Allow button click whilst do loop is true
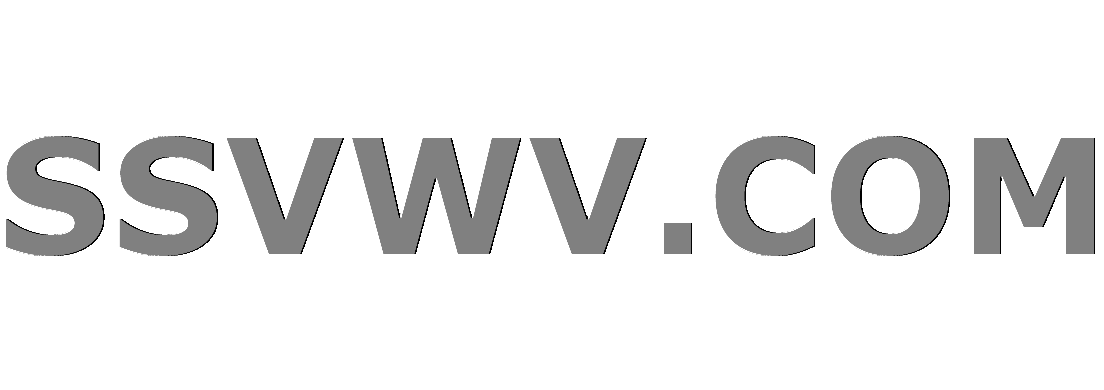
Multi tool use
I created a little RPG at the request of my 8 year old in order to learn javascript and teach her a bit too. It's taken a while (be gentle with my code!) and it's fairly simple but it works well enough by assigning a random enemy for you to fight and then doing a simple combat sequence (round 1, round 2, round 3 etc.) until you or the enemy is dead (runs in a do while loop).
What I'd like to do is have each round activated by a 'FIGHT!' button, providing you or the enemy have > 0 health. I've fiddled around with the code but can't seem to get it to wait between rounds for the button to be pressed - it just runs through each round in one go (as I'd expect it to!).
<h1><b>Halloween RPG Battle</b></h1>
<script>
var enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
var hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
function battle() {
var x = 1;
var randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
do {
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
document.write("<br>" + "<b>" + "Round " + x++ + "</b>");
document.write("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
document.write("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
document.write("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
document.write("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
document.write("<br>" + "You have been killed by the " + randomEnemy.name);
break;
} else {
document.write("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
document.write("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
document.write("<br>" + "Mary did " + heroDamage + " damage");
document.write("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
if (randomEnemy.health <= 0) {
document.write("<br>" + "The " + randomEnemy.name + " is dead!");
break;
} else {
continue;
}
}
while (hero[0].health > 0 || randomEnemy.health > 0);
}
battle()
</script>
javascript
add a comment |
I created a little RPG at the request of my 8 year old in order to learn javascript and teach her a bit too. It's taken a while (be gentle with my code!) and it's fairly simple but it works well enough by assigning a random enemy for you to fight and then doing a simple combat sequence (round 1, round 2, round 3 etc.) until you or the enemy is dead (runs in a do while loop).
What I'd like to do is have each round activated by a 'FIGHT!' button, providing you or the enemy have > 0 health. I've fiddled around with the code but can't seem to get it to wait between rounds for the button to be pressed - it just runs through each round in one go (as I'd expect it to!).
<h1><b>Halloween RPG Battle</b></h1>
<script>
var enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
var hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
function battle() {
var x = 1;
var randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
do {
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
document.write("<br>" + "<b>" + "Round " + x++ + "</b>");
document.write("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
document.write("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
document.write("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
document.write("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
document.write("<br>" + "You have been killed by the " + randomEnemy.name);
break;
} else {
document.write("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
document.write("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
document.write("<br>" + "Mary did " + heroDamage + " damage");
document.write("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
if (randomEnemy.health <= 0) {
document.write("<br>" + "The " + randomEnemy.name + " is dead!");
break;
} else {
continue;
}
}
while (hero[0].health > 0 || randomEnemy.health > 0);
}
battle()
</script>
javascript
1
1: the title needs to be in title tags when in the head, otherwise a H1 belongs in the body 2: Where is the fight button in the HTML? Please click edit and fix the snippet I made to reflect your html. 3: NEVER document.write after the page has loaded . I could not put your script in the pane for script for the same reason. Use innerHTML instead
– mplungjan
Nov 26 '18 at 15:53
2
Remove the while-loop from the battle(), then add a check at the end of the battle() that checks if there's enough health left for the fight. That way you have to click 'battle' for each round of fighting and you can redirect to a different function once the battle is won by someone.
– Shilly
Nov 26 '18 at 15:54
1
tryconfirm("Press a button!");
to fight
– javimovi
Nov 26 '18 at 16:00
mplungjan = Sorry, im about as new to Stack Exchange as coding :) I meant to take all those button code out as it wasnt working, but I obviously left a bit in. confused.com. Thanks for your observation on document.write - Im just going with what works (you should see it before it got to this point!) Shilly - thanks Ill try that first when I have a moment......
– user2497517
Nov 26 '18 at 16:01
add a comment |
I created a little RPG at the request of my 8 year old in order to learn javascript and teach her a bit too. It's taken a while (be gentle with my code!) and it's fairly simple but it works well enough by assigning a random enemy for you to fight and then doing a simple combat sequence (round 1, round 2, round 3 etc.) until you or the enemy is dead (runs in a do while loop).
What I'd like to do is have each round activated by a 'FIGHT!' button, providing you or the enemy have > 0 health. I've fiddled around with the code but can't seem to get it to wait between rounds for the button to be pressed - it just runs through each round in one go (as I'd expect it to!).
<h1><b>Halloween RPG Battle</b></h1>
<script>
var enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
var hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
function battle() {
var x = 1;
var randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
do {
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
document.write("<br>" + "<b>" + "Round " + x++ + "</b>");
document.write("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
document.write("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
document.write("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
document.write("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
document.write("<br>" + "You have been killed by the " + randomEnemy.name);
break;
} else {
document.write("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
document.write("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
document.write("<br>" + "Mary did " + heroDamage + " damage");
document.write("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
if (randomEnemy.health <= 0) {
document.write("<br>" + "The " + randomEnemy.name + " is dead!");
break;
} else {
continue;
}
}
while (hero[0].health > 0 || randomEnemy.health > 0);
}
battle()
</script>
javascript
I created a little RPG at the request of my 8 year old in order to learn javascript and teach her a bit too. It's taken a while (be gentle with my code!) and it's fairly simple but it works well enough by assigning a random enemy for you to fight and then doing a simple combat sequence (round 1, round 2, round 3 etc.) until you or the enemy is dead (runs in a do while loop).
What I'd like to do is have each round activated by a 'FIGHT!' button, providing you or the enemy have > 0 health. I've fiddled around with the code but can't seem to get it to wait between rounds for the button to be pressed - it just runs through each round in one go (as I'd expect it to!).
<h1><b>Halloween RPG Battle</b></h1>
<script>
var enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
var hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
function battle() {
var x = 1;
var randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
do {
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
document.write("<br>" + "<b>" + "Round " + x++ + "</b>");
document.write("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
document.write("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
document.write("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
document.write("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
document.write("<br>" + "You have been killed by the " + randomEnemy.name);
break;
} else {
document.write("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
document.write("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
document.write("<br>" + "Mary did " + heroDamage + " damage");
document.write("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
if (randomEnemy.health <= 0) {
document.write("<br>" + "The " + randomEnemy.name + " is dead!");
break;
} else {
continue;
}
}
while (hero[0].health > 0 || randomEnemy.health > 0);
}
battle()
</script>
<h1><b>Halloween RPG Battle</b></h1>
<script>
var enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
var hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
function battle() {
var x = 1;
var randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
do {
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
document.write("<br>" + "<b>" + "Round " + x++ + "</b>");
document.write("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
document.write("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
document.write("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
document.write("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
document.write("<br>" + "You have been killed by the " + randomEnemy.name);
break;
} else {
document.write("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
document.write("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
document.write("<br>" + "Mary did " + heroDamage + " damage");
document.write("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
if (randomEnemy.health <= 0) {
document.write("<br>" + "The " + randomEnemy.name + " is dead!");
break;
} else {
continue;
}
}
while (hero[0].health > 0 || randomEnemy.health > 0);
}
battle()
</script>
<h1><b>Halloween RPG Battle</b></h1>
<script>
var enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
var hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
function battle() {
var x = 1;
var randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
do {
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
document.write("<br>" + "<b>" + "Round " + x++ + "</b>");
document.write("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
document.write("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
document.write("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
document.write("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
document.write("<br>" + "You have been killed by the " + randomEnemy.name);
break;
} else {
document.write("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
document.write("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
document.write("<br>" + "Mary did " + heroDamage + " damage");
document.write("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
if (randomEnemy.health <= 0) {
document.write("<br>" + "The " + randomEnemy.name + " is dead!");
break;
} else {
continue;
}
}
while (hero[0].health > 0 || randomEnemy.health > 0);
}
battle()
</script>
javascript
javascript
edited Nov 26 '18 at 15:59
user2497517
asked Nov 26 '18 at 15:49
user2497517user2497517
213
213
1
1: the title needs to be in title tags when in the head, otherwise a H1 belongs in the body 2: Where is the fight button in the HTML? Please click edit and fix the snippet I made to reflect your html. 3: NEVER document.write after the page has loaded . I could not put your script in the pane for script for the same reason. Use innerHTML instead
– mplungjan
Nov 26 '18 at 15:53
2
Remove the while-loop from the battle(), then add a check at the end of the battle() that checks if there's enough health left for the fight. That way you have to click 'battle' for each round of fighting and you can redirect to a different function once the battle is won by someone.
– Shilly
Nov 26 '18 at 15:54
1
tryconfirm("Press a button!");
to fight
– javimovi
Nov 26 '18 at 16:00
mplungjan = Sorry, im about as new to Stack Exchange as coding :) I meant to take all those button code out as it wasnt working, but I obviously left a bit in. confused.com. Thanks for your observation on document.write - Im just going with what works (you should see it before it got to this point!) Shilly - thanks Ill try that first when I have a moment......
– user2497517
Nov 26 '18 at 16:01
add a comment |
1
1: the title needs to be in title tags when in the head, otherwise a H1 belongs in the body 2: Where is the fight button in the HTML? Please click edit and fix the snippet I made to reflect your html. 3: NEVER document.write after the page has loaded . I could not put your script in the pane for script for the same reason. Use innerHTML instead
– mplungjan
Nov 26 '18 at 15:53
2
Remove the while-loop from the battle(), then add a check at the end of the battle() that checks if there's enough health left for the fight. That way you have to click 'battle' for each round of fighting and you can redirect to a different function once the battle is won by someone.
– Shilly
Nov 26 '18 at 15:54
1
tryconfirm("Press a button!");
to fight
– javimovi
Nov 26 '18 at 16:00
mplungjan = Sorry, im about as new to Stack Exchange as coding :) I meant to take all those button code out as it wasnt working, but I obviously left a bit in. confused.com. Thanks for your observation on document.write - Im just going with what works (you should see it before it got to this point!) Shilly - thanks Ill try that first when I have a moment......
– user2497517
Nov 26 '18 at 16:01
1
1
1: the title needs to be in title tags when in the head, otherwise a H1 belongs in the body 2: Where is the fight button in the HTML? Please click edit and fix the snippet I made to reflect your html. 3: NEVER document.write after the page has loaded . I could not put your script in the pane for script for the same reason. Use innerHTML instead
– mplungjan
Nov 26 '18 at 15:53
1: the title needs to be in title tags when in the head, otherwise a H1 belongs in the body 2: Where is the fight button in the HTML? Please click edit and fix the snippet I made to reflect your html. 3: NEVER document.write after the page has loaded . I could not put your script in the pane for script for the same reason. Use innerHTML instead
– mplungjan
Nov 26 '18 at 15:53
2
2
Remove the while-loop from the battle(), then add a check at the end of the battle() that checks if there's enough health left for the fight. That way you have to click 'battle' for each round of fighting and you can redirect to a different function once the battle is won by someone.
– Shilly
Nov 26 '18 at 15:54
Remove the while-loop from the battle(), then add a check at the end of the battle() that checks if there's enough health left for the fight. That way you have to click 'battle' for each round of fighting and you can redirect to a different function once the battle is won by someone.
– Shilly
Nov 26 '18 at 15:54
1
1
try
confirm("Press a button!");
to fight– javimovi
Nov 26 '18 at 16:00
try
confirm("Press a button!");
to fight– javimovi
Nov 26 '18 at 16:00
mplungjan = Sorry, im about as new to Stack Exchange as coding :) I meant to take all those button code out as it wasnt working, but I obviously left a bit in. confused.com. Thanks for your observation on document.write - Im just going with what works (you should see it before it got to this point!) Shilly - thanks Ill try that first when I have a moment......
– user2497517
Nov 26 '18 at 16:01
mplungjan = Sorry, im about as new to Stack Exchange as coding :) I meant to take all those button code out as it wasnt working, but I obviously left a bit in. confused.com. Thanks for your observation on document.write - Im just going with what works (you should see it before it got to this point!) Shilly - thanks Ill try that first when I have a moment......
– user2497517
Nov 26 '18 at 16:01
add a comment |
3 Answers
3
active
oldest
votes
Here is my solution. You need to remove the do/while loop and set the randomEnemy outside of your battle method.
To keep the fight button after a Round has completed, I put the content of the round in a container element that is before your button "fight".
const container = document.getElementById("container");
var hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
var randomEnemy = null;
var x = 1;
function pickNextEnemy(){
randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
}
function battle() {
if(hero[0].health <= 0 || randomEnemy.health <= 0){
console.log("can't continue, someone has died");
return;
};
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
container.innerHTML += ("<br>" + "<b>" + "Round " + x++ + "</b>");
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
container.innerHTML += ("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
container.innerHTML += ("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
container.innerHTML += ("<br>" + "You have been killed by the " + randomEnemy.name);
return;
} else {
container.innerHTML += ("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
container.innerHTML += ("<br>" + "Mary did " + heroDamage + " damage");
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
if (randomEnemy.health <= 0) {
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " is dead!");
}
}
pickNextEnemy();
battle()
document.getElementById("fight").addEventListener("click", battle);
<div id="container">
</div>
<button id="fight">Fight!</button>
<script>
var enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
</script>
add a comment |
The answer is to not use a loop at all, but rather have your battle
function just run one iteration of the logical game loop. When the battle is over, you can disable the "Fight" button by setting its disabled
property true
.
The snippet below anticipates that you will eventually want to add other heroes besides Mary, and that you'd like to randomly select those as well. I feel that lists read better if they have plural names, so I renamed "enemy" to "enemies" and "hero" to "heroes". That way, a new variable "hero" can refer to the active hero. Also, I make a copies of the objects from those lists using Object.create
instead of changing the values on those objects directly. That way, you can always reset to the original values.
I hope you and your 8-year-old have fun working on this together! It's got the wheels turning for me. You could add images for the different enemies and load those on reset. There are endless possibilities. Enjoy!
var enemies = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
var heroes = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
function getRandomElement(list) {
return Object.create(list[Math.floor(Math.random() * list.length)]);
}
function getRandomEnemy() {
return getRandomElement(enemies);
}
function getRandomHero() {
return getRandomElement(heroes);
}
var x, randomEnemy, hero;
var output = document.getElementById("output");
var fightBtn = document.getElementById("fight");
var resetBtn = document.getElementById("reset");
fightBtn.addEventListener("click", battle);
function reset() {
x = 1;
randomEnemy = getRandomEnemy();
fightBtn.disabled = false;
hero = getRandomHero();
output.innerHTML = "";
}
resetBtn.addEventListener("click", reset);
reset();
function battle() {
if (hero.health <= 0 || randomEnemy.health <= 0) {
fightBtn.disabled = true;
return;
}
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero.dodge)];
var heroDamage = Math.floor((Math.random() * hero.damage) + 1);
output.innerHTML += "<br>" + "<b>" + "Round " + x++ + "</b>";
output.innerHTML += "<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon;
if (randomNumber < heroDodge) {
output.innerHTML += "<br>" + "You evade the attack!";
} else if (hero.health > 0) {
hero.health = hero.health - enemyDamage;
if (hero.health < 0)
hero.health = 0;
output.innerHTML += "<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage";
output.innerHTML += "<br>" + "You have " + hero.health + " health remaining.";
}
if (hero.health <= 0) {
output.innerHTML += "<br>" + "You have been killed by the " + randomEnemy.name;
fightBtn.disabled = true;
return;
} else {
output.innerHTML += "<br>" + hero.name + " attacks the " + randomEnemy.name + " with their " + hero.weapon;
}
if (randomNumber < enemyDodge) {
output.innerHTML += "<br>" + "The " + randomEnemy.name + " evades the attack!";
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
if (randomEnemy.health < 0)
randomEnemy.health = 0;
output.innerHTML += "<br>" + hero.name + " did " + heroDamage + " damage";
output.innerHTML += "<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health";
}
if (randomEnemy.health <= 0) {
output.innerHTML += "<br>" + "The " + randomEnemy.name + " is dead!";
fightBtn.disabled = true;
}
}
<h1><b>Halloween RPG Battle</b></h1>
<p><button id="fight">Fight</button><button id="reset">Reset</button></p>
<div id="output"></div>
Thanks very much for this. Took me ages to understand and write the original code, now I'm going to have to learn some more :) I've found the hardest thing for me is to get my head round the order code runs and how that relates to how it appears on screen. I'm quite a visual person and being faced by lots of code is hard work!
– user2497517
Nov 27 '18 at 14:29
Could you explain this function getRandomElement(list) ? I see you mention it above but Im at a loss at what it does as I can't see what it reference?
– user2497517
Nov 29 '18 at 15:49
1
Sure. It fetches a random element of the list. Math.random() returns a number between 0 and 1 (exclusive). Multiply that by the number of elements in the list to get something between 0 and that number (exclusive). Math.floor() returns the integer before the number, so you get a valid index
– Austin Mullins
Nov 30 '18 at 19:40
1
Then, I call Object.create() on that element to make a copy of it.
– Austin Mullins
Nov 30 '18 at 19:41
Thanks. I didnt read it carefully enough first time round and failed to realise getRandomElement is called by the other two functions. I've got some time today so hopefully the changes you made will sink in! :) It takes me such a long time to a) Understand concepts and b) remember them a few days later (especially if i have to take a break for a few days). 1 step forwards then 2 back! :(
– user2497517
Dec 2 '18 at 14:29
add a comment |
My main modification is to push the texts to an array and show the array when something happens
NEVER use document.write after load - it will wipe the page and script
Also added a load eventhandler and a reset
function show() {
document.getElementById("action").innerHTML = text.join("");
if (hero[0].health <= 0) {
if (confirm("Reset?")) {
reset();
}
}
}
var enemy,hero;
function reset() {
document.getElementById("action").innerHTML = "Ready for battle!!!";
enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
}
var text = ;
function battle() {
var x = 1;
var randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
text = ; // reset;
text.push("<br>" + "<b>" + "Round " + x++ + "</b>");
text.push("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
text.push("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
text.push("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
text.push("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
text.push("<br>" + "You have been killed by the " + randomEnemy.name);
show();
return
} else {
text.push("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
text.push("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
text.push("<br>" + "Mary did " + heroDamage + " damage");
text.push("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
show();
}
window.addEventListener("load",function() {
reset();
document.getElementById("fight").addEventListener("click", battle);
});
<h1><b>Halloween RPG Battle</b></h1>
<button type="button" id="fight">FIGHT</button>
<div id="action"></div>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53484707%2fallow-button-click-whilst-do-loop-is-true%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Here is my solution. You need to remove the do/while loop and set the randomEnemy outside of your battle method.
To keep the fight button after a Round has completed, I put the content of the round in a container element that is before your button "fight".
const container = document.getElementById("container");
var hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
var randomEnemy = null;
var x = 1;
function pickNextEnemy(){
randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
}
function battle() {
if(hero[0].health <= 0 || randomEnemy.health <= 0){
console.log("can't continue, someone has died");
return;
};
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
container.innerHTML += ("<br>" + "<b>" + "Round " + x++ + "</b>");
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
container.innerHTML += ("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
container.innerHTML += ("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
container.innerHTML += ("<br>" + "You have been killed by the " + randomEnemy.name);
return;
} else {
container.innerHTML += ("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
container.innerHTML += ("<br>" + "Mary did " + heroDamage + " damage");
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
if (randomEnemy.health <= 0) {
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " is dead!");
}
}
pickNextEnemy();
battle()
document.getElementById("fight").addEventListener("click", battle);
<div id="container">
</div>
<button id="fight">Fight!</button>
<script>
var enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
</script>
add a comment |
Here is my solution. You need to remove the do/while loop and set the randomEnemy outside of your battle method.
To keep the fight button after a Round has completed, I put the content of the round in a container element that is before your button "fight".
const container = document.getElementById("container");
var hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
var randomEnemy = null;
var x = 1;
function pickNextEnemy(){
randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
}
function battle() {
if(hero[0].health <= 0 || randomEnemy.health <= 0){
console.log("can't continue, someone has died");
return;
};
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
container.innerHTML += ("<br>" + "<b>" + "Round " + x++ + "</b>");
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
container.innerHTML += ("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
container.innerHTML += ("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
container.innerHTML += ("<br>" + "You have been killed by the " + randomEnemy.name);
return;
} else {
container.innerHTML += ("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
container.innerHTML += ("<br>" + "Mary did " + heroDamage + " damage");
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
if (randomEnemy.health <= 0) {
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " is dead!");
}
}
pickNextEnemy();
battle()
document.getElementById("fight").addEventListener("click", battle);
<div id="container">
</div>
<button id="fight">Fight!</button>
<script>
var enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
</script>
add a comment |
Here is my solution. You need to remove the do/while loop and set the randomEnemy outside of your battle method.
To keep the fight button after a Round has completed, I put the content of the round in a container element that is before your button "fight".
const container = document.getElementById("container");
var hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
var randomEnemy = null;
var x = 1;
function pickNextEnemy(){
randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
}
function battle() {
if(hero[0].health <= 0 || randomEnemy.health <= 0){
console.log("can't continue, someone has died");
return;
};
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
container.innerHTML += ("<br>" + "<b>" + "Round " + x++ + "</b>");
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
container.innerHTML += ("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
container.innerHTML += ("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
container.innerHTML += ("<br>" + "You have been killed by the " + randomEnemy.name);
return;
} else {
container.innerHTML += ("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
container.innerHTML += ("<br>" + "Mary did " + heroDamage + " damage");
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
if (randomEnemy.health <= 0) {
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " is dead!");
}
}
pickNextEnemy();
battle()
document.getElementById("fight").addEventListener("click", battle);
<div id="container">
</div>
<button id="fight">Fight!</button>
<script>
var enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
</script>
Here is my solution. You need to remove the do/while loop and set the randomEnemy outside of your battle method.
To keep the fight button after a Round has completed, I put the content of the round in a container element that is before your button "fight".
const container = document.getElementById("container");
var hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
var randomEnemy = null;
var x = 1;
function pickNextEnemy(){
randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
}
function battle() {
if(hero[0].health <= 0 || randomEnemy.health <= 0){
console.log("can't continue, someone has died");
return;
};
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
container.innerHTML += ("<br>" + "<b>" + "Round " + x++ + "</b>");
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
container.innerHTML += ("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
container.innerHTML += ("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
container.innerHTML += ("<br>" + "You have been killed by the " + randomEnemy.name);
return;
} else {
container.innerHTML += ("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
container.innerHTML += ("<br>" + "Mary did " + heroDamage + " damage");
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
if (randomEnemy.health <= 0) {
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " is dead!");
}
}
pickNextEnemy();
battle()
document.getElementById("fight").addEventListener("click", battle);
<div id="container">
</div>
<button id="fight">Fight!</button>
<script>
var enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
</script>
const container = document.getElementById("container");
var hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
var randomEnemy = null;
var x = 1;
function pickNextEnemy(){
randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
}
function battle() {
if(hero[0].health <= 0 || randomEnemy.health <= 0){
console.log("can't continue, someone has died");
return;
};
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
container.innerHTML += ("<br>" + "<b>" + "Round " + x++ + "</b>");
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
container.innerHTML += ("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
container.innerHTML += ("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
container.innerHTML += ("<br>" + "You have been killed by the " + randomEnemy.name);
return;
} else {
container.innerHTML += ("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
container.innerHTML += ("<br>" + "Mary did " + heroDamage + " damage");
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
if (randomEnemy.health <= 0) {
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " is dead!");
}
}
pickNextEnemy();
battle()
document.getElementById("fight").addEventListener("click", battle);
<div id="container">
</div>
<button id="fight">Fight!</button>
<script>
var enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
</script>
const container = document.getElementById("container");
var hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
var randomEnemy = null;
var x = 1;
function pickNextEnemy(){
randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
}
function battle() {
if(hero[0].health <= 0 || randomEnemy.health <= 0){
console.log("can't continue, someone has died");
return;
};
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
container.innerHTML += ("<br>" + "<b>" + "Round " + x++ + "</b>");
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
container.innerHTML += ("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
container.innerHTML += ("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
container.innerHTML += ("<br>" + "You have been killed by the " + randomEnemy.name);
return;
} else {
container.innerHTML += ("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
container.innerHTML += ("<br>" + "Mary did " + heroDamage + " damage");
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
if (randomEnemy.health <= 0) {
container.innerHTML += ("<br>" + "The " + randomEnemy.name + " is dead!");
}
}
pickNextEnemy();
battle()
document.getElementById("fight").addEventListener("click", battle);
<div id="container">
</div>
<button id="fight">Fight!</button>
<script>
var enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
</script>
answered Nov 26 '18 at 16:14


kemicofakemicofa
10k43881
10k43881
add a comment |
add a comment |
The answer is to not use a loop at all, but rather have your battle
function just run one iteration of the logical game loop. When the battle is over, you can disable the "Fight" button by setting its disabled
property true
.
The snippet below anticipates that you will eventually want to add other heroes besides Mary, and that you'd like to randomly select those as well. I feel that lists read better if they have plural names, so I renamed "enemy" to "enemies" and "hero" to "heroes". That way, a new variable "hero" can refer to the active hero. Also, I make a copies of the objects from those lists using Object.create
instead of changing the values on those objects directly. That way, you can always reset to the original values.
I hope you and your 8-year-old have fun working on this together! It's got the wheels turning for me. You could add images for the different enemies and load those on reset. There are endless possibilities. Enjoy!
var enemies = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
var heroes = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
function getRandomElement(list) {
return Object.create(list[Math.floor(Math.random() * list.length)]);
}
function getRandomEnemy() {
return getRandomElement(enemies);
}
function getRandomHero() {
return getRandomElement(heroes);
}
var x, randomEnemy, hero;
var output = document.getElementById("output");
var fightBtn = document.getElementById("fight");
var resetBtn = document.getElementById("reset");
fightBtn.addEventListener("click", battle);
function reset() {
x = 1;
randomEnemy = getRandomEnemy();
fightBtn.disabled = false;
hero = getRandomHero();
output.innerHTML = "";
}
resetBtn.addEventListener("click", reset);
reset();
function battle() {
if (hero.health <= 0 || randomEnemy.health <= 0) {
fightBtn.disabled = true;
return;
}
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero.dodge)];
var heroDamage = Math.floor((Math.random() * hero.damage) + 1);
output.innerHTML += "<br>" + "<b>" + "Round " + x++ + "</b>";
output.innerHTML += "<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon;
if (randomNumber < heroDodge) {
output.innerHTML += "<br>" + "You evade the attack!";
} else if (hero.health > 0) {
hero.health = hero.health - enemyDamage;
if (hero.health < 0)
hero.health = 0;
output.innerHTML += "<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage";
output.innerHTML += "<br>" + "You have " + hero.health + " health remaining.";
}
if (hero.health <= 0) {
output.innerHTML += "<br>" + "You have been killed by the " + randomEnemy.name;
fightBtn.disabled = true;
return;
} else {
output.innerHTML += "<br>" + hero.name + " attacks the " + randomEnemy.name + " with their " + hero.weapon;
}
if (randomNumber < enemyDodge) {
output.innerHTML += "<br>" + "The " + randomEnemy.name + " evades the attack!";
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
if (randomEnemy.health < 0)
randomEnemy.health = 0;
output.innerHTML += "<br>" + hero.name + " did " + heroDamage + " damage";
output.innerHTML += "<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health";
}
if (randomEnemy.health <= 0) {
output.innerHTML += "<br>" + "The " + randomEnemy.name + " is dead!";
fightBtn.disabled = true;
}
}
<h1><b>Halloween RPG Battle</b></h1>
<p><button id="fight">Fight</button><button id="reset">Reset</button></p>
<div id="output"></div>
Thanks very much for this. Took me ages to understand and write the original code, now I'm going to have to learn some more :) I've found the hardest thing for me is to get my head round the order code runs and how that relates to how it appears on screen. I'm quite a visual person and being faced by lots of code is hard work!
– user2497517
Nov 27 '18 at 14:29
Could you explain this function getRandomElement(list) ? I see you mention it above but Im at a loss at what it does as I can't see what it reference?
– user2497517
Nov 29 '18 at 15:49
1
Sure. It fetches a random element of the list. Math.random() returns a number between 0 and 1 (exclusive). Multiply that by the number of elements in the list to get something between 0 and that number (exclusive). Math.floor() returns the integer before the number, so you get a valid index
– Austin Mullins
Nov 30 '18 at 19:40
1
Then, I call Object.create() on that element to make a copy of it.
– Austin Mullins
Nov 30 '18 at 19:41
Thanks. I didnt read it carefully enough first time round and failed to realise getRandomElement is called by the other two functions. I've got some time today so hopefully the changes you made will sink in! :) It takes me such a long time to a) Understand concepts and b) remember them a few days later (especially if i have to take a break for a few days). 1 step forwards then 2 back! :(
– user2497517
Dec 2 '18 at 14:29
add a comment |
The answer is to not use a loop at all, but rather have your battle
function just run one iteration of the logical game loop. When the battle is over, you can disable the "Fight" button by setting its disabled
property true
.
The snippet below anticipates that you will eventually want to add other heroes besides Mary, and that you'd like to randomly select those as well. I feel that lists read better if they have plural names, so I renamed "enemy" to "enemies" and "hero" to "heroes". That way, a new variable "hero" can refer to the active hero. Also, I make a copies of the objects from those lists using Object.create
instead of changing the values on those objects directly. That way, you can always reset to the original values.
I hope you and your 8-year-old have fun working on this together! It's got the wheels turning for me. You could add images for the different enemies and load those on reset. There are endless possibilities. Enjoy!
var enemies = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
var heroes = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
function getRandomElement(list) {
return Object.create(list[Math.floor(Math.random() * list.length)]);
}
function getRandomEnemy() {
return getRandomElement(enemies);
}
function getRandomHero() {
return getRandomElement(heroes);
}
var x, randomEnemy, hero;
var output = document.getElementById("output");
var fightBtn = document.getElementById("fight");
var resetBtn = document.getElementById("reset");
fightBtn.addEventListener("click", battle);
function reset() {
x = 1;
randomEnemy = getRandomEnemy();
fightBtn.disabled = false;
hero = getRandomHero();
output.innerHTML = "";
}
resetBtn.addEventListener("click", reset);
reset();
function battle() {
if (hero.health <= 0 || randomEnemy.health <= 0) {
fightBtn.disabled = true;
return;
}
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero.dodge)];
var heroDamage = Math.floor((Math.random() * hero.damage) + 1);
output.innerHTML += "<br>" + "<b>" + "Round " + x++ + "</b>";
output.innerHTML += "<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon;
if (randomNumber < heroDodge) {
output.innerHTML += "<br>" + "You evade the attack!";
} else if (hero.health > 0) {
hero.health = hero.health - enemyDamage;
if (hero.health < 0)
hero.health = 0;
output.innerHTML += "<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage";
output.innerHTML += "<br>" + "You have " + hero.health + " health remaining.";
}
if (hero.health <= 0) {
output.innerHTML += "<br>" + "You have been killed by the " + randomEnemy.name;
fightBtn.disabled = true;
return;
} else {
output.innerHTML += "<br>" + hero.name + " attacks the " + randomEnemy.name + " with their " + hero.weapon;
}
if (randomNumber < enemyDodge) {
output.innerHTML += "<br>" + "The " + randomEnemy.name + " evades the attack!";
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
if (randomEnemy.health < 0)
randomEnemy.health = 0;
output.innerHTML += "<br>" + hero.name + " did " + heroDamage + " damage";
output.innerHTML += "<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health";
}
if (randomEnemy.health <= 0) {
output.innerHTML += "<br>" + "The " + randomEnemy.name + " is dead!";
fightBtn.disabled = true;
}
}
<h1><b>Halloween RPG Battle</b></h1>
<p><button id="fight">Fight</button><button id="reset">Reset</button></p>
<div id="output"></div>
Thanks very much for this. Took me ages to understand and write the original code, now I'm going to have to learn some more :) I've found the hardest thing for me is to get my head round the order code runs and how that relates to how it appears on screen. I'm quite a visual person and being faced by lots of code is hard work!
– user2497517
Nov 27 '18 at 14:29
Could you explain this function getRandomElement(list) ? I see you mention it above but Im at a loss at what it does as I can't see what it reference?
– user2497517
Nov 29 '18 at 15:49
1
Sure. It fetches a random element of the list. Math.random() returns a number between 0 and 1 (exclusive). Multiply that by the number of elements in the list to get something between 0 and that number (exclusive). Math.floor() returns the integer before the number, so you get a valid index
– Austin Mullins
Nov 30 '18 at 19:40
1
Then, I call Object.create() on that element to make a copy of it.
– Austin Mullins
Nov 30 '18 at 19:41
Thanks. I didnt read it carefully enough first time round and failed to realise getRandomElement is called by the other two functions. I've got some time today so hopefully the changes you made will sink in! :) It takes me such a long time to a) Understand concepts and b) remember them a few days later (especially if i have to take a break for a few days). 1 step forwards then 2 back! :(
– user2497517
Dec 2 '18 at 14:29
add a comment |
The answer is to not use a loop at all, but rather have your battle
function just run one iteration of the logical game loop. When the battle is over, you can disable the "Fight" button by setting its disabled
property true
.
The snippet below anticipates that you will eventually want to add other heroes besides Mary, and that you'd like to randomly select those as well. I feel that lists read better if they have plural names, so I renamed "enemy" to "enemies" and "hero" to "heroes". That way, a new variable "hero" can refer to the active hero. Also, I make a copies of the objects from those lists using Object.create
instead of changing the values on those objects directly. That way, you can always reset to the original values.
I hope you and your 8-year-old have fun working on this together! It's got the wheels turning for me. You could add images for the different enemies and load those on reset. There are endless possibilities. Enjoy!
var enemies = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
var heroes = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
function getRandomElement(list) {
return Object.create(list[Math.floor(Math.random() * list.length)]);
}
function getRandomEnemy() {
return getRandomElement(enemies);
}
function getRandomHero() {
return getRandomElement(heroes);
}
var x, randomEnemy, hero;
var output = document.getElementById("output");
var fightBtn = document.getElementById("fight");
var resetBtn = document.getElementById("reset");
fightBtn.addEventListener("click", battle);
function reset() {
x = 1;
randomEnemy = getRandomEnemy();
fightBtn.disabled = false;
hero = getRandomHero();
output.innerHTML = "";
}
resetBtn.addEventListener("click", reset);
reset();
function battle() {
if (hero.health <= 0 || randomEnemy.health <= 0) {
fightBtn.disabled = true;
return;
}
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero.dodge)];
var heroDamage = Math.floor((Math.random() * hero.damage) + 1);
output.innerHTML += "<br>" + "<b>" + "Round " + x++ + "</b>";
output.innerHTML += "<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon;
if (randomNumber < heroDodge) {
output.innerHTML += "<br>" + "You evade the attack!";
} else if (hero.health > 0) {
hero.health = hero.health - enemyDamage;
if (hero.health < 0)
hero.health = 0;
output.innerHTML += "<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage";
output.innerHTML += "<br>" + "You have " + hero.health + " health remaining.";
}
if (hero.health <= 0) {
output.innerHTML += "<br>" + "You have been killed by the " + randomEnemy.name;
fightBtn.disabled = true;
return;
} else {
output.innerHTML += "<br>" + hero.name + " attacks the " + randomEnemy.name + " with their " + hero.weapon;
}
if (randomNumber < enemyDodge) {
output.innerHTML += "<br>" + "The " + randomEnemy.name + " evades the attack!";
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
if (randomEnemy.health < 0)
randomEnemy.health = 0;
output.innerHTML += "<br>" + hero.name + " did " + heroDamage + " damage";
output.innerHTML += "<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health";
}
if (randomEnemy.health <= 0) {
output.innerHTML += "<br>" + "The " + randomEnemy.name + " is dead!";
fightBtn.disabled = true;
}
}
<h1><b>Halloween RPG Battle</b></h1>
<p><button id="fight">Fight</button><button id="reset">Reset</button></p>
<div id="output"></div>
The answer is to not use a loop at all, but rather have your battle
function just run one iteration of the logical game loop. When the battle is over, you can disable the "Fight" button by setting its disabled
property true
.
The snippet below anticipates that you will eventually want to add other heroes besides Mary, and that you'd like to randomly select those as well. I feel that lists read better if they have plural names, so I renamed "enemy" to "enemies" and "hero" to "heroes". That way, a new variable "hero" can refer to the active hero. Also, I make a copies of the objects from those lists using Object.create
instead of changing the values on those objects directly. That way, you can always reset to the original values.
I hope you and your 8-year-old have fun working on this together! It's got the wheels turning for me. You could add images for the different enemies and load those on reset. There are endless possibilities. Enjoy!
var enemies = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
var heroes = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
function getRandomElement(list) {
return Object.create(list[Math.floor(Math.random() * list.length)]);
}
function getRandomEnemy() {
return getRandomElement(enemies);
}
function getRandomHero() {
return getRandomElement(heroes);
}
var x, randomEnemy, hero;
var output = document.getElementById("output");
var fightBtn = document.getElementById("fight");
var resetBtn = document.getElementById("reset");
fightBtn.addEventListener("click", battle);
function reset() {
x = 1;
randomEnemy = getRandomEnemy();
fightBtn.disabled = false;
hero = getRandomHero();
output.innerHTML = "";
}
resetBtn.addEventListener("click", reset);
reset();
function battle() {
if (hero.health <= 0 || randomEnemy.health <= 0) {
fightBtn.disabled = true;
return;
}
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero.dodge)];
var heroDamage = Math.floor((Math.random() * hero.damage) + 1);
output.innerHTML += "<br>" + "<b>" + "Round " + x++ + "</b>";
output.innerHTML += "<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon;
if (randomNumber < heroDodge) {
output.innerHTML += "<br>" + "You evade the attack!";
} else if (hero.health > 0) {
hero.health = hero.health - enemyDamage;
if (hero.health < 0)
hero.health = 0;
output.innerHTML += "<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage";
output.innerHTML += "<br>" + "You have " + hero.health + " health remaining.";
}
if (hero.health <= 0) {
output.innerHTML += "<br>" + "You have been killed by the " + randomEnemy.name;
fightBtn.disabled = true;
return;
} else {
output.innerHTML += "<br>" + hero.name + " attacks the " + randomEnemy.name + " with their " + hero.weapon;
}
if (randomNumber < enemyDodge) {
output.innerHTML += "<br>" + "The " + randomEnemy.name + " evades the attack!";
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
if (randomEnemy.health < 0)
randomEnemy.health = 0;
output.innerHTML += "<br>" + hero.name + " did " + heroDamage + " damage";
output.innerHTML += "<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health";
}
if (randomEnemy.health <= 0) {
output.innerHTML += "<br>" + "The " + randomEnemy.name + " is dead!";
fightBtn.disabled = true;
}
}
<h1><b>Halloween RPG Battle</b></h1>
<p><button id="fight">Fight</button><button id="reset">Reset</button></p>
<div id="output"></div>
var enemies = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
var heroes = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
function getRandomElement(list) {
return Object.create(list[Math.floor(Math.random() * list.length)]);
}
function getRandomEnemy() {
return getRandomElement(enemies);
}
function getRandomHero() {
return getRandomElement(heroes);
}
var x, randomEnemy, hero;
var output = document.getElementById("output");
var fightBtn = document.getElementById("fight");
var resetBtn = document.getElementById("reset");
fightBtn.addEventListener("click", battle);
function reset() {
x = 1;
randomEnemy = getRandomEnemy();
fightBtn.disabled = false;
hero = getRandomHero();
output.innerHTML = "";
}
resetBtn.addEventListener("click", reset);
reset();
function battle() {
if (hero.health <= 0 || randomEnemy.health <= 0) {
fightBtn.disabled = true;
return;
}
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero.dodge)];
var heroDamage = Math.floor((Math.random() * hero.damage) + 1);
output.innerHTML += "<br>" + "<b>" + "Round " + x++ + "</b>";
output.innerHTML += "<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon;
if (randomNumber < heroDodge) {
output.innerHTML += "<br>" + "You evade the attack!";
} else if (hero.health > 0) {
hero.health = hero.health - enemyDamage;
if (hero.health < 0)
hero.health = 0;
output.innerHTML += "<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage";
output.innerHTML += "<br>" + "You have " + hero.health + " health remaining.";
}
if (hero.health <= 0) {
output.innerHTML += "<br>" + "You have been killed by the " + randomEnemy.name;
fightBtn.disabled = true;
return;
} else {
output.innerHTML += "<br>" + hero.name + " attacks the " + randomEnemy.name + " with their " + hero.weapon;
}
if (randomNumber < enemyDodge) {
output.innerHTML += "<br>" + "The " + randomEnemy.name + " evades the attack!";
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
if (randomEnemy.health < 0)
randomEnemy.health = 0;
output.innerHTML += "<br>" + hero.name + " did " + heroDamage + " damage";
output.innerHTML += "<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health";
}
if (randomEnemy.health <= 0) {
output.innerHTML += "<br>" + "The " + randomEnemy.name + " is dead!";
fightBtn.disabled = true;
}
}
<h1><b>Halloween RPG Battle</b></h1>
<p><button id="fight">Fight</button><button id="reset">Reset</button></p>
<div id="output"></div>
var enemies = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
var heroes = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
function getRandomElement(list) {
return Object.create(list[Math.floor(Math.random() * list.length)]);
}
function getRandomEnemy() {
return getRandomElement(enemies);
}
function getRandomHero() {
return getRandomElement(heroes);
}
var x, randomEnemy, hero;
var output = document.getElementById("output");
var fightBtn = document.getElementById("fight");
var resetBtn = document.getElementById("reset");
fightBtn.addEventListener("click", battle);
function reset() {
x = 1;
randomEnemy = getRandomEnemy();
fightBtn.disabled = false;
hero = getRandomHero();
output.innerHTML = "";
}
resetBtn.addEventListener("click", reset);
reset();
function battle() {
if (hero.health <= 0 || randomEnemy.health <= 0) {
fightBtn.disabled = true;
return;
}
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero.dodge)];
var heroDamage = Math.floor((Math.random() * hero.damage) + 1);
output.innerHTML += "<br>" + "<b>" + "Round " + x++ + "</b>";
output.innerHTML += "<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon;
if (randomNumber < heroDodge) {
output.innerHTML += "<br>" + "You evade the attack!";
} else if (hero.health > 0) {
hero.health = hero.health - enemyDamage;
if (hero.health < 0)
hero.health = 0;
output.innerHTML += "<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage";
output.innerHTML += "<br>" + "You have " + hero.health + " health remaining.";
}
if (hero.health <= 0) {
output.innerHTML += "<br>" + "You have been killed by the " + randomEnemy.name;
fightBtn.disabled = true;
return;
} else {
output.innerHTML += "<br>" + hero.name + " attacks the " + randomEnemy.name + " with their " + hero.weapon;
}
if (randomNumber < enemyDodge) {
output.innerHTML += "<br>" + "The " + randomEnemy.name + " evades the attack!";
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
if (randomEnemy.health < 0)
randomEnemy.health = 0;
output.innerHTML += "<br>" + hero.name + " did " + heroDamage + " damage";
output.innerHTML += "<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health";
}
if (randomEnemy.health <= 0) {
output.innerHTML += "<br>" + "The " + randomEnemy.name + " is dead!";
fightBtn.disabled = true;
}
}
<h1><b>Halloween RPG Battle</b></h1>
<p><button id="fight">Fight</button><button id="reset">Reset</button></p>
<div id="output"></div>
answered Nov 26 '18 at 16:57
Austin MullinsAustin Mullins
6,18022543
6,18022543
Thanks very much for this. Took me ages to understand and write the original code, now I'm going to have to learn some more :) I've found the hardest thing for me is to get my head round the order code runs and how that relates to how it appears on screen. I'm quite a visual person and being faced by lots of code is hard work!
– user2497517
Nov 27 '18 at 14:29
Could you explain this function getRandomElement(list) ? I see you mention it above but Im at a loss at what it does as I can't see what it reference?
– user2497517
Nov 29 '18 at 15:49
1
Sure. It fetches a random element of the list. Math.random() returns a number between 0 and 1 (exclusive). Multiply that by the number of elements in the list to get something between 0 and that number (exclusive). Math.floor() returns the integer before the number, so you get a valid index
– Austin Mullins
Nov 30 '18 at 19:40
1
Then, I call Object.create() on that element to make a copy of it.
– Austin Mullins
Nov 30 '18 at 19:41
Thanks. I didnt read it carefully enough first time round and failed to realise getRandomElement is called by the other two functions. I've got some time today so hopefully the changes you made will sink in! :) It takes me such a long time to a) Understand concepts and b) remember them a few days later (especially if i have to take a break for a few days). 1 step forwards then 2 back! :(
– user2497517
Dec 2 '18 at 14:29
add a comment |
Thanks very much for this. Took me ages to understand and write the original code, now I'm going to have to learn some more :) I've found the hardest thing for me is to get my head round the order code runs and how that relates to how it appears on screen. I'm quite a visual person and being faced by lots of code is hard work!
– user2497517
Nov 27 '18 at 14:29
Could you explain this function getRandomElement(list) ? I see you mention it above but Im at a loss at what it does as I can't see what it reference?
– user2497517
Nov 29 '18 at 15:49
1
Sure. It fetches a random element of the list. Math.random() returns a number between 0 and 1 (exclusive). Multiply that by the number of elements in the list to get something between 0 and that number (exclusive). Math.floor() returns the integer before the number, so you get a valid index
– Austin Mullins
Nov 30 '18 at 19:40
1
Then, I call Object.create() on that element to make a copy of it.
– Austin Mullins
Nov 30 '18 at 19:41
Thanks. I didnt read it carefully enough first time round and failed to realise getRandomElement is called by the other two functions. I've got some time today so hopefully the changes you made will sink in! :) It takes me such a long time to a) Understand concepts and b) remember them a few days later (especially if i have to take a break for a few days). 1 step forwards then 2 back! :(
– user2497517
Dec 2 '18 at 14:29
Thanks very much for this. Took me ages to understand and write the original code, now I'm going to have to learn some more :) I've found the hardest thing for me is to get my head round the order code runs and how that relates to how it appears on screen. I'm quite a visual person and being faced by lots of code is hard work!
– user2497517
Nov 27 '18 at 14:29
Thanks very much for this. Took me ages to understand and write the original code, now I'm going to have to learn some more :) I've found the hardest thing for me is to get my head round the order code runs and how that relates to how it appears on screen. I'm quite a visual person and being faced by lots of code is hard work!
– user2497517
Nov 27 '18 at 14:29
Could you explain this function getRandomElement(list) ? I see you mention it above but Im at a loss at what it does as I can't see what it reference?
– user2497517
Nov 29 '18 at 15:49
Could you explain this function getRandomElement(list) ? I see you mention it above but Im at a loss at what it does as I can't see what it reference?
– user2497517
Nov 29 '18 at 15:49
1
1
Sure. It fetches a random element of the list. Math.random() returns a number between 0 and 1 (exclusive). Multiply that by the number of elements in the list to get something between 0 and that number (exclusive). Math.floor() returns the integer before the number, so you get a valid index
– Austin Mullins
Nov 30 '18 at 19:40
Sure. It fetches a random element of the list. Math.random() returns a number between 0 and 1 (exclusive). Multiply that by the number of elements in the list to get something between 0 and that number (exclusive). Math.floor() returns the integer before the number, so you get a valid index
– Austin Mullins
Nov 30 '18 at 19:40
1
1
Then, I call Object.create() on that element to make a copy of it.
– Austin Mullins
Nov 30 '18 at 19:41
Then, I call Object.create() on that element to make a copy of it.
– Austin Mullins
Nov 30 '18 at 19:41
Thanks. I didnt read it carefully enough first time round and failed to realise getRandomElement is called by the other two functions. I've got some time today so hopefully the changes you made will sink in! :) It takes me such a long time to a) Understand concepts and b) remember them a few days later (especially if i have to take a break for a few days). 1 step forwards then 2 back! :(
– user2497517
Dec 2 '18 at 14:29
Thanks. I didnt read it carefully enough first time round and failed to realise getRandomElement is called by the other two functions. I've got some time today so hopefully the changes you made will sink in! :) It takes me such a long time to a) Understand concepts and b) remember them a few days later (especially if i have to take a break for a few days). 1 step forwards then 2 back! :(
– user2497517
Dec 2 '18 at 14:29
add a comment |
My main modification is to push the texts to an array and show the array when something happens
NEVER use document.write after load - it will wipe the page and script
Also added a load eventhandler and a reset
function show() {
document.getElementById("action").innerHTML = text.join("");
if (hero[0].health <= 0) {
if (confirm("Reset?")) {
reset();
}
}
}
var enemy,hero;
function reset() {
document.getElementById("action").innerHTML = "Ready for battle!!!";
enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
}
var text = ;
function battle() {
var x = 1;
var randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
text = ; // reset;
text.push("<br>" + "<b>" + "Round " + x++ + "</b>");
text.push("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
text.push("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
text.push("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
text.push("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
text.push("<br>" + "You have been killed by the " + randomEnemy.name);
show();
return
} else {
text.push("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
text.push("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
text.push("<br>" + "Mary did " + heroDamage + " damage");
text.push("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
show();
}
window.addEventListener("load",function() {
reset();
document.getElementById("fight").addEventListener("click", battle);
});
<h1><b>Halloween RPG Battle</b></h1>
<button type="button" id="fight">FIGHT</button>
<div id="action"></div>
add a comment |
My main modification is to push the texts to an array and show the array when something happens
NEVER use document.write after load - it will wipe the page and script
Also added a load eventhandler and a reset
function show() {
document.getElementById("action").innerHTML = text.join("");
if (hero[0].health <= 0) {
if (confirm("Reset?")) {
reset();
}
}
}
var enemy,hero;
function reset() {
document.getElementById("action").innerHTML = "Ready for battle!!!";
enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
}
var text = ;
function battle() {
var x = 1;
var randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
text = ; // reset;
text.push("<br>" + "<b>" + "Round " + x++ + "</b>");
text.push("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
text.push("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
text.push("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
text.push("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
text.push("<br>" + "You have been killed by the " + randomEnemy.name);
show();
return
} else {
text.push("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
text.push("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
text.push("<br>" + "Mary did " + heroDamage + " damage");
text.push("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
show();
}
window.addEventListener("load",function() {
reset();
document.getElementById("fight").addEventListener("click", battle);
});
<h1><b>Halloween RPG Battle</b></h1>
<button type="button" id="fight">FIGHT</button>
<div id="action"></div>
add a comment |
My main modification is to push the texts to an array and show the array when something happens
NEVER use document.write after load - it will wipe the page and script
Also added a load eventhandler and a reset
function show() {
document.getElementById("action").innerHTML = text.join("");
if (hero[0].health <= 0) {
if (confirm("Reset?")) {
reset();
}
}
}
var enemy,hero;
function reset() {
document.getElementById("action").innerHTML = "Ready for battle!!!";
enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
}
var text = ;
function battle() {
var x = 1;
var randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
text = ; // reset;
text.push("<br>" + "<b>" + "Round " + x++ + "</b>");
text.push("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
text.push("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
text.push("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
text.push("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
text.push("<br>" + "You have been killed by the " + randomEnemy.name);
show();
return
} else {
text.push("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
text.push("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
text.push("<br>" + "Mary did " + heroDamage + " damage");
text.push("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
show();
}
window.addEventListener("load",function() {
reset();
document.getElementById("fight").addEventListener("click", battle);
});
<h1><b>Halloween RPG Battle</b></h1>
<button type="button" id="fight">FIGHT</button>
<div id="action"></div>
My main modification is to push the texts to an array and show the array when something happens
NEVER use document.write after load - it will wipe the page and script
Also added a load eventhandler and a reset
function show() {
document.getElementById("action").innerHTML = text.join("");
if (hero[0].health <= 0) {
if (confirm("Reset?")) {
reset();
}
}
}
var enemy,hero;
function reset() {
document.getElementById("action").innerHTML = "Ready for battle!!!";
enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
}
var text = ;
function battle() {
var x = 1;
var randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
text = ; // reset;
text.push("<br>" + "<b>" + "Round " + x++ + "</b>");
text.push("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
text.push("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
text.push("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
text.push("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
text.push("<br>" + "You have been killed by the " + randomEnemy.name);
show();
return
} else {
text.push("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
text.push("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
text.push("<br>" + "Mary did " + heroDamage + " damage");
text.push("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
show();
}
window.addEventListener("load",function() {
reset();
document.getElementById("fight").addEventListener("click", battle);
});
<h1><b>Halloween RPG Battle</b></h1>
<button type="button" id="fight">FIGHT</button>
<div id="action"></div>
function show() {
document.getElementById("action").innerHTML = text.join("");
if (hero[0].health <= 0) {
if (confirm("Reset?")) {
reset();
}
}
}
var enemy,hero;
function reset() {
document.getElementById("action").innerHTML = "Ready for battle!!!";
enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
}
var text = ;
function battle() {
var x = 1;
var randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
text = ; // reset;
text.push("<br>" + "<b>" + "Round " + x++ + "</b>");
text.push("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
text.push("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
text.push("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
text.push("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
text.push("<br>" + "You have been killed by the " + randomEnemy.name);
show();
return
} else {
text.push("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
text.push("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
text.push("<br>" + "Mary did " + heroDamage + " damage");
text.push("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
show();
}
window.addEventListener("load",function() {
reset();
document.getElementById("fight").addEventListener("click", battle);
});
<h1><b>Halloween RPG Battle</b></h1>
<button type="button" id="fight">FIGHT</button>
<div id="action"></div>
function show() {
document.getElementById("action").innerHTML = text.join("");
if (hero[0].health <= 0) {
if (confirm("Reset?")) {
reset();
}
}
}
var enemy,hero;
function reset() {
document.getElementById("action").innerHTML = "Ready for battle!!!";
enemy = [{
name: 'Wizard',
health: 10,
weapon: 'his staff.',
damage: 12,
dodge: 10,
backpack: 'Cloak of Invisibility.'
},
{
name: 'Elf',
health: 4,
weapon: 'a dagger.',
damage: 6,
dodge: 8,
backpack: 'Bow & Arrow.'
},
{
name: 'Dragon',
health: 20,
weapon: 'a fireball.',
damage: 15,
dodge: 2,
backpack: ''
},
{
name: 'Goblin',
health: 12,
weapon: 'his bow and arrow.',
damage: 4,
dodge: 6,
backpack: 'gold coins.'
},
{
name: 'Dwarf',
health: 7,
weapon: 'his axe.',
damage: 5,
dodge: 4,
backpack: 'map'
},
{
name: 'Orc',
health: 8,
weapon: 'a sword.',
damage: 5,
dodge: 5,
backpack: 'silver tooth.'
},
{
name: 'Witch',
health: 6,
weapon: 'her potion of the undead.',
damage: 7,
dodge: 6,
backpack: 'potion of the living.'
},
{
name: 'Old Lady',
health: 3,
weapon: 'her frying pan.',
damage: 1,
dodge: 1,
backpack: 'fruit and vegetables.'
},
{
name: 'Villagers',
health: 15,
weapon: 'sharpened sticks.',
damage: 5,
dodge: 1,
backpack: 'meat.'
},
{
name: 'Thief',
health: 4,
weapon: 'his fists.',
damage: 3,
dodge: 9,
backpack: 'jewels.'
}
];
hero = [{
name: 'Mary',
health: 15,
weapon: 'sword',
damage: 6,
dodge: 8,
backpack: ''
}];
}
var text = ;
function battle() {
var x = 1;
var randomEnemy = enemy[Math.floor(Math.random() * enemy.length)];
var enemyDamage = Math.floor((Math.random() * (randomEnemy.damage)) + 1);
var enemyDodge = Math.floor((Math.random() * (randomEnemy.dodge)) + 1);
var randomNumber = Math.floor(Math.random() * 4) + 1;
var heroDodge = [Math.floor(Math.random() * hero[0].dodge)];
var heroDamage = Math.floor((Math.random() * hero[0].damage) + 1);
text = ; // reset;
text.push("<br>" + "<b>" + "Round " + x++ + "</b>");
text.push("<br>" + "The " + randomEnemy.name + " attacks you with " + randomEnemy.weapon);
if (randomNumber < heroDodge) {
text.push("<br>" + "You evade the attack!");
} else if (hero[0].health > 0) {
hero[0].health = hero[0].health - enemyDamage;
text.push("<br>" + "The " + randomEnemy.name + " did " + enemyDamage + " damage");
text.push("<br>" + "You have " + hero[0].health + " health remaining.");
}
if (hero[0].health <= 0) {
text.push("<br>" + "You have been killed by the " + randomEnemy.name);
show();
return
} else {
text.push("<br>" + "Mary attacks the " + randomEnemy.name + " with her sword.");
}
if (randomNumber < enemyDodge) {
text.push("<br>" + "The " + randomEnemy.name + " evades the attack!");
} else if (randomEnemy.health > 0) {
randomEnemy.health = randomEnemy.health - heroDamage;
text.push("<br>" + "Mary did " + heroDamage + " damage");
text.push("<br>" + "The " + randomEnemy.name + " has " + randomEnemy.health + " health");
}
show();
}
window.addEventListener("load",function() {
reset();
document.getElementById("fight").addEventListener("click", battle);
});
<h1><b>Halloween RPG Battle</b></h1>
<button type="button" id="fight">FIGHT</button>
<div id="action"></div>
edited Nov 27 '18 at 8:31
answered Nov 26 '18 at 16:11
mplungjanmplungjan
88.4k21125182
88.4k21125182
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53484707%2fallow-button-click-whilst-do-loop-is-true%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vglguTt7aw95
1
1: the title needs to be in title tags when in the head, otherwise a H1 belongs in the body 2: Where is the fight button in the HTML? Please click edit and fix the snippet I made to reflect your html. 3: NEVER document.write after the page has loaded . I could not put your script in the pane for script for the same reason. Use innerHTML instead
– mplungjan
Nov 26 '18 at 15:53
2
Remove the while-loop from the battle(), then add a check at the end of the battle() that checks if there's enough health left for the fight. That way you have to click 'battle' for each round of fighting and you can redirect to a different function once the battle is won by someone.
– Shilly
Nov 26 '18 at 15:54
1
try
confirm("Press a button!");
to fight– javimovi
Nov 26 '18 at 16:00
mplungjan = Sorry, im about as new to Stack Exchange as coding :) I meant to take all those button code out as it wasnt working, but I obviously left a bit in. confused.com. Thanks for your observation on document.write - Im just going with what works (you should see it before it got to this point!) Shilly - thanks Ill try that first when I have a moment......
– user2497517
Nov 26 '18 at 16:01