What should the generator return if it is used in a multi-model functional API?
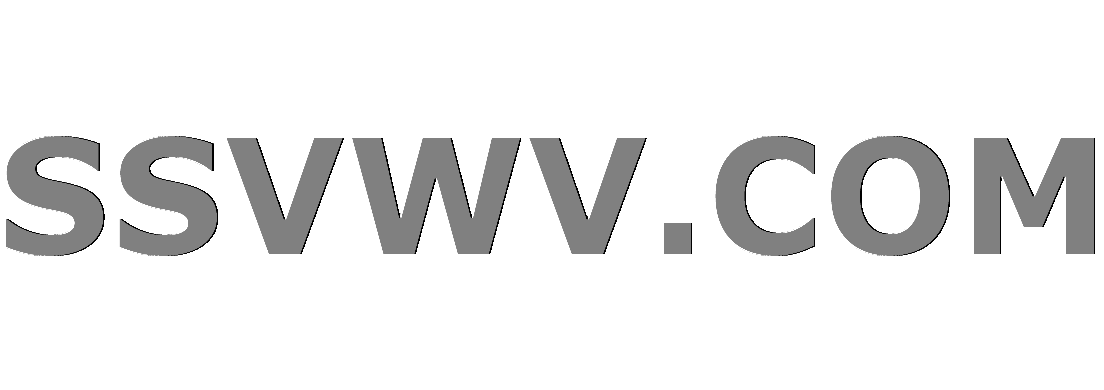
Multi tool use
Following this article, I'm trying to implement a generative RNN. In the mentioned article, the training and validation data are passed as fully loaded np.array
s. But I'm trying to use the model.fit_generator
method and provide a generator instead.
I know that if it was a straightforward model, the generator should return:
def generator():
...
yield (samples, targets)
But this is a generative model which means there are two models involved:
encoder_inputs = Input(shape=(None,))
x = Embedding(num_encoder_tokens, embedding_dim)(encoder_inputs)
x.set_weights([embedding_matrix])
x.trainable = False
x, state_h, state_c = LSTM(embedding_dim, return_state=True)(x)
encoder_states = [state_h, state_c]
decoder_inputs = Input(shape=(None,))
x = Embedding(num_decoder_tokens, embedding_dim)(decoder_inputs)
x.set_weights([embedding_matrix])
x.trainable = False
x = LSTM(embedding_dim, return_sequences=True)(x, initial_state=encoder_states)
decoder_outputs = Dense(num_decoder_tokens, activation='softmax')(x)
model = Model([encoder_inputs, decoder_inputs], decoder_outputs)
model.fit([encoder_input_data, decoder_input_data], decoder_target_data,
batch_size=batch_size,
epochs=epochs,
validation_split=0.2)
As mentioned before, I'm trying to use a generator:
model.fit_generator(generator(),
steps_per_epoch=500,
epochs=20,
validation_data=generator(),
validation_steps=val_steps)
But what should the generator()
return? I'm a little confused since there are two input collections and one target.
python machine-learning keras generator rnn
add a comment |
Following this article, I'm trying to implement a generative RNN. In the mentioned article, the training and validation data are passed as fully loaded np.array
s. But I'm trying to use the model.fit_generator
method and provide a generator instead.
I know that if it was a straightforward model, the generator should return:
def generator():
...
yield (samples, targets)
But this is a generative model which means there are two models involved:
encoder_inputs = Input(shape=(None,))
x = Embedding(num_encoder_tokens, embedding_dim)(encoder_inputs)
x.set_weights([embedding_matrix])
x.trainable = False
x, state_h, state_c = LSTM(embedding_dim, return_state=True)(x)
encoder_states = [state_h, state_c]
decoder_inputs = Input(shape=(None,))
x = Embedding(num_decoder_tokens, embedding_dim)(decoder_inputs)
x.set_weights([embedding_matrix])
x.trainable = False
x = LSTM(embedding_dim, return_sequences=True)(x, initial_state=encoder_states)
decoder_outputs = Dense(num_decoder_tokens, activation='softmax')(x)
model = Model([encoder_inputs, decoder_inputs], decoder_outputs)
model.fit([encoder_input_data, decoder_input_data], decoder_target_data,
batch_size=batch_size,
epochs=epochs,
validation_split=0.2)
As mentioned before, I'm trying to use a generator:
model.fit_generator(generator(),
steps_per_epoch=500,
epochs=20,
validation_data=generator(),
validation_steps=val_steps)
But what should the generator()
return? I'm a little confused since there are two input collections and one target.
python machine-learning keras generator rnn
add a comment |
Following this article, I'm trying to implement a generative RNN. In the mentioned article, the training and validation data are passed as fully loaded np.array
s. But I'm trying to use the model.fit_generator
method and provide a generator instead.
I know that if it was a straightforward model, the generator should return:
def generator():
...
yield (samples, targets)
But this is a generative model which means there are two models involved:
encoder_inputs = Input(shape=(None,))
x = Embedding(num_encoder_tokens, embedding_dim)(encoder_inputs)
x.set_weights([embedding_matrix])
x.trainable = False
x, state_h, state_c = LSTM(embedding_dim, return_state=True)(x)
encoder_states = [state_h, state_c]
decoder_inputs = Input(shape=(None,))
x = Embedding(num_decoder_tokens, embedding_dim)(decoder_inputs)
x.set_weights([embedding_matrix])
x.trainable = False
x = LSTM(embedding_dim, return_sequences=True)(x, initial_state=encoder_states)
decoder_outputs = Dense(num_decoder_tokens, activation='softmax')(x)
model = Model([encoder_inputs, decoder_inputs], decoder_outputs)
model.fit([encoder_input_data, decoder_input_data], decoder_target_data,
batch_size=batch_size,
epochs=epochs,
validation_split=0.2)
As mentioned before, I'm trying to use a generator:
model.fit_generator(generator(),
steps_per_epoch=500,
epochs=20,
validation_data=generator(),
validation_steps=val_steps)
But what should the generator()
return? I'm a little confused since there are two input collections and one target.
python machine-learning keras generator rnn
Following this article, I'm trying to implement a generative RNN. In the mentioned article, the training and validation data are passed as fully loaded np.array
s. But I'm trying to use the model.fit_generator
method and provide a generator instead.
I know that if it was a straightforward model, the generator should return:
def generator():
...
yield (samples, targets)
But this is a generative model which means there are two models involved:
encoder_inputs = Input(shape=(None,))
x = Embedding(num_encoder_tokens, embedding_dim)(encoder_inputs)
x.set_weights([embedding_matrix])
x.trainable = False
x, state_h, state_c = LSTM(embedding_dim, return_state=True)(x)
encoder_states = [state_h, state_c]
decoder_inputs = Input(shape=(None,))
x = Embedding(num_decoder_tokens, embedding_dim)(decoder_inputs)
x.set_weights([embedding_matrix])
x.trainable = False
x = LSTM(embedding_dim, return_sequences=True)(x, initial_state=encoder_states)
decoder_outputs = Dense(num_decoder_tokens, activation='softmax')(x)
model = Model([encoder_inputs, decoder_inputs], decoder_outputs)
model.fit([encoder_input_data, decoder_input_data], decoder_target_data,
batch_size=batch_size,
epochs=epochs,
validation_split=0.2)
As mentioned before, I'm trying to use a generator:
model.fit_generator(generator(),
steps_per_epoch=500,
epochs=20,
validation_data=generator(),
validation_steps=val_steps)
But what should the generator()
return? I'm a little confused since there are two input collections and one target.
python machine-learning keras generator rnn
python machine-learning keras generator rnn
edited Nov 26 '18 at 9:32
today
10.8k21837
10.8k21837
asked Nov 26 '18 at 3:39


MehranMehran
3,925746112
3,925746112
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Since your model has two inputs and one output, the generator should return a tuple with two elements where the first element is a list containing two arrays, which corresponds to two input layers, and the second element is an array corresponding to output layer:
def generator():
...
yield [input_samples1, input_samples2], targets
Generally, in a model with M
inputs and N
outputs, the generator should return a tuple of two lists where the first one has M
arrays and the second one has N
arrays:
def generator():
...
yield [in1, in2, ..., inM], [out1, out2, ..., outN]
Thanks. I'll accept this once I get to verify it. Right now, my computer is busy with other stuff.
– Mehran
Nov 26 '18 at 14:12
Thanks for the answer, it worked. I just learned that you can also use the "named" layers and a dictionary. It would be great to add that to your answer to make it complete.
– Mehran
Nov 27 '18 at 14:03
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474497%2fwhat-should-the-generator-return-if-it-is-used-in-a-multi-model-functional-api%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Since your model has two inputs and one output, the generator should return a tuple with two elements where the first element is a list containing two arrays, which corresponds to two input layers, and the second element is an array corresponding to output layer:
def generator():
...
yield [input_samples1, input_samples2], targets
Generally, in a model with M
inputs and N
outputs, the generator should return a tuple of two lists where the first one has M
arrays and the second one has N
arrays:
def generator():
...
yield [in1, in2, ..., inM], [out1, out2, ..., outN]
Thanks. I'll accept this once I get to verify it. Right now, my computer is busy with other stuff.
– Mehran
Nov 26 '18 at 14:12
Thanks for the answer, it worked. I just learned that you can also use the "named" layers and a dictionary. It would be great to add that to your answer to make it complete.
– Mehran
Nov 27 '18 at 14:03
add a comment |
Since your model has two inputs and one output, the generator should return a tuple with two elements where the first element is a list containing two arrays, which corresponds to two input layers, and the second element is an array corresponding to output layer:
def generator():
...
yield [input_samples1, input_samples2], targets
Generally, in a model with M
inputs and N
outputs, the generator should return a tuple of two lists where the first one has M
arrays and the second one has N
arrays:
def generator():
...
yield [in1, in2, ..., inM], [out1, out2, ..., outN]
Thanks. I'll accept this once I get to verify it. Right now, my computer is busy with other stuff.
– Mehran
Nov 26 '18 at 14:12
Thanks for the answer, it worked. I just learned that you can also use the "named" layers and a dictionary. It would be great to add that to your answer to make it complete.
– Mehran
Nov 27 '18 at 14:03
add a comment |
Since your model has two inputs and one output, the generator should return a tuple with two elements where the first element is a list containing two arrays, which corresponds to two input layers, and the second element is an array corresponding to output layer:
def generator():
...
yield [input_samples1, input_samples2], targets
Generally, in a model with M
inputs and N
outputs, the generator should return a tuple of two lists where the first one has M
arrays and the second one has N
arrays:
def generator():
...
yield [in1, in2, ..., inM], [out1, out2, ..., outN]
Since your model has two inputs and one output, the generator should return a tuple with two elements where the first element is a list containing two arrays, which corresponds to two input layers, and the second element is an array corresponding to output layer:
def generator():
...
yield [input_samples1, input_samples2], targets
Generally, in a model with M
inputs and N
outputs, the generator should return a tuple of two lists where the first one has M
arrays and the second one has N
arrays:
def generator():
...
yield [in1, in2, ..., inM], [out1, out2, ..., outN]
edited Nov 27 '18 at 10:43
answered Nov 26 '18 at 9:30
todaytoday
10.8k21837
10.8k21837
Thanks. I'll accept this once I get to verify it. Right now, my computer is busy with other stuff.
– Mehran
Nov 26 '18 at 14:12
Thanks for the answer, it worked. I just learned that you can also use the "named" layers and a dictionary. It would be great to add that to your answer to make it complete.
– Mehran
Nov 27 '18 at 14:03
add a comment |
Thanks. I'll accept this once I get to verify it. Right now, my computer is busy with other stuff.
– Mehran
Nov 26 '18 at 14:12
Thanks for the answer, it worked. I just learned that you can also use the "named" layers and a dictionary. It would be great to add that to your answer to make it complete.
– Mehran
Nov 27 '18 at 14:03
Thanks. I'll accept this once I get to verify it. Right now, my computer is busy with other stuff.
– Mehran
Nov 26 '18 at 14:12
Thanks. I'll accept this once I get to verify it. Right now, my computer is busy with other stuff.
– Mehran
Nov 26 '18 at 14:12
Thanks for the answer, it worked. I just learned that you can also use the "named" layers and a dictionary. It would be great to add that to your answer to make it complete.
– Mehran
Nov 27 '18 at 14:03
Thanks for the answer, it worked. I just learned that you can also use the "named" layers and a dictionary. It would be great to add that to your answer to make it complete.
– Mehran
Nov 27 '18 at 14:03
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474497%2fwhat-should-the-generator-return-if-it-is-used-in-a-multi-model-functional-api%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
rn U,h75Zn84HpKDjCm