Is there a way to pass an rvalue by reference in C++?
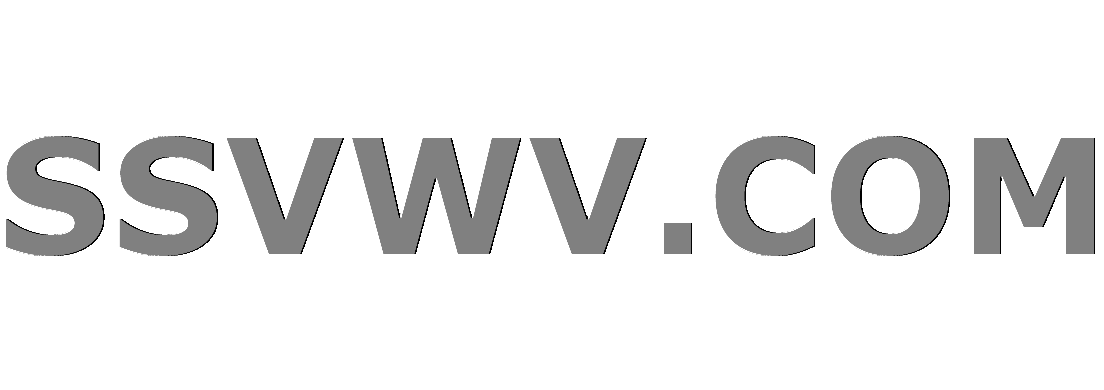
Multi tool use
I'm trying to create "rollback" functionality for a small database assignment.
I have a stack of binary search trees that I am using to store backups of the database called:
GenStack<GenBST<Student>> masterStudentStack;
Both the stack and BST are my own implementations (per the instructions for my assignment).
I have no problem pushing a copy of the BST onto the stack,
masterStudentStack.push(*masterStudent);
however when I try to retrieve this BST and return it to my primary BST pointer
using:
void rollBack() {
masterStudent = new GenBST<Student>(masterStudentStack.pop());
}
I receive an error.
Menu.cpp:419:63: error: invalid initialization of non-const reference of
type ‘GenBST<Student>&’ from an rvalue of type ‘GenBST<Student>’
masterStudent = new GenBST<Student>(masterStudentStack.pop());
~~~~~~~~~~~~~~~~~~~~~~^~
In file included from Menu.h:7:0,
from Menu.cpp:1:
GenBST.h:49:1: note: initializing argument 1 of
‘GenBST<T>::GenBST(GenBST<T>&) [with T = Student]’
GenBST<T>::GenBST(GenBST<T>& other) {
^~~~~~~~~
The copy constructor for the BST is functional when an lvalue is passed in by reference, (this is the declaration for the constructor)
GenBST(GenBST<T>& other);
but I don't know how to pop something off the stack in a way in which the copy constructor will accept it. So, my question is: Can I something create a new BST using the rvalue "stack.pop()"?
Thanks,
Matthew
Edit:
After adding "const" to my BST copy constructor, I got this error
In file included from Menu.h:7:0,
from Menu.cpp:1:
GenBST.h: In instantiation of ‘GenBST<T>::GenBST(const GenBST<T>&) [with T =
Student]’:
Menu.cpp:419:65: required from here
GenBST.h:50:22: error: passing ‘const GenBST<Student>’ as ‘this’ argument
discards qualifiers [-fpermissive]
if(other.getRoot() == NULL) {
GenBST.h:77:17: note: in call to ‘GenTreeNode<T>* GenBST<T>::getRoot()
[with T = Student]’
GenTreeNode<T>* GenBST<T>::getRoot()
^~~~~~~~~
GenBST.h:54:32: error: binding ‘GenTreeNode<Student>* const’ to reference of
type ‘GenTreeNode<Student>*&’ discards qualifiers
copyTree(this->root, other.root);
~~~~~~^~~~
GenBST.h:108:6: note: initializing argument 2 of ‘void
GenBST<T>::copyTree(GenTreeNode<T>*&, GenTreeNode<T>*&) [with T = Student]’
void GenBST<T>::copyTree(GenTreeNode<T> *& thisNode, GenTreeNode<T> *&
otherNode) {
Here is my constructor and the method it calls:
template <class T>
GenBST<T>::GenBST(const GenBST<T>& other) {
if(other.getRoot() == NULL) {
root = NULL;
}
else {
copyTree(this->root, other.root);
}
}
template <class T>
void GenBST<T>::copyTree(GenTreeNode<T> *& thisNode, GenTreeNode<T> *&
otherNode) {
if(otherNode == NULL) {
thisNode = NULL;
}
else {
thisNode = new GenTreeNode<T>(otherNode->key);
copyTree(thisNode->left, otherNode->left);
copyTree(thisNode->right, otherNode->right);
}
}
Any ideas?
Edit 2:
Thank so much for the help everybody. I added const to both my getRoot() and copyTree() methods and am now down to one error.
GenBST.h: In instantiation of ‘GenBST<T>::GenBST(const GenBST<T>&) [with T =
Student]’:
Menu.cpp:419:65: required from here
GenBST.h:54:32: error: binding ‘GenTreeNode<Student>* const’ to reference of
type ‘GenTreeNode<Student>*&’ discards qualifiers
copyTree(this->root, other.root);
~~~~~~^~~~
GenBST.h:108:6: note: initializing argument 2 of ‘void
GenBST<T>::copyTree(GenTreeNode<T>*&, GenTreeNode<T>*&) const [with T =
Student]’
void GenBST<T>::copyTree(GenTreeNode<T> *& thisNode, GenTreeNode<T> *&
otherNode) const {
c++ stack binary-search-tree rvalue lvalue
add a comment |
I'm trying to create "rollback" functionality for a small database assignment.
I have a stack of binary search trees that I am using to store backups of the database called:
GenStack<GenBST<Student>> masterStudentStack;
Both the stack and BST are my own implementations (per the instructions for my assignment).
I have no problem pushing a copy of the BST onto the stack,
masterStudentStack.push(*masterStudent);
however when I try to retrieve this BST and return it to my primary BST pointer
using:
void rollBack() {
masterStudent = new GenBST<Student>(masterStudentStack.pop());
}
I receive an error.
Menu.cpp:419:63: error: invalid initialization of non-const reference of
type ‘GenBST<Student>&’ from an rvalue of type ‘GenBST<Student>’
masterStudent = new GenBST<Student>(masterStudentStack.pop());
~~~~~~~~~~~~~~~~~~~~~~^~
In file included from Menu.h:7:0,
from Menu.cpp:1:
GenBST.h:49:1: note: initializing argument 1 of
‘GenBST<T>::GenBST(GenBST<T>&) [with T = Student]’
GenBST<T>::GenBST(GenBST<T>& other) {
^~~~~~~~~
The copy constructor for the BST is functional when an lvalue is passed in by reference, (this is the declaration for the constructor)
GenBST(GenBST<T>& other);
but I don't know how to pop something off the stack in a way in which the copy constructor will accept it. So, my question is: Can I something create a new BST using the rvalue "stack.pop()"?
Thanks,
Matthew
Edit:
After adding "const" to my BST copy constructor, I got this error
In file included from Menu.h:7:0,
from Menu.cpp:1:
GenBST.h: In instantiation of ‘GenBST<T>::GenBST(const GenBST<T>&) [with T =
Student]’:
Menu.cpp:419:65: required from here
GenBST.h:50:22: error: passing ‘const GenBST<Student>’ as ‘this’ argument
discards qualifiers [-fpermissive]
if(other.getRoot() == NULL) {
GenBST.h:77:17: note: in call to ‘GenTreeNode<T>* GenBST<T>::getRoot()
[with T = Student]’
GenTreeNode<T>* GenBST<T>::getRoot()
^~~~~~~~~
GenBST.h:54:32: error: binding ‘GenTreeNode<Student>* const’ to reference of
type ‘GenTreeNode<Student>*&’ discards qualifiers
copyTree(this->root, other.root);
~~~~~~^~~~
GenBST.h:108:6: note: initializing argument 2 of ‘void
GenBST<T>::copyTree(GenTreeNode<T>*&, GenTreeNode<T>*&) [with T = Student]’
void GenBST<T>::copyTree(GenTreeNode<T> *& thisNode, GenTreeNode<T> *&
otherNode) {
Here is my constructor and the method it calls:
template <class T>
GenBST<T>::GenBST(const GenBST<T>& other) {
if(other.getRoot() == NULL) {
root = NULL;
}
else {
copyTree(this->root, other.root);
}
}
template <class T>
void GenBST<T>::copyTree(GenTreeNode<T> *& thisNode, GenTreeNode<T> *&
otherNode) {
if(otherNode == NULL) {
thisNode = NULL;
}
else {
thisNode = new GenTreeNode<T>(otherNode->key);
copyTree(thisNode->left, otherNode->left);
copyTree(thisNode->right, otherNode->right);
}
}
Any ideas?
Edit 2:
Thank so much for the help everybody. I added const to both my getRoot() and copyTree() methods and am now down to one error.
GenBST.h: In instantiation of ‘GenBST<T>::GenBST(const GenBST<T>&) [with T =
Student]’:
Menu.cpp:419:65: required from here
GenBST.h:54:32: error: binding ‘GenTreeNode<Student>* const’ to reference of
type ‘GenTreeNode<Student>*&’ discards qualifiers
copyTree(this->root, other.root);
~~~~~~^~~~
GenBST.h:108:6: note: initializing argument 2 of ‘void
GenBST<T>::copyTree(GenTreeNode<T>*&, GenTreeNode<T>*&) const [with T =
Student]’
void GenBST<T>::copyTree(GenTreeNode<T> *& thisNode, GenTreeNode<T> *&
otherNode) const {
c++ stack binary-search-tree rvalue lvalue
you also need to makegetRoot()
andcopyTree
be const methods
– M.M
Nov 26 '18 at 3:39
Also you probably shouldn't be usingnew
anddelete
withmasterStudent
(it could be aGenBST
instead of a pointer to one , since you seem to be using value semantics with it anyway)
– M.M
Nov 26 '18 at 3:40
add a comment |
I'm trying to create "rollback" functionality for a small database assignment.
I have a stack of binary search trees that I am using to store backups of the database called:
GenStack<GenBST<Student>> masterStudentStack;
Both the stack and BST are my own implementations (per the instructions for my assignment).
I have no problem pushing a copy of the BST onto the stack,
masterStudentStack.push(*masterStudent);
however when I try to retrieve this BST and return it to my primary BST pointer
using:
void rollBack() {
masterStudent = new GenBST<Student>(masterStudentStack.pop());
}
I receive an error.
Menu.cpp:419:63: error: invalid initialization of non-const reference of
type ‘GenBST<Student>&’ from an rvalue of type ‘GenBST<Student>’
masterStudent = new GenBST<Student>(masterStudentStack.pop());
~~~~~~~~~~~~~~~~~~~~~~^~
In file included from Menu.h:7:0,
from Menu.cpp:1:
GenBST.h:49:1: note: initializing argument 1 of
‘GenBST<T>::GenBST(GenBST<T>&) [with T = Student]’
GenBST<T>::GenBST(GenBST<T>& other) {
^~~~~~~~~
The copy constructor for the BST is functional when an lvalue is passed in by reference, (this is the declaration for the constructor)
GenBST(GenBST<T>& other);
but I don't know how to pop something off the stack in a way in which the copy constructor will accept it. So, my question is: Can I something create a new BST using the rvalue "stack.pop()"?
Thanks,
Matthew
Edit:
After adding "const" to my BST copy constructor, I got this error
In file included from Menu.h:7:0,
from Menu.cpp:1:
GenBST.h: In instantiation of ‘GenBST<T>::GenBST(const GenBST<T>&) [with T =
Student]’:
Menu.cpp:419:65: required from here
GenBST.h:50:22: error: passing ‘const GenBST<Student>’ as ‘this’ argument
discards qualifiers [-fpermissive]
if(other.getRoot() == NULL) {
GenBST.h:77:17: note: in call to ‘GenTreeNode<T>* GenBST<T>::getRoot()
[with T = Student]’
GenTreeNode<T>* GenBST<T>::getRoot()
^~~~~~~~~
GenBST.h:54:32: error: binding ‘GenTreeNode<Student>* const’ to reference of
type ‘GenTreeNode<Student>*&’ discards qualifiers
copyTree(this->root, other.root);
~~~~~~^~~~
GenBST.h:108:6: note: initializing argument 2 of ‘void
GenBST<T>::copyTree(GenTreeNode<T>*&, GenTreeNode<T>*&) [with T = Student]’
void GenBST<T>::copyTree(GenTreeNode<T> *& thisNode, GenTreeNode<T> *&
otherNode) {
Here is my constructor and the method it calls:
template <class T>
GenBST<T>::GenBST(const GenBST<T>& other) {
if(other.getRoot() == NULL) {
root = NULL;
}
else {
copyTree(this->root, other.root);
}
}
template <class T>
void GenBST<T>::copyTree(GenTreeNode<T> *& thisNode, GenTreeNode<T> *&
otherNode) {
if(otherNode == NULL) {
thisNode = NULL;
}
else {
thisNode = new GenTreeNode<T>(otherNode->key);
copyTree(thisNode->left, otherNode->left);
copyTree(thisNode->right, otherNode->right);
}
}
Any ideas?
Edit 2:
Thank so much for the help everybody. I added const to both my getRoot() and copyTree() methods and am now down to one error.
GenBST.h: In instantiation of ‘GenBST<T>::GenBST(const GenBST<T>&) [with T =
Student]’:
Menu.cpp:419:65: required from here
GenBST.h:54:32: error: binding ‘GenTreeNode<Student>* const’ to reference of
type ‘GenTreeNode<Student>*&’ discards qualifiers
copyTree(this->root, other.root);
~~~~~~^~~~
GenBST.h:108:6: note: initializing argument 2 of ‘void
GenBST<T>::copyTree(GenTreeNode<T>*&, GenTreeNode<T>*&) const [with T =
Student]’
void GenBST<T>::copyTree(GenTreeNode<T> *& thisNode, GenTreeNode<T> *&
otherNode) const {
c++ stack binary-search-tree rvalue lvalue
I'm trying to create "rollback" functionality for a small database assignment.
I have a stack of binary search trees that I am using to store backups of the database called:
GenStack<GenBST<Student>> masterStudentStack;
Both the stack and BST are my own implementations (per the instructions for my assignment).
I have no problem pushing a copy of the BST onto the stack,
masterStudentStack.push(*masterStudent);
however when I try to retrieve this BST and return it to my primary BST pointer
using:
void rollBack() {
masterStudent = new GenBST<Student>(masterStudentStack.pop());
}
I receive an error.
Menu.cpp:419:63: error: invalid initialization of non-const reference of
type ‘GenBST<Student>&’ from an rvalue of type ‘GenBST<Student>’
masterStudent = new GenBST<Student>(masterStudentStack.pop());
~~~~~~~~~~~~~~~~~~~~~~^~
In file included from Menu.h:7:0,
from Menu.cpp:1:
GenBST.h:49:1: note: initializing argument 1 of
‘GenBST<T>::GenBST(GenBST<T>&) [with T = Student]’
GenBST<T>::GenBST(GenBST<T>& other) {
^~~~~~~~~
The copy constructor for the BST is functional when an lvalue is passed in by reference, (this is the declaration for the constructor)
GenBST(GenBST<T>& other);
but I don't know how to pop something off the stack in a way in which the copy constructor will accept it. So, my question is: Can I something create a new BST using the rvalue "stack.pop()"?
Thanks,
Matthew
Edit:
After adding "const" to my BST copy constructor, I got this error
In file included from Menu.h:7:0,
from Menu.cpp:1:
GenBST.h: In instantiation of ‘GenBST<T>::GenBST(const GenBST<T>&) [with T =
Student]’:
Menu.cpp:419:65: required from here
GenBST.h:50:22: error: passing ‘const GenBST<Student>’ as ‘this’ argument
discards qualifiers [-fpermissive]
if(other.getRoot() == NULL) {
GenBST.h:77:17: note: in call to ‘GenTreeNode<T>* GenBST<T>::getRoot()
[with T = Student]’
GenTreeNode<T>* GenBST<T>::getRoot()
^~~~~~~~~
GenBST.h:54:32: error: binding ‘GenTreeNode<Student>* const’ to reference of
type ‘GenTreeNode<Student>*&’ discards qualifiers
copyTree(this->root, other.root);
~~~~~~^~~~
GenBST.h:108:6: note: initializing argument 2 of ‘void
GenBST<T>::copyTree(GenTreeNode<T>*&, GenTreeNode<T>*&) [with T = Student]’
void GenBST<T>::copyTree(GenTreeNode<T> *& thisNode, GenTreeNode<T> *&
otherNode) {
Here is my constructor and the method it calls:
template <class T>
GenBST<T>::GenBST(const GenBST<T>& other) {
if(other.getRoot() == NULL) {
root = NULL;
}
else {
copyTree(this->root, other.root);
}
}
template <class T>
void GenBST<T>::copyTree(GenTreeNode<T> *& thisNode, GenTreeNode<T> *&
otherNode) {
if(otherNode == NULL) {
thisNode = NULL;
}
else {
thisNode = new GenTreeNode<T>(otherNode->key);
copyTree(thisNode->left, otherNode->left);
copyTree(thisNode->right, otherNode->right);
}
}
Any ideas?
Edit 2:
Thank so much for the help everybody. I added const to both my getRoot() and copyTree() methods and am now down to one error.
GenBST.h: In instantiation of ‘GenBST<T>::GenBST(const GenBST<T>&) [with T =
Student]’:
Menu.cpp:419:65: required from here
GenBST.h:54:32: error: binding ‘GenTreeNode<Student>* const’ to reference of
type ‘GenTreeNode<Student>*&’ discards qualifiers
copyTree(this->root, other.root);
~~~~~~^~~~
GenBST.h:108:6: note: initializing argument 2 of ‘void
GenBST<T>::copyTree(GenTreeNode<T>*&, GenTreeNode<T>*&) const [with T =
Student]’
void GenBST<T>::copyTree(GenTreeNode<T> *& thisNode, GenTreeNode<T> *&
otherNode) const {
c++ stack binary-search-tree rvalue lvalue
c++ stack binary-search-tree rvalue lvalue
edited Nov 26 '18 at 3:44
Matthew Parnham
asked Nov 26 '18 at 3:20
Matthew ParnhamMatthew Parnham
224
224
you also need to makegetRoot()
andcopyTree
be const methods
– M.M
Nov 26 '18 at 3:39
Also you probably shouldn't be usingnew
anddelete
withmasterStudent
(it could be aGenBST
instead of a pointer to one , since you seem to be using value semantics with it anyway)
– M.M
Nov 26 '18 at 3:40
add a comment |
you also need to makegetRoot()
andcopyTree
be const methods
– M.M
Nov 26 '18 at 3:39
Also you probably shouldn't be usingnew
anddelete
withmasterStudent
(it could be aGenBST
instead of a pointer to one , since you seem to be using value semantics with it anyway)
– M.M
Nov 26 '18 at 3:40
you also need to make
getRoot()
and copyTree
be const methods– M.M
Nov 26 '18 at 3:39
you also need to make
getRoot()
and copyTree
be const methods– M.M
Nov 26 '18 at 3:39
Also you probably shouldn't be using
new
and delete
with masterStudent
(it could be a GenBST
instead of a pointer to one , since you seem to be using value semantics with it anyway)– M.M
Nov 26 '18 at 3:40
Also you probably shouldn't be using
new
and delete
with masterStudent
(it could be a GenBST
instead of a pointer to one , since you seem to be using value semantics with it anyway)– M.M
Nov 26 '18 at 3:40
add a comment |
1 Answer
1
active
oldest
votes
The canonical form of a copy constructor takes a reference to a const object to copy. Conceptually, making a copy of something normally implies that the original object remains unchanged. There should hardly ever be a need to modify the object you're copying. An rvalue can be bound to a reference to const but not to a reference to non-const. Unless making a copy of a GenBST
really does actually entail modifying the object you're copying (I assume and sincerely hope it does not), you can simply change the signature of your copy constructor to
GenBST(const GenBST& other);
Thank you for the quick reply! I tried this, but it resulted in a different error that I don't quite understand. I'll attach it to my original post.
– Matthew Parnham
Nov 26 '18 at 3:32
Well, the problem is thatconst
is transitive. YourcopyTree()
method expects references to non-const objects but you give it references to objects that are nowconst
. You'll have to addconst
there too. With const-correctness it's all or nothing…
– Michael Kenzel
Nov 26 '18 at 3:40
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474381%2fis-there-a-way-to-pass-an-rvalue-by-reference-in-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The canonical form of a copy constructor takes a reference to a const object to copy. Conceptually, making a copy of something normally implies that the original object remains unchanged. There should hardly ever be a need to modify the object you're copying. An rvalue can be bound to a reference to const but not to a reference to non-const. Unless making a copy of a GenBST
really does actually entail modifying the object you're copying (I assume and sincerely hope it does not), you can simply change the signature of your copy constructor to
GenBST(const GenBST& other);
Thank you for the quick reply! I tried this, but it resulted in a different error that I don't quite understand. I'll attach it to my original post.
– Matthew Parnham
Nov 26 '18 at 3:32
Well, the problem is thatconst
is transitive. YourcopyTree()
method expects references to non-const objects but you give it references to objects that are nowconst
. You'll have to addconst
there too. With const-correctness it's all or nothing…
– Michael Kenzel
Nov 26 '18 at 3:40
add a comment |
The canonical form of a copy constructor takes a reference to a const object to copy. Conceptually, making a copy of something normally implies that the original object remains unchanged. There should hardly ever be a need to modify the object you're copying. An rvalue can be bound to a reference to const but not to a reference to non-const. Unless making a copy of a GenBST
really does actually entail modifying the object you're copying (I assume and sincerely hope it does not), you can simply change the signature of your copy constructor to
GenBST(const GenBST& other);
Thank you for the quick reply! I tried this, but it resulted in a different error that I don't quite understand. I'll attach it to my original post.
– Matthew Parnham
Nov 26 '18 at 3:32
Well, the problem is thatconst
is transitive. YourcopyTree()
method expects references to non-const objects but you give it references to objects that are nowconst
. You'll have to addconst
there too. With const-correctness it's all or nothing…
– Michael Kenzel
Nov 26 '18 at 3:40
add a comment |
The canonical form of a copy constructor takes a reference to a const object to copy. Conceptually, making a copy of something normally implies that the original object remains unchanged. There should hardly ever be a need to modify the object you're copying. An rvalue can be bound to a reference to const but not to a reference to non-const. Unless making a copy of a GenBST
really does actually entail modifying the object you're copying (I assume and sincerely hope it does not), you can simply change the signature of your copy constructor to
GenBST(const GenBST& other);
The canonical form of a copy constructor takes a reference to a const object to copy. Conceptually, making a copy of something normally implies that the original object remains unchanged. There should hardly ever be a need to modify the object you're copying. An rvalue can be bound to a reference to const but not to a reference to non-const. Unless making a copy of a GenBST
really does actually entail modifying the object you're copying (I assume and sincerely hope it does not), you can simply change the signature of your copy constructor to
GenBST(const GenBST& other);
edited Nov 26 '18 at 3:34
answered Nov 26 '18 at 3:27


Michael KenzelMichael Kenzel
4,2781819
4,2781819
Thank you for the quick reply! I tried this, but it resulted in a different error that I don't quite understand. I'll attach it to my original post.
– Matthew Parnham
Nov 26 '18 at 3:32
Well, the problem is thatconst
is transitive. YourcopyTree()
method expects references to non-const objects but you give it references to objects that are nowconst
. You'll have to addconst
there too. With const-correctness it's all or nothing…
– Michael Kenzel
Nov 26 '18 at 3:40
add a comment |
Thank you for the quick reply! I tried this, but it resulted in a different error that I don't quite understand. I'll attach it to my original post.
– Matthew Parnham
Nov 26 '18 at 3:32
Well, the problem is thatconst
is transitive. YourcopyTree()
method expects references to non-const objects but you give it references to objects that are nowconst
. You'll have to addconst
there too. With const-correctness it's all or nothing…
– Michael Kenzel
Nov 26 '18 at 3:40
Thank you for the quick reply! I tried this, but it resulted in a different error that I don't quite understand. I'll attach it to my original post.
– Matthew Parnham
Nov 26 '18 at 3:32
Thank you for the quick reply! I tried this, but it resulted in a different error that I don't quite understand. I'll attach it to my original post.
– Matthew Parnham
Nov 26 '18 at 3:32
Well, the problem is that
const
is transitive. Your copyTree()
method expects references to non-const objects but you give it references to objects that are now const
. You'll have to add const
there too. With const-correctness it's all or nothing…– Michael Kenzel
Nov 26 '18 at 3:40
Well, the problem is that
const
is transitive. Your copyTree()
method expects references to non-const objects but you give it references to objects that are now const
. You'll have to add const
there too. With const-correctness it's all or nothing…– Michael Kenzel
Nov 26 '18 at 3:40
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474381%2fis-there-a-way-to-pass-an-rvalue-by-reference-in-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8ZcM YQ9S6,E5CkHF yU b0L8wmQCFaXBGir9fTGkiYa5,xwdNh,mq J33a7766eQV3h3wSYLTF
you also need to make
getRoot()
andcopyTree
be const methods– M.M
Nov 26 '18 at 3:39
Also you probably shouldn't be using
new
anddelete
withmasterStudent
(it could be aGenBST
instead of a pointer to one , since you seem to be using value semantics with it anyway)– M.M
Nov 26 '18 at 3:40