Auto function returning value using switch
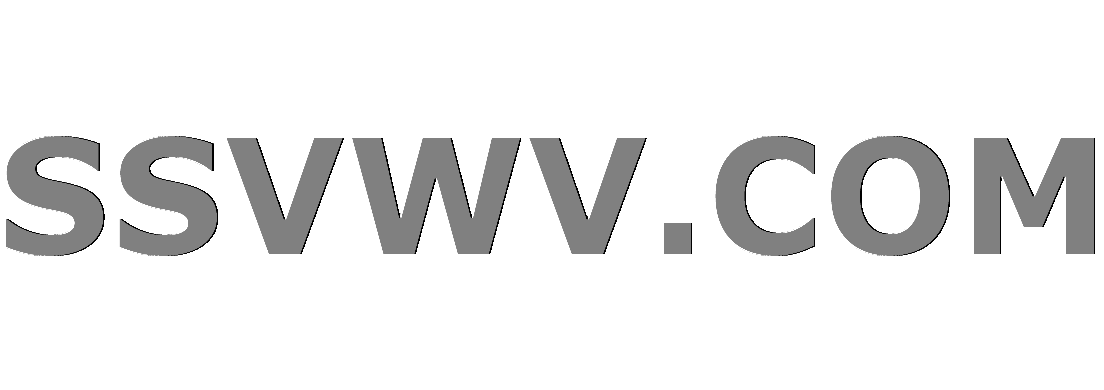
Multi tool use
I'm trying to create a function which return type should depend on switch statement, something like:
auto function_name (int value) {
switch (value) {
case 1 : {return 2.3;}
case 2 : {return 1;}
case 3 : {return "string";}
}
}
But I can't because of an error:
error: inconsistent deduction for auto return type: 'double' and then 'int'
What can I do to create something similar by functionality to the example above?
c++ return-type auto type-deduction
add a comment |
I'm trying to create a function which return type should depend on switch statement, something like:
auto function_name (int value) {
switch (value) {
case 1 : {return 2.3;}
case 2 : {return 1;}
case 3 : {return "string";}
}
}
But I can't because of an error:
error: inconsistent deduction for auto return type: 'double' and then 'int'
What can I do to create something similar by functionality to the example above?
c++ return-type auto type-deduction
3
You can't do this for multiple reasons.auto
has to be known at compile time.
– drescherjm
Nov 26 '18 at 15:48
1
C++
is a statically typed language.
– imreal
Nov 26 '18 at 15:48
4
You might be interested instd::variant
.
– HolyBlackCat
Nov 26 '18 at 15:50
4
What actual problem are you trying to solve with this? That is simply not how types work in C++. While you could use a std::variant, I'm hesitant to recommend it.
– paddy
Nov 26 '18 at 15:50
add a comment |
I'm trying to create a function which return type should depend on switch statement, something like:
auto function_name (int value) {
switch (value) {
case 1 : {return 2.3;}
case 2 : {return 1;}
case 3 : {return "string";}
}
}
But I can't because of an error:
error: inconsistent deduction for auto return type: 'double' and then 'int'
What can I do to create something similar by functionality to the example above?
c++ return-type auto type-deduction
I'm trying to create a function which return type should depend on switch statement, something like:
auto function_name (int value) {
switch (value) {
case 1 : {return 2.3;}
case 2 : {return 1;}
case 3 : {return "string";}
}
}
But I can't because of an error:
error: inconsistent deduction for auto return type: 'double' and then 'int'
What can I do to create something similar by functionality to the example above?
c++ return-type auto type-deduction
c++ return-type auto type-deduction
edited Nov 26 '18 at 16:48


NathanOliver
92.4k16129195
92.4k16129195
asked Nov 26 '18 at 15:47


NickNick
526
526
3
You can't do this for multiple reasons.auto
has to be known at compile time.
– drescherjm
Nov 26 '18 at 15:48
1
C++
is a statically typed language.
– imreal
Nov 26 '18 at 15:48
4
You might be interested instd::variant
.
– HolyBlackCat
Nov 26 '18 at 15:50
4
What actual problem are you trying to solve with this? That is simply not how types work in C++. While you could use a std::variant, I'm hesitant to recommend it.
– paddy
Nov 26 '18 at 15:50
add a comment |
3
You can't do this for multiple reasons.auto
has to be known at compile time.
– drescherjm
Nov 26 '18 at 15:48
1
C++
is a statically typed language.
– imreal
Nov 26 '18 at 15:48
4
You might be interested instd::variant
.
– HolyBlackCat
Nov 26 '18 at 15:50
4
What actual problem are you trying to solve with this? That is simply not how types work in C++. While you could use a std::variant, I'm hesitant to recommend it.
– paddy
Nov 26 '18 at 15:50
3
3
You can't do this for multiple reasons.
auto
has to be known at compile time.– drescherjm
Nov 26 '18 at 15:48
You can't do this for multiple reasons.
auto
has to be known at compile time.– drescherjm
Nov 26 '18 at 15:48
1
1
C++
is a statically typed language.– imreal
Nov 26 '18 at 15:48
C++
is a statically typed language.– imreal
Nov 26 '18 at 15:48
4
4
You might be interested in
std::variant
.– HolyBlackCat
Nov 26 '18 at 15:50
You might be interested in
std::variant
.– HolyBlackCat
Nov 26 '18 at 15:50
4
4
What actual problem are you trying to solve with this? That is simply not how types work in C++. While you could use a std::variant, I'm hesitant to recommend it.
– paddy
Nov 26 '18 at 15:50
What actual problem are you trying to solve with this? That is simply not how types work in C++. While you could use a std::variant, I'm hesitant to recommend it.
– paddy
Nov 26 '18 at 15:50
add a comment |
3 Answers
3
active
oldest
votes
A function in C++ can only have a single type that it returns. If you use auto
as the return type and you have different return statements that return different types then the code is ill-formed as it violates the single type rule.
This where std::variant
or std::any
needs to be used. If you have a few different types that could be returned via some run-time value then you could use either of those types as "generic type". std::variant
is more restrictive as you have to specify the types it could be, but it also less expensive then std::any
because you know what types it could be.
std::variant<double, int, std::string> function_name (int value) {
using namespace std::literals::string_literals;
switch (value) {
case 1 : {return 2.3;}
case 2 : {return 1;}
case 3 : {return "string"s;} // use ""s here to force it to be a std::string
}
}
Will let you return different types.
1
std::variant<auto>
would be pretty nice, though.
– Bartek Banachewicz
Nov 26 '18 at 15:57
3
@BartekBanachewicz And have it gather all the types the return statements use? That would be cool.
– NathanOliver
Nov 26 '18 at 15:57
1
would have to change"string"
by"string"s
though then.
– Jarod42
Nov 26 '18 at 15:59
1
IMO this answer should mention that this is possibly/likely the wrong way.variant
is the right tool in specific cases, but it can easily be an indicator of a design flaw. Yes, I know, you can't tell exactly from the question, but I find it worth mentioning. Otherwise the asker is potentially left trying to recover from halfway (or fully, withany
) leaving the type system which leads to more abuse.
– Max Langhof
Nov 26 '18 at 16:00
@Jarod42 That was bugging me too. I was editing when you commeted.
– NathanOliver
Nov 26 '18 at 16:01
|
show 4 more comments
If the function argument is known at compile time, you can use a compile time dispatch like e.g.
template <int N>
constexpr auto function_name()
{
if constexpr(N == 1)
return 2.3;
else if constexpr (N == 2)
return 1;
else
return "string";
}
which can be instantiated and invoked as follows
std::cout << function_name<1>() << "n";
C++17 is necessary for the if constexpr
part. Note that when binding the return value to a variable, carefully choose the type (e.g. to not implicitly convert a double
to an int
by accident), use type deduction or a variant
-type as shown in the existing answers.
Note that as @NathanOliver pointed out in the comments, there is also a pre-C++17 solution that uses template specialization instead of if constexpr
:
template <int N> constexpr auto function_name() { return "string"; }
template <> constexpr auto function_name<1>() { return 2.3; }
template <> constexpr auto function_name<2>() { return 1; }
The usage of this template and its specializations does not differ from the above.
add a comment |
The error message says it all: all branches of the function must return the same type. This limitation is not specific to auto
return type.
One possible fix:
std::variant<double, int, std::string> function_name(int value) {
switch(value) {
case 1 : return 2.3;
case 2 : return 1;
case 3 : return "string";
default: throw;
}
}
Alternatively, you can use boost::variant
.
There is a standard variant type now.
– François Andrieux
Nov 26 '18 at 16:18
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53484672%2fauto-function-returning-value-using-switch%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
A function in C++ can only have a single type that it returns. If you use auto
as the return type and you have different return statements that return different types then the code is ill-formed as it violates the single type rule.
This where std::variant
or std::any
needs to be used. If you have a few different types that could be returned via some run-time value then you could use either of those types as "generic type". std::variant
is more restrictive as you have to specify the types it could be, but it also less expensive then std::any
because you know what types it could be.
std::variant<double, int, std::string> function_name (int value) {
using namespace std::literals::string_literals;
switch (value) {
case 1 : {return 2.3;}
case 2 : {return 1;}
case 3 : {return "string"s;} // use ""s here to force it to be a std::string
}
}
Will let you return different types.
1
std::variant<auto>
would be pretty nice, though.
– Bartek Banachewicz
Nov 26 '18 at 15:57
3
@BartekBanachewicz And have it gather all the types the return statements use? That would be cool.
– NathanOliver
Nov 26 '18 at 15:57
1
would have to change"string"
by"string"s
though then.
– Jarod42
Nov 26 '18 at 15:59
1
IMO this answer should mention that this is possibly/likely the wrong way.variant
is the right tool in specific cases, but it can easily be an indicator of a design flaw. Yes, I know, you can't tell exactly from the question, but I find it worth mentioning. Otherwise the asker is potentially left trying to recover from halfway (or fully, withany
) leaving the type system which leads to more abuse.
– Max Langhof
Nov 26 '18 at 16:00
@Jarod42 That was bugging me too. I was editing when you commeted.
– NathanOliver
Nov 26 '18 at 16:01
|
show 4 more comments
A function in C++ can only have a single type that it returns. If you use auto
as the return type and you have different return statements that return different types then the code is ill-formed as it violates the single type rule.
This where std::variant
or std::any
needs to be used. If you have a few different types that could be returned via some run-time value then you could use either of those types as "generic type". std::variant
is more restrictive as you have to specify the types it could be, but it also less expensive then std::any
because you know what types it could be.
std::variant<double, int, std::string> function_name (int value) {
using namespace std::literals::string_literals;
switch (value) {
case 1 : {return 2.3;}
case 2 : {return 1;}
case 3 : {return "string"s;} // use ""s here to force it to be a std::string
}
}
Will let you return different types.
1
std::variant<auto>
would be pretty nice, though.
– Bartek Banachewicz
Nov 26 '18 at 15:57
3
@BartekBanachewicz And have it gather all the types the return statements use? That would be cool.
– NathanOliver
Nov 26 '18 at 15:57
1
would have to change"string"
by"string"s
though then.
– Jarod42
Nov 26 '18 at 15:59
1
IMO this answer should mention that this is possibly/likely the wrong way.variant
is the right tool in specific cases, but it can easily be an indicator of a design flaw. Yes, I know, you can't tell exactly from the question, but I find it worth mentioning. Otherwise the asker is potentially left trying to recover from halfway (or fully, withany
) leaving the type system which leads to more abuse.
– Max Langhof
Nov 26 '18 at 16:00
@Jarod42 That was bugging me too. I was editing when you commeted.
– NathanOliver
Nov 26 '18 at 16:01
|
show 4 more comments
A function in C++ can only have a single type that it returns. If you use auto
as the return type and you have different return statements that return different types then the code is ill-formed as it violates the single type rule.
This where std::variant
or std::any
needs to be used. If you have a few different types that could be returned via some run-time value then you could use either of those types as "generic type". std::variant
is more restrictive as you have to specify the types it could be, but it also less expensive then std::any
because you know what types it could be.
std::variant<double, int, std::string> function_name (int value) {
using namespace std::literals::string_literals;
switch (value) {
case 1 : {return 2.3;}
case 2 : {return 1;}
case 3 : {return "string"s;} // use ""s here to force it to be a std::string
}
}
Will let you return different types.
A function in C++ can only have a single type that it returns. If you use auto
as the return type and you have different return statements that return different types then the code is ill-formed as it violates the single type rule.
This where std::variant
or std::any
needs to be used. If you have a few different types that could be returned via some run-time value then you could use either of those types as "generic type". std::variant
is more restrictive as you have to specify the types it could be, but it also less expensive then std::any
because you know what types it could be.
std::variant<double, int, std::string> function_name (int value) {
using namespace std::literals::string_literals;
switch (value) {
case 1 : {return 2.3;}
case 2 : {return 1;}
case 3 : {return "string"s;} // use ""s here to force it to be a std::string
}
}
Will let you return different types.
edited Nov 26 '18 at 16:10
answered Nov 26 '18 at 15:52


NathanOliverNathanOliver
92.4k16129195
92.4k16129195
1
std::variant<auto>
would be pretty nice, though.
– Bartek Banachewicz
Nov 26 '18 at 15:57
3
@BartekBanachewicz And have it gather all the types the return statements use? That would be cool.
– NathanOliver
Nov 26 '18 at 15:57
1
would have to change"string"
by"string"s
though then.
– Jarod42
Nov 26 '18 at 15:59
1
IMO this answer should mention that this is possibly/likely the wrong way.variant
is the right tool in specific cases, but it can easily be an indicator of a design flaw. Yes, I know, you can't tell exactly from the question, but I find it worth mentioning. Otherwise the asker is potentially left trying to recover from halfway (or fully, withany
) leaving the type system which leads to more abuse.
– Max Langhof
Nov 26 '18 at 16:00
@Jarod42 That was bugging me too. I was editing when you commeted.
– NathanOliver
Nov 26 '18 at 16:01
|
show 4 more comments
1
std::variant<auto>
would be pretty nice, though.
– Bartek Banachewicz
Nov 26 '18 at 15:57
3
@BartekBanachewicz And have it gather all the types the return statements use? That would be cool.
– NathanOliver
Nov 26 '18 at 15:57
1
would have to change"string"
by"string"s
though then.
– Jarod42
Nov 26 '18 at 15:59
1
IMO this answer should mention that this is possibly/likely the wrong way.variant
is the right tool in specific cases, but it can easily be an indicator of a design flaw. Yes, I know, you can't tell exactly from the question, but I find it worth mentioning. Otherwise the asker is potentially left trying to recover from halfway (or fully, withany
) leaving the type system which leads to more abuse.
– Max Langhof
Nov 26 '18 at 16:00
@Jarod42 That was bugging me too. I was editing when you commeted.
– NathanOliver
Nov 26 '18 at 16:01
1
1
std::variant<auto>
would be pretty nice, though.– Bartek Banachewicz
Nov 26 '18 at 15:57
std::variant<auto>
would be pretty nice, though.– Bartek Banachewicz
Nov 26 '18 at 15:57
3
3
@BartekBanachewicz And have it gather all the types the return statements use? That would be cool.
– NathanOliver
Nov 26 '18 at 15:57
@BartekBanachewicz And have it gather all the types the return statements use? That would be cool.
– NathanOliver
Nov 26 '18 at 15:57
1
1
would have to change
"string"
by "string"s
though then.– Jarod42
Nov 26 '18 at 15:59
would have to change
"string"
by "string"s
though then.– Jarod42
Nov 26 '18 at 15:59
1
1
IMO this answer should mention that this is possibly/likely the wrong way.
variant
is the right tool in specific cases, but it can easily be an indicator of a design flaw. Yes, I know, you can't tell exactly from the question, but I find it worth mentioning. Otherwise the asker is potentially left trying to recover from halfway (or fully, with any
) leaving the type system which leads to more abuse.– Max Langhof
Nov 26 '18 at 16:00
IMO this answer should mention that this is possibly/likely the wrong way.
variant
is the right tool in specific cases, but it can easily be an indicator of a design flaw. Yes, I know, you can't tell exactly from the question, but I find it worth mentioning. Otherwise the asker is potentially left trying to recover from halfway (or fully, with any
) leaving the type system which leads to more abuse.– Max Langhof
Nov 26 '18 at 16:00
@Jarod42 That was bugging me too. I was editing when you commeted.
– NathanOliver
Nov 26 '18 at 16:01
@Jarod42 That was bugging me too. I was editing when you commeted.
– NathanOliver
Nov 26 '18 at 16:01
|
show 4 more comments
If the function argument is known at compile time, you can use a compile time dispatch like e.g.
template <int N>
constexpr auto function_name()
{
if constexpr(N == 1)
return 2.3;
else if constexpr (N == 2)
return 1;
else
return "string";
}
which can be instantiated and invoked as follows
std::cout << function_name<1>() << "n";
C++17 is necessary for the if constexpr
part. Note that when binding the return value to a variable, carefully choose the type (e.g. to not implicitly convert a double
to an int
by accident), use type deduction or a variant
-type as shown in the existing answers.
Note that as @NathanOliver pointed out in the comments, there is also a pre-C++17 solution that uses template specialization instead of if constexpr
:
template <int N> constexpr auto function_name() { return "string"; }
template <> constexpr auto function_name<1>() { return 2.3; }
template <> constexpr auto function_name<2>() { return 1; }
The usage of this template and its specializations does not differ from the above.
add a comment |
If the function argument is known at compile time, you can use a compile time dispatch like e.g.
template <int N>
constexpr auto function_name()
{
if constexpr(N == 1)
return 2.3;
else if constexpr (N == 2)
return 1;
else
return "string";
}
which can be instantiated and invoked as follows
std::cout << function_name<1>() << "n";
C++17 is necessary for the if constexpr
part. Note that when binding the return value to a variable, carefully choose the type (e.g. to not implicitly convert a double
to an int
by accident), use type deduction or a variant
-type as shown in the existing answers.
Note that as @NathanOliver pointed out in the comments, there is also a pre-C++17 solution that uses template specialization instead of if constexpr
:
template <int N> constexpr auto function_name() { return "string"; }
template <> constexpr auto function_name<1>() { return 2.3; }
template <> constexpr auto function_name<2>() { return 1; }
The usage of this template and its specializations does not differ from the above.
add a comment |
If the function argument is known at compile time, you can use a compile time dispatch like e.g.
template <int N>
constexpr auto function_name()
{
if constexpr(N == 1)
return 2.3;
else if constexpr (N == 2)
return 1;
else
return "string";
}
which can be instantiated and invoked as follows
std::cout << function_name<1>() << "n";
C++17 is necessary for the if constexpr
part. Note that when binding the return value to a variable, carefully choose the type (e.g. to not implicitly convert a double
to an int
by accident), use type deduction or a variant
-type as shown in the existing answers.
Note that as @NathanOliver pointed out in the comments, there is also a pre-C++17 solution that uses template specialization instead of if constexpr
:
template <int N> constexpr auto function_name() { return "string"; }
template <> constexpr auto function_name<1>() { return 2.3; }
template <> constexpr auto function_name<2>() { return 1; }
The usage of this template and its specializations does not differ from the above.
If the function argument is known at compile time, you can use a compile time dispatch like e.g.
template <int N>
constexpr auto function_name()
{
if constexpr(N == 1)
return 2.3;
else if constexpr (N == 2)
return 1;
else
return "string";
}
which can be instantiated and invoked as follows
std::cout << function_name<1>() << "n";
C++17 is necessary for the if constexpr
part. Note that when binding the return value to a variable, carefully choose the type (e.g. to not implicitly convert a double
to an int
by accident), use type deduction or a variant
-type as shown in the existing answers.
Note that as @NathanOliver pointed out in the comments, there is also a pre-C++17 solution that uses template specialization instead of if constexpr
:
template <int N> constexpr auto function_name() { return "string"; }
template <> constexpr auto function_name<1>() { return 2.3; }
template <> constexpr auto function_name<2>() { return 1; }
The usage of this template and its specializations does not differ from the above.
edited Nov 26 '18 at 16:19
answered Nov 26 '18 at 15:53


lubgrlubgr
11.9k21846
11.9k21846
add a comment |
add a comment |
The error message says it all: all branches of the function must return the same type. This limitation is not specific to auto
return type.
One possible fix:
std::variant<double, int, std::string> function_name(int value) {
switch(value) {
case 1 : return 2.3;
case 2 : return 1;
case 3 : return "string";
default: throw;
}
}
Alternatively, you can use boost::variant
.
There is a standard variant type now.
– François Andrieux
Nov 26 '18 at 16:18
add a comment |
The error message says it all: all branches of the function must return the same type. This limitation is not specific to auto
return type.
One possible fix:
std::variant<double, int, std::string> function_name(int value) {
switch(value) {
case 1 : return 2.3;
case 2 : return 1;
case 3 : return "string";
default: throw;
}
}
Alternatively, you can use boost::variant
.
There is a standard variant type now.
– François Andrieux
Nov 26 '18 at 16:18
add a comment |
The error message says it all: all branches of the function must return the same type. This limitation is not specific to auto
return type.
One possible fix:
std::variant<double, int, std::string> function_name(int value) {
switch(value) {
case 1 : return 2.3;
case 2 : return 1;
case 3 : return "string";
default: throw;
}
}
Alternatively, you can use boost::variant
.
The error message says it all: all branches of the function must return the same type. This limitation is not specific to auto
return type.
One possible fix:
std::variant<double, int, std::string> function_name(int value) {
switch(value) {
case 1 : return 2.3;
case 2 : return 1;
case 3 : return "string";
default: throw;
}
}
Alternatively, you can use boost::variant
.
edited Nov 26 '18 at 16:32
answered Nov 26 '18 at 15:51
Maxim EgorushkinMaxim Egorushkin
87.7k11102186
87.7k11102186
There is a standard variant type now.
– François Andrieux
Nov 26 '18 at 16:18
add a comment |
There is a standard variant type now.
– François Andrieux
Nov 26 '18 at 16:18
There is a standard variant type now.
– François Andrieux
Nov 26 '18 at 16:18
There is a standard variant type now.
– François Andrieux
Nov 26 '18 at 16:18
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53484672%2fauto-function-returning-value-using-switch%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
GSgf7,S4fHX,6ct7LJDAX1BboGoUL8jqIrAq GT9z eMyG53PBE8GoGo F99XW VMZTDTKY7IdgR
3
You can't do this for multiple reasons.
auto
has to be known at compile time.– drescherjm
Nov 26 '18 at 15:48
1
C++
is a statically typed language.– imreal
Nov 26 '18 at 15:48
4
You might be interested in
std::variant
.– HolyBlackCat
Nov 26 '18 at 15:50
4
What actual problem are you trying to solve with this? That is simply not how types work in C++. While you could use a std::variant, I'm hesitant to recommend it.
– paddy
Nov 26 '18 at 15:50