How to print out the word with the most vowels
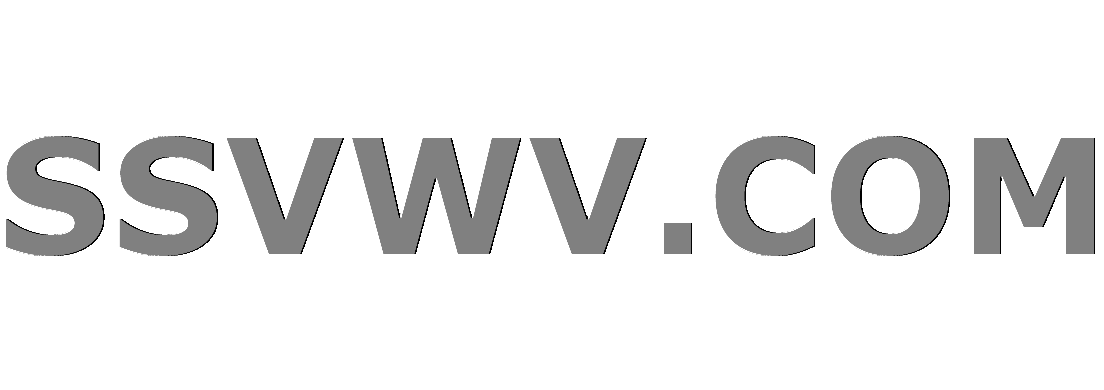
Multi tool use
I got a code which finds a word in a line that has at least 3 different vowels. The problem is I don't know why it goes out of bounds.
static string findword(string e)
{
char vowels = { 'a', 'e', 'i', 'o', 'u' };
char sk = { ' ', '.', ',', '!', '?', ':', ';', '(', ')', 't' };
string word= "";
string parts = e.Split(sk, StringSplitOptions.RemoveEmptyEntries);
for (int i = 0; i < e.Length; i++)
{
if (parts[i].IndexOf(vowels[i]) >= 3)
{
word = parts[i];
}
}
return word;
}
So for e = "I was going home today"
it should return "today"
.
c#
add a comment |
I got a code which finds a word in a line that has at least 3 different vowels. The problem is I don't know why it goes out of bounds.
static string findword(string e)
{
char vowels = { 'a', 'e', 'i', 'o', 'u' };
char sk = { ' ', '.', ',', '!', '?', ':', ';', '(', ')', 't' };
string word= "";
string parts = e.Split(sk, StringSplitOptions.RemoveEmptyEntries);
for (int i = 0; i < e.Length; i++)
{
if (parts[i].IndexOf(vowels[i]) >= 3)
{
word = parts[i];
}
}
return word;
}
So for e = "I was going home today"
it should return "today"
.
c#
Side-Note: if you just want the first word you should return it immediately from the loop
– Rango
Nov 26 '18 at 15:50
To check, is your question "Why do I get index out of bounds?", or "How do I return the word with the most vowels?"?
– JohnLBevan
Nov 26 '18 at 15:53
2
Besides the issues with iterating over the length of the string, according to thatvowels
array, none of the words have at least 3 vowels.
– Danny
Nov 26 '18 at 15:56
add a comment |
I got a code which finds a word in a line that has at least 3 different vowels. The problem is I don't know why it goes out of bounds.
static string findword(string e)
{
char vowels = { 'a', 'e', 'i', 'o', 'u' };
char sk = { ' ', '.', ',', '!', '?', ':', ';', '(', ')', 't' };
string word= "";
string parts = e.Split(sk, StringSplitOptions.RemoveEmptyEntries);
for (int i = 0; i < e.Length; i++)
{
if (parts[i].IndexOf(vowels[i]) >= 3)
{
word = parts[i];
}
}
return word;
}
So for e = "I was going home today"
it should return "today"
.
c#
I got a code which finds a word in a line that has at least 3 different vowels. The problem is I don't know why it goes out of bounds.
static string findword(string e)
{
char vowels = { 'a', 'e', 'i', 'o', 'u' };
char sk = { ' ', '.', ',', '!', '?', ':', ';', '(', ')', 't' };
string word= "";
string parts = e.Split(sk, StringSplitOptions.RemoveEmptyEntries);
for (int i = 0; i < e.Length; i++)
{
if (parts[i].IndexOf(vowels[i]) >= 3)
{
word = parts[i];
}
}
return word;
}
So for e = "I was going home today"
it should return "today"
.
c#
c#
edited Nov 26 '18 at 15:50
Micha Wiedenmann
10.4k1364104
10.4k1364104
asked Nov 26 '18 at 15:47
Scr1pt25Scr1pt25
304
304
Side-Note: if you just want the first word you should return it immediately from the loop
– Rango
Nov 26 '18 at 15:50
To check, is your question "Why do I get index out of bounds?", or "How do I return the word with the most vowels?"?
– JohnLBevan
Nov 26 '18 at 15:53
2
Besides the issues with iterating over the length of the string, according to thatvowels
array, none of the words have at least 3 vowels.
– Danny
Nov 26 '18 at 15:56
add a comment |
Side-Note: if you just want the first word you should return it immediately from the loop
– Rango
Nov 26 '18 at 15:50
To check, is your question "Why do I get index out of bounds?", or "How do I return the word with the most vowels?"?
– JohnLBevan
Nov 26 '18 at 15:53
2
Besides the issues with iterating over the length of the string, according to thatvowels
array, none of the words have at least 3 vowels.
– Danny
Nov 26 '18 at 15:56
Side-Note: if you just want the first word you should return it immediately from the loop
– Rango
Nov 26 '18 at 15:50
Side-Note: if you just want the first word you should return it immediately from the loop
– Rango
Nov 26 '18 at 15:50
To check, is your question "Why do I get index out of bounds?", or "How do I return the word with the most vowels?"?
– JohnLBevan
Nov 26 '18 at 15:53
To check, is your question "Why do I get index out of bounds?", or "How do I return the word with the most vowels?"?
– JohnLBevan
Nov 26 '18 at 15:53
2
2
Besides the issues with iterating over the length of the string, according to that
vowels
array, none of the words have at least 3 vowels.– Danny
Nov 26 '18 at 15:56
Besides the issues with iterating over the length of the string, according to that
vowels
array, none of the words have at least 3 vowels.– Danny
Nov 26 '18 at 15:56
add a comment |
3 Answers
3
active
oldest
votes
Here's my version:
var e = "I was going home today";
char vowels = { 'a', 'e', 'i', 'o', 'u' };
char sk = { ' ', '.', ',', '!', '?', ':', ';', '(', ')', 't' };
string word = "";
string parts = e.Split(sk, StringSplitOptions.RemoveEmptyEntries);
var mostVowels = 0;
for (int i = 0; i < parts.Length; i++)
{
var part = parts[i];
var numberOfVowels = 0;
foreach (var vowel in vowels)
{
if (part.Contains(vowel)) numberOfVowels++;
}
if (mostVowels < numberOfVowels)
{
mostVowels = i;
word = part;
}
}
return(word);
It loops through the parts, then another loop checks if the part contains each vowel. Then it checks whether this word is better than the previous best.
Note that this returns 'going' because it was the first word with two vowels.
Note also that I've used 'foreach' instead of a normal for loop, this helps to avoid many common mistakes.
This code could be better, as it only looks for each vowel once. A word like 'Aardvark' would only score 1. No doubt there's a really concise way to do this with LINQ and Regular Expressions, but hopefully this will help you see where you were going wrong with your loops and arrays.
EDIT: Here's a regex & LINQ version for you:
var r = new Regex("(a|e|i|o|u)");
var mostVowels = parts.Max(y => r.Matches(y).Count);
var result = parts.First(x => r.Matches(x).Count == mostVowels);
The Regex is a helper for finding vowels. The first query works out the number of vowels in each word and returns the maximum. The second finds the first word with that many vowels.
add a comment |
i
goes from 0 to e.Length - 1
, but you use it to access the array parts
, which might have different length or might not.
1
Alsovowels[i]
– Robin Bennett
Nov 26 '18 at 15:52
add a comment |
When the question is
why it goes out of bounds
(The question title suggest a totally different question problem than the context, it would be nice to fix the title.)
Then the answer is very simple. It is because of this:
Reason 1:
for (int i = 0; i < e.Length; i++)
Should be:
for (int i = 0; i < parths.Length; i++)
Reason 2:
if (parts[i].IndexOf(vowels[i]) >= 3)
Should be:
if (CountVowels(parts[i]) >= 3)
Unfortunately the CountVowels
function is out of scope for this question and since it sound like a homework you need to create the function CountVowels
by your self.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53484674%2fhow-to-print-out-the-word-with-the-most-vowels%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Here's my version:
var e = "I was going home today";
char vowels = { 'a', 'e', 'i', 'o', 'u' };
char sk = { ' ', '.', ',', '!', '?', ':', ';', '(', ')', 't' };
string word = "";
string parts = e.Split(sk, StringSplitOptions.RemoveEmptyEntries);
var mostVowels = 0;
for (int i = 0; i < parts.Length; i++)
{
var part = parts[i];
var numberOfVowels = 0;
foreach (var vowel in vowels)
{
if (part.Contains(vowel)) numberOfVowels++;
}
if (mostVowels < numberOfVowels)
{
mostVowels = i;
word = part;
}
}
return(word);
It loops through the parts, then another loop checks if the part contains each vowel. Then it checks whether this word is better than the previous best.
Note that this returns 'going' because it was the first word with two vowels.
Note also that I've used 'foreach' instead of a normal for loop, this helps to avoid many common mistakes.
This code could be better, as it only looks for each vowel once. A word like 'Aardvark' would only score 1. No doubt there's a really concise way to do this with LINQ and Regular Expressions, but hopefully this will help you see where you were going wrong with your loops and arrays.
EDIT: Here's a regex & LINQ version for you:
var r = new Regex("(a|e|i|o|u)");
var mostVowels = parts.Max(y => r.Matches(y).Count);
var result = parts.First(x => r.Matches(x).Count == mostVowels);
The Regex is a helper for finding vowels. The first query works out the number of vowels in each word and returns the maximum. The second finds the first word with that many vowels.
add a comment |
Here's my version:
var e = "I was going home today";
char vowels = { 'a', 'e', 'i', 'o', 'u' };
char sk = { ' ', '.', ',', '!', '?', ':', ';', '(', ')', 't' };
string word = "";
string parts = e.Split(sk, StringSplitOptions.RemoveEmptyEntries);
var mostVowels = 0;
for (int i = 0; i < parts.Length; i++)
{
var part = parts[i];
var numberOfVowels = 0;
foreach (var vowel in vowels)
{
if (part.Contains(vowel)) numberOfVowels++;
}
if (mostVowels < numberOfVowels)
{
mostVowels = i;
word = part;
}
}
return(word);
It loops through the parts, then another loop checks if the part contains each vowel. Then it checks whether this word is better than the previous best.
Note that this returns 'going' because it was the first word with two vowels.
Note also that I've used 'foreach' instead of a normal for loop, this helps to avoid many common mistakes.
This code could be better, as it only looks for each vowel once. A word like 'Aardvark' would only score 1. No doubt there's a really concise way to do this with LINQ and Regular Expressions, but hopefully this will help you see where you were going wrong with your loops and arrays.
EDIT: Here's a regex & LINQ version for you:
var r = new Regex("(a|e|i|o|u)");
var mostVowels = parts.Max(y => r.Matches(y).Count);
var result = parts.First(x => r.Matches(x).Count == mostVowels);
The Regex is a helper for finding vowels. The first query works out the number of vowels in each word and returns the maximum. The second finds the first word with that many vowels.
add a comment |
Here's my version:
var e = "I was going home today";
char vowels = { 'a', 'e', 'i', 'o', 'u' };
char sk = { ' ', '.', ',', '!', '?', ':', ';', '(', ')', 't' };
string word = "";
string parts = e.Split(sk, StringSplitOptions.RemoveEmptyEntries);
var mostVowels = 0;
for (int i = 0; i < parts.Length; i++)
{
var part = parts[i];
var numberOfVowels = 0;
foreach (var vowel in vowels)
{
if (part.Contains(vowel)) numberOfVowels++;
}
if (mostVowels < numberOfVowels)
{
mostVowels = i;
word = part;
}
}
return(word);
It loops through the parts, then another loop checks if the part contains each vowel. Then it checks whether this word is better than the previous best.
Note that this returns 'going' because it was the first word with two vowels.
Note also that I've used 'foreach' instead of a normal for loop, this helps to avoid many common mistakes.
This code could be better, as it only looks for each vowel once. A word like 'Aardvark' would only score 1. No doubt there's a really concise way to do this with LINQ and Regular Expressions, but hopefully this will help you see where you were going wrong with your loops and arrays.
EDIT: Here's a regex & LINQ version for you:
var r = new Regex("(a|e|i|o|u)");
var mostVowels = parts.Max(y => r.Matches(y).Count);
var result = parts.First(x => r.Matches(x).Count == mostVowels);
The Regex is a helper for finding vowels. The first query works out the number of vowels in each word and returns the maximum. The second finds the first word with that many vowels.
Here's my version:
var e = "I was going home today";
char vowels = { 'a', 'e', 'i', 'o', 'u' };
char sk = { ' ', '.', ',', '!', '?', ':', ';', '(', ')', 't' };
string word = "";
string parts = e.Split(sk, StringSplitOptions.RemoveEmptyEntries);
var mostVowels = 0;
for (int i = 0; i < parts.Length; i++)
{
var part = parts[i];
var numberOfVowels = 0;
foreach (var vowel in vowels)
{
if (part.Contains(vowel)) numberOfVowels++;
}
if (mostVowels < numberOfVowels)
{
mostVowels = i;
word = part;
}
}
return(word);
It loops through the parts, then another loop checks if the part contains each vowel. Then it checks whether this word is better than the previous best.
Note that this returns 'going' because it was the first word with two vowels.
Note also that I've used 'foreach' instead of a normal for loop, this helps to avoid many common mistakes.
This code could be better, as it only looks for each vowel once. A word like 'Aardvark' would only score 1. No doubt there's a really concise way to do this with LINQ and Regular Expressions, but hopefully this will help you see where you were going wrong with your loops and arrays.
EDIT: Here's a regex & LINQ version for you:
var r = new Regex("(a|e|i|o|u)");
var mostVowels = parts.Max(y => r.Matches(y).Count);
var result = parts.First(x => r.Matches(x).Count == mostVowels);
The Regex is a helper for finding vowels. The first query works out the number of vowels in each word and returns the maximum. The second finds the first word with that many vowels.
edited Nov 26 '18 at 16:28
answered Nov 26 '18 at 16:14


Robin BennettRobin Bennett
1,681312
1,681312
add a comment |
add a comment |
i
goes from 0 to e.Length - 1
, but you use it to access the array parts
, which might have different length or might not.
1
Alsovowels[i]
– Robin Bennett
Nov 26 '18 at 15:52
add a comment |
i
goes from 0 to e.Length - 1
, but you use it to access the array parts
, which might have different length or might not.
1
Alsovowels[i]
– Robin Bennett
Nov 26 '18 at 15:52
add a comment |
i
goes from 0 to e.Length - 1
, but you use it to access the array parts
, which might have different length or might not.
i
goes from 0 to e.Length - 1
, but you use it to access the array parts
, which might have different length or might not.
answered Nov 26 '18 at 15:50
Micha WiedenmannMicha Wiedenmann
10.4k1364104
10.4k1364104
1
Alsovowels[i]
– Robin Bennett
Nov 26 '18 at 15:52
add a comment |
1
Alsovowels[i]
– Robin Bennett
Nov 26 '18 at 15:52
1
1
Also
vowels[i]
– Robin Bennett
Nov 26 '18 at 15:52
Also
vowels[i]
– Robin Bennett
Nov 26 '18 at 15:52
add a comment |
When the question is
why it goes out of bounds
(The question title suggest a totally different question problem than the context, it would be nice to fix the title.)
Then the answer is very simple. It is because of this:
Reason 1:
for (int i = 0; i < e.Length; i++)
Should be:
for (int i = 0; i < parths.Length; i++)
Reason 2:
if (parts[i].IndexOf(vowels[i]) >= 3)
Should be:
if (CountVowels(parts[i]) >= 3)
Unfortunately the CountVowels
function is out of scope for this question and since it sound like a homework you need to create the function CountVowels
by your self.
add a comment |
When the question is
why it goes out of bounds
(The question title suggest a totally different question problem than the context, it would be nice to fix the title.)
Then the answer is very simple. It is because of this:
Reason 1:
for (int i = 0; i < e.Length; i++)
Should be:
for (int i = 0; i < parths.Length; i++)
Reason 2:
if (parts[i].IndexOf(vowels[i]) >= 3)
Should be:
if (CountVowels(parts[i]) >= 3)
Unfortunately the CountVowels
function is out of scope for this question and since it sound like a homework you need to create the function CountVowels
by your self.
add a comment |
When the question is
why it goes out of bounds
(The question title suggest a totally different question problem than the context, it would be nice to fix the title.)
Then the answer is very simple. It is because of this:
Reason 1:
for (int i = 0; i < e.Length; i++)
Should be:
for (int i = 0; i < parths.Length; i++)
Reason 2:
if (parts[i].IndexOf(vowels[i]) >= 3)
Should be:
if (CountVowels(parts[i]) >= 3)
Unfortunately the CountVowels
function is out of scope for this question and since it sound like a homework you need to create the function CountVowels
by your self.
When the question is
why it goes out of bounds
(The question title suggest a totally different question problem than the context, it would be nice to fix the title.)
Then the answer is very simple. It is because of this:
Reason 1:
for (int i = 0; i < e.Length; i++)
Should be:
for (int i = 0; i < parths.Length; i++)
Reason 2:
if (parts[i].IndexOf(vowels[i]) >= 3)
Should be:
if (CountVowels(parts[i]) >= 3)
Unfortunately the CountVowels
function is out of scope for this question and since it sound like a homework you need to create the function CountVowels
by your self.
answered Nov 26 '18 at 16:12
JuloJulo
8771815
8771815
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53484674%2fhow-to-print-out-the-word-with-the-most-vowels%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2RiBY8C9uV5bo7M1HoixSI4IiKvtf,ZxCDX,WWW9k ofnW xDxf2z jWB
Side-Note: if you just want the first word you should return it immediately from the loop
– Rango
Nov 26 '18 at 15:50
To check, is your question "Why do I get index out of bounds?", or "How do I return the word with the most vowels?"?
– JohnLBevan
Nov 26 '18 at 15:53
2
Besides the issues with iterating over the length of the string, according to that
vowels
array, none of the words have at least 3 vowels.– Danny
Nov 26 '18 at 15:56