}
java android
marked as duplicate by Mike M.
StackExchange.ready(function() {
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function() {
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function() {
$hover.showInfoMessage('', {
messageElement: $msg.clone().show(),
transient: false,
position: { my: 'bottom left', at: 'top center', offsetTop: -7 },
dismissable: false,
relativeToBody: true
});
},
function() {
StackExchange.helpers.removeMessages();
}
);
});
});
Nov 23 '18 at 14:58
This question was marked as an exact duplicate of an existing question.
add a comment |
This question is an exact duplicate of:
Passing a Spinner value from one fragment to another
1 answer
I need to get the value of the spinner set in the first fragment and pass the value along to fragment 2. At the moment I'm just trying to set one text field in fragment 2 to the spinner's value, but later on I'll need to somehow have the fragment 1 spinner value match a unit like meters, and then for fragment 2 to be able to take that unit and a user input to then convert it into a second chosen user input.
In fragment 2 I have just typed out some 'bad' psuedo code to showcase what I'm trying to do:
Main Activity
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
TabLayout tabLayout = (TabLayout) findViewById(R.id.tab_layout);
tabLayout.addTab(tabLayout.newTab().setText(R.string.tab_page1));
tabLayout.addTab(tabLayout.newTab().setText(R.string.tab_page2));
tabLayout.setTabGravity(TabLayout.GRAVITY_FILL);
final ViewPager viewPager = (ViewPager) findViewById(R.id.pager);
final PagerAdapter adapter = new PagerAdapter(getSupportFragmentManager(),
tabLayout.getTabCount());
viewPager.setAdapter(adapter);
viewPager.addOnPageChangeListener(new
TabLayout.TabLayoutOnPageChangeListener(tabLayout));
tabLayout.setOnTabSelectedListener(new TabLayout.OnTabSelectedListener() {
@Override
public void onTabSelected(TabLayout.Tab tab) {
viewPager.setCurrentItem(tab.getPosition());
}
@Override
public void onTabUnselected(TabLayout.Tab tab) {}
@Override
public void onTabReselected(TabLayout.Tab tab) {}
});
}
Fragment 1
public class Page1Fragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle
savedInstanceState) {
View view = inflater.inflate(R.layout.page1_fragment, container, false);
Spinner spinner = (Spinner) view.findViewById(R.id.spinner);
// Create an ArrayAdapter using the string array and a default spinner layout
ArrayAdapter<CharSequence> adapter = ArrayAdapter.createFromResource(getActivity(), R.array.planets_array, android.R.layout.simple_spinner_item);
// Specify the layout to use when the list of choices appears
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
// Apply the adapter to the spinner
spinner.setAdapter(adapter);
return view;
}
Fragment 2
public class Page2Fragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle
savedInstanceState) {
View view = inflater.inflate(R.layout.page2_fragment, container, false);
fragment1Spinner = Page1Fragment.getSpinnerValue
TextView text = (TextView) view.findViewById(R.id.textView2);
text.setText(fragment1Spinner);
return view;
}
}
java android
marked as duplicate by Mike M.
StackExchange.ready(function() {
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function() {
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function() {
$hover.showInfoMessage('', {
messageElement: $msg.clone().show(),
transient: false,
position: { my: 'bottom left', at: 'top center', offsetTop: -7 },
dismissable: false,
relativeToBody: true
});
},
function() {
StackExchange.helpers.removeMessages();
}
);
});
});
Nov 23 '18 at 14:58
This question was marked as an exact duplicate of an existing question.
add a comment |
This question is an exact duplicate of:
Passing a Spinner value from one fragment to another
1 answer
I need to get the value of the spinner set in the first fragment and pass the value along to fragment 2. At the moment I'm just trying to set one text field in fragment 2 to the spinner's value, but later on I'll need to somehow have the fragment 1 spinner value match a unit like meters, and then for fragment 2 to be able to take that unit and a user input to then convert it into a second chosen user input.
In fragment 2 I have just typed out some 'bad' psuedo code to showcase what I'm trying to do:
Main Activity
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
TabLayout tabLayout = (TabLayout) findViewById(R.id.tab_layout);
tabLayout.addTab(tabLayout.newTab().setText(R.string.tab_page1));
tabLayout.addTab(tabLayout.newTab().setText(R.string.tab_page2));
tabLayout.setTabGravity(TabLayout.GRAVITY_FILL);
final ViewPager viewPager = (ViewPager) findViewById(R.id.pager);
final PagerAdapter adapter = new PagerAdapter(getSupportFragmentManager(),
tabLayout.getTabCount());
viewPager.setAdapter(adapter);
viewPager.addOnPageChangeListener(new
TabLayout.TabLayoutOnPageChangeListener(tabLayout));
tabLayout.setOnTabSelectedListener(new TabLayout.OnTabSelectedListener() {
@Override
public void onTabSelected(TabLayout.Tab tab) {
viewPager.setCurrentItem(tab.getPosition());
}
@Override
public void onTabUnselected(TabLayout.Tab tab) {}
@Override
public void onTabReselected(TabLayout.Tab tab) {}
});
}
Fragment 1
public class Page1Fragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle
savedInstanceState) {
View view = inflater.inflate(R.layout.page1_fragment, container, false);
Spinner spinner = (Spinner) view.findViewById(R.id.spinner);
// Create an ArrayAdapter using the string array and a default spinner layout
ArrayAdapter<CharSequence> adapter = ArrayAdapter.createFromResource(getActivity(), R.array.planets_array, android.R.layout.simple_spinner_item);
// Specify the layout to use when the list of choices appears
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
// Apply the adapter to the spinner
spinner.setAdapter(adapter);
return view;
}
Fragment 2
public class Page2Fragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle
savedInstanceState) {
View view = inflater.inflate(R.layout.page2_fragment, container, false);
fragment1Spinner = Page1Fragment.getSpinnerValue
TextView text = (TextView) view.findViewById(R.id.textView2);
text.setText(fragment1Spinner);
return view;
}
}
java android
This question is an exact duplicate of:
Passing a Spinner value from one fragment to another
1 answer
I need to get the value of the spinner set in the first fragment and pass the value along to fragment 2. At the moment I'm just trying to set one text field in fragment 2 to the spinner's value, but later on I'll need to somehow have the fragment 1 spinner value match a unit like meters, and then for fragment 2 to be able to take that unit and a user input to then convert it into a second chosen user input.
In fragment 2 I have just typed out some 'bad' psuedo code to showcase what I'm trying to do:
Main Activity
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar);
setSupportActionBar(toolbar);
TabLayout tabLayout = (TabLayout) findViewById(R.id.tab_layout);
tabLayout.addTab(tabLayout.newTab().setText(R.string.tab_page1));
tabLayout.addTab(tabLayout.newTab().setText(R.string.tab_page2));
tabLayout.setTabGravity(TabLayout.GRAVITY_FILL);
final ViewPager viewPager = (ViewPager) findViewById(R.id.pager);
final PagerAdapter adapter = new PagerAdapter(getSupportFragmentManager(),
tabLayout.getTabCount());
viewPager.setAdapter(adapter);
viewPager.addOnPageChangeListener(new
TabLayout.TabLayoutOnPageChangeListener(tabLayout));
tabLayout.setOnTabSelectedListener(new TabLayout.OnTabSelectedListener() {
@Override
public void onTabSelected(TabLayout.Tab tab) {
viewPager.setCurrentItem(tab.getPosition());
}
@Override
public void onTabUnselected(TabLayout.Tab tab) {}
@Override
public void onTabReselected(TabLayout.Tab tab) {}
});
}
Fragment 1
public class Page1Fragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle
savedInstanceState) {
View view = inflater.inflate(R.layout.page1_fragment, container, false);
Spinner spinner = (Spinner) view.findViewById(R.id.spinner);
// Create an ArrayAdapter using the string array and a default spinner layout
ArrayAdapter<CharSequence> adapter = ArrayAdapter.createFromResource(getActivity(), R.array.planets_array, android.R.layout.simple_spinner_item);
// Specify the layout to use when the list of choices appears
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
// Apply the adapter to the spinner
spinner.setAdapter(adapter);
return view;
}
Fragment 2
public class Page2Fragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle
savedInstanceState) {
View view = inflater.inflate(R.layout.page2_fragment, container, false);
fragment1Spinner = Page1Fragment.getSpinnerValue
TextView text = (TextView) view.findViewById(R.id.textView2);
text.setText(fragment1Spinner);
return view;
}
}
This question is an exact duplicate of:
Passing a Spinner value from one fragment to another
1 answer
java android
java android
asked Nov 23 '18 at 14:53
user9488593
marked as duplicate by Mike M.
StackExchange.ready(function() {
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function() {
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function() {
$hover.showInfoMessage('', {
messageElement: $msg.clone().show(),
transient: false,
position: { my: 'bottom left', at: 'top center', offsetTop: -7 },
dismissable: false,
relativeToBody: true
});
},
function() {
StackExchange.helpers.removeMessages();
}
);
});
});
Nov 23 '18 at 14:58
This question was marked as an exact duplicate of an existing question.
marked as duplicate by Mike M.
StackExchange.ready(function() {
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function() {
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function() {
$hover.showInfoMessage('', {
messageElement: $msg.clone().show(),
transient: false,
position: { my: 'bottom left', at: 'top center', offsetTop: -7 },
dismissable: false,
relativeToBody: true
});
},
function() {
StackExchange.helpers.removeMessages();
}
);
});
});
Nov 23 '18 at 14:58
This question was marked as an exact duplicate of an existing question.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I'd recommend looking into the Data Binding Library to easily access data from all Fragments (or anything really) using the ViewModel software principle.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I'd recommend looking into the Data Binding Library to easily access data from all Fragments (or anything really) using the ViewModel software principle.
add a comment |
I'd recommend looking into the Data Binding Library to easily access data from all Fragments (or anything really) using the ViewModel software principle.
add a comment |
I'd recommend looking into the Data Binding Library to easily access data from all Fragments (or anything really) using the ViewModel software principle.
I'd recommend looking into the Data Binding Library to easily access data from all Fragments (or anything really) using the ViewModel software principle.
answered Nov 23 '18 at 14:58


SnakeyHips
540111
540111
add a comment |
add a comment |
tnz j fdFKu8WPOtUEpM4R bEhcpu