NodeJs - fetch request from client always returns a 200 status response from the server regardless if there...
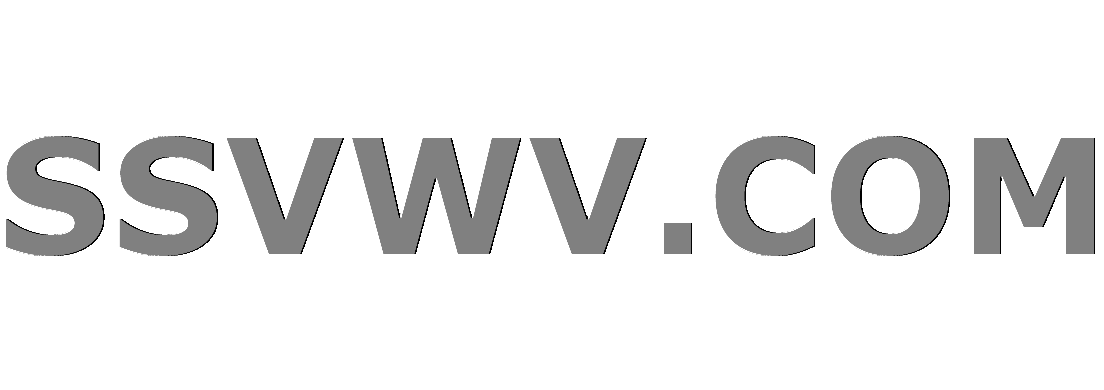
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am using a fetch()
request from the client to PUT updated user data to the backend, to then be saved into a DB. So far, the all of the route is working fine, verified and tested in Postman.
In this User Update
route, there is an if
statement that checks for an error when searching for the user in the database, and if this error is thrown, it sends a response of 404 and a message to the client.
When I make the fetch()
request from the client to this route, regardless if there is an error, the response is always a status 200, and does not include any response from my route. I need the client to be able to handle the potential errors the routes might produce. For example with this user update route, if the user is not found in the database for whatever reason, an error and message is returned, so the client needs to know this.
Here is some code:
Client-side:
fetch(`http://localhost:3000/users/${userId}`, {
method: "put",
headers: {
Accept: "application/json",
"Content-Type": "application/json"
},
body: JSON.stringify(userData)
}).then(response => console.log(response))
.catch(err => console.log(err));
Here in the client, I am using console.log()
to visualize everything. When the response returns, I get back:
Response {type: "basic", url: "http://localhost:3000/users/accounts/", redirected: false, status: 200, ok: true, …}
Server-side route controller:
exports.user_update = (req, res) => {
const { params, body } = req;
const { userid } = params;
User.findByIdAndUpdate({ _id: userid }, body, { new: true }, (err, user) => {
if (err)
res.send({
status: 404,
message:
"There was an issue finding and updating the user on the server."
});
else
res.send({
status: 200,
user
});
});
};
Now here on the server, I anticipated the response of the fetch()
to be either the 404 error or the 200 success along with their payloads. Neither are returned in a response from the server. Instead as mentioned above, I am getting a generic 200 ok
response simply letting me know the fetch()
made a connection to the route. This route(along with the others) has been tested in Postman, and all work as anticipated returning the expected responses.
What am I not understanding here? Is my idea of using a fetch()
request in this manner wrong? I feel like I might be close, but that's just my ignorant guess. Thank for reading!
javascript node.js reactjs fetch
add a comment |
I am using a fetch()
request from the client to PUT updated user data to the backend, to then be saved into a DB. So far, the all of the route is working fine, verified and tested in Postman.
In this User Update
route, there is an if
statement that checks for an error when searching for the user in the database, and if this error is thrown, it sends a response of 404 and a message to the client.
When I make the fetch()
request from the client to this route, regardless if there is an error, the response is always a status 200, and does not include any response from my route. I need the client to be able to handle the potential errors the routes might produce. For example with this user update route, if the user is not found in the database for whatever reason, an error and message is returned, so the client needs to know this.
Here is some code:
Client-side:
fetch(`http://localhost:3000/users/${userId}`, {
method: "put",
headers: {
Accept: "application/json",
"Content-Type": "application/json"
},
body: JSON.stringify(userData)
}).then(response => console.log(response))
.catch(err => console.log(err));
Here in the client, I am using console.log()
to visualize everything. When the response returns, I get back:
Response {type: "basic", url: "http://localhost:3000/users/accounts/", redirected: false, status: 200, ok: true, …}
Server-side route controller:
exports.user_update = (req, res) => {
const { params, body } = req;
const { userid } = params;
User.findByIdAndUpdate({ _id: userid }, body, { new: true }, (err, user) => {
if (err)
res.send({
status: 404,
message:
"There was an issue finding and updating the user on the server."
});
else
res.send({
status: 200,
user
});
});
};
Now here on the server, I anticipated the response of the fetch()
to be either the 404 error or the 200 success along with their payloads. Neither are returned in a response from the server. Instead as mentioned above, I am getting a generic 200 ok
response simply letting me know the fetch()
made a connection to the route. This route(along with the others) has been tested in Postman, and all work as anticipated returning the expected responses.
What am I not understanding here? Is my idea of using a fetch()
request in this manner wrong? I feel like I might be close, but that's just my ignorant guess. Thank for reading!
javascript node.js reactjs fetch
add a comment |
I am using a fetch()
request from the client to PUT updated user data to the backend, to then be saved into a DB. So far, the all of the route is working fine, verified and tested in Postman.
In this User Update
route, there is an if
statement that checks for an error when searching for the user in the database, and if this error is thrown, it sends a response of 404 and a message to the client.
When I make the fetch()
request from the client to this route, regardless if there is an error, the response is always a status 200, and does not include any response from my route. I need the client to be able to handle the potential errors the routes might produce. For example with this user update route, if the user is not found in the database for whatever reason, an error and message is returned, so the client needs to know this.
Here is some code:
Client-side:
fetch(`http://localhost:3000/users/${userId}`, {
method: "put",
headers: {
Accept: "application/json",
"Content-Type": "application/json"
},
body: JSON.stringify(userData)
}).then(response => console.log(response))
.catch(err => console.log(err));
Here in the client, I am using console.log()
to visualize everything. When the response returns, I get back:
Response {type: "basic", url: "http://localhost:3000/users/accounts/", redirected: false, status: 200, ok: true, …}
Server-side route controller:
exports.user_update = (req, res) => {
const { params, body } = req;
const { userid } = params;
User.findByIdAndUpdate({ _id: userid }, body, { new: true }, (err, user) => {
if (err)
res.send({
status: 404,
message:
"There was an issue finding and updating the user on the server."
});
else
res.send({
status: 200,
user
});
});
};
Now here on the server, I anticipated the response of the fetch()
to be either the 404 error or the 200 success along with their payloads. Neither are returned in a response from the server. Instead as mentioned above, I am getting a generic 200 ok
response simply letting me know the fetch()
made a connection to the route. This route(along with the others) has been tested in Postman, and all work as anticipated returning the expected responses.
What am I not understanding here? Is my idea of using a fetch()
request in this manner wrong? I feel like I might be close, but that's just my ignorant guess. Thank for reading!
javascript node.js reactjs fetch
I am using a fetch()
request from the client to PUT updated user data to the backend, to then be saved into a DB. So far, the all of the route is working fine, verified and tested in Postman.
In this User Update
route, there is an if
statement that checks for an error when searching for the user in the database, and if this error is thrown, it sends a response of 404 and a message to the client.
When I make the fetch()
request from the client to this route, regardless if there is an error, the response is always a status 200, and does not include any response from my route. I need the client to be able to handle the potential errors the routes might produce. For example with this user update route, if the user is not found in the database for whatever reason, an error and message is returned, so the client needs to know this.
Here is some code:
Client-side:
fetch(`http://localhost:3000/users/${userId}`, {
method: "put",
headers: {
Accept: "application/json",
"Content-Type": "application/json"
},
body: JSON.stringify(userData)
}).then(response => console.log(response))
.catch(err => console.log(err));
Here in the client, I am using console.log()
to visualize everything. When the response returns, I get back:
Response {type: "basic", url: "http://localhost:3000/users/accounts/", redirected: false, status: 200, ok: true, …}
Server-side route controller:
exports.user_update = (req, res) => {
const { params, body } = req;
const { userid } = params;
User.findByIdAndUpdate({ _id: userid }, body, { new: true }, (err, user) => {
if (err)
res.send({
status: 404,
message:
"There was an issue finding and updating the user on the server."
});
else
res.send({
status: 200,
user
});
});
};
Now here on the server, I anticipated the response of the fetch()
to be either the 404 error or the 200 success along with their payloads. Neither are returned in a response from the server. Instead as mentioned above, I am getting a generic 200 ok
response simply letting me know the fetch()
made a connection to the route. This route(along with the others) has been tested in Postman, and all work as anticipated returning the expected responses.
What am I not understanding here? Is my idea of using a fetch()
request in this manner wrong? I feel like I might be close, but that's just my ignorant guess. Thank for reading!
javascript node.js reactjs fetch
javascript node.js reactjs fetch
asked Nov 29 '18 at 2:35
maison.mmaison.m
11610
11610
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
According to express documentation you sent status as a field of JSON. To correctly send http status replace your res.send(...)
with
res.status(404).send("There was an issue finding and updating the user on the server.");
and
res.send(user);
I tried to do this, and I am still not getting the response from the server. I am only getting back the generic response of status 200.
– maison.m
Nov 30 '18 at 2:53
Could you check yourUser.findByIdAndUpdate(..)
, or, at least, log theerr
anduser
values after the call
– Vasyl Moskalov
Nov 30 '18 at 3:30
add a comment |
You are sending the status
field inside your payload, which is not parsed by fetch
API.
To solve this you could something as below
exports.user_update = (req, res) => {
const { params, body } = req;
const { userid } = params;
User.findByIdAndUpdate({ _id: userid }, body, { new: true }, (err, user) =>
{
if (err)
res.status(404).send({
message: "There was an issue finding and updating the user on the server."
});
else
res.status(200).send({
user
});
});
};
add a comment |
I figured out the solution.
The fetch request was wrong, here is the update:
fetch(`http://localhost:3000/users/${userId}`, {
method: "put",
headers: {
Accept: "application/json",
"Content-Type": "application/json"
},
body: JSON.stringify(userData)
}).then(response => response.json())
.then(response => console.log(response))
.catch(err => console.log(err));
I had to call response.json()
to parse the response as a JSON object.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53531014%2fnodejs-fetch-request-from-client-always-returns-a-200-status-response-from-the%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
According to express documentation you sent status as a field of JSON. To correctly send http status replace your res.send(...)
with
res.status(404).send("There was an issue finding and updating the user on the server.");
and
res.send(user);
I tried to do this, and I am still not getting the response from the server. I am only getting back the generic response of status 200.
– maison.m
Nov 30 '18 at 2:53
Could you check yourUser.findByIdAndUpdate(..)
, or, at least, log theerr
anduser
values after the call
– Vasyl Moskalov
Nov 30 '18 at 3:30
add a comment |
According to express documentation you sent status as a field of JSON. To correctly send http status replace your res.send(...)
with
res.status(404).send("There was an issue finding and updating the user on the server.");
and
res.send(user);
I tried to do this, and I am still not getting the response from the server. I am only getting back the generic response of status 200.
– maison.m
Nov 30 '18 at 2:53
Could you check yourUser.findByIdAndUpdate(..)
, or, at least, log theerr
anduser
values after the call
– Vasyl Moskalov
Nov 30 '18 at 3:30
add a comment |
According to express documentation you sent status as a field of JSON. To correctly send http status replace your res.send(...)
with
res.status(404).send("There was an issue finding and updating the user on the server.");
and
res.send(user);
According to express documentation you sent status as a field of JSON. To correctly send http status replace your res.send(...)
with
res.status(404).send("There was an issue finding and updating the user on the server.");
and
res.send(user);
answered Nov 29 '18 at 2:47


Vasyl MoskalovVasyl Moskalov
2,1181219
2,1181219
I tried to do this, and I am still not getting the response from the server. I am only getting back the generic response of status 200.
– maison.m
Nov 30 '18 at 2:53
Could you check yourUser.findByIdAndUpdate(..)
, or, at least, log theerr
anduser
values after the call
– Vasyl Moskalov
Nov 30 '18 at 3:30
add a comment |
I tried to do this, and I am still not getting the response from the server. I am only getting back the generic response of status 200.
– maison.m
Nov 30 '18 at 2:53
Could you check yourUser.findByIdAndUpdate(..)
, or, at least, log theerr
anduser
values after the call
– Vasyl Moskalov
Nov 30 '18 at 3:30
I tried to do this, and I am still not getting the response from the server. I am only getting back the generic response of status 200.
– maison.m
Nov 30 '18 at 2:53
I tried to do this, and I am still not getting the response from the server. I am only getting back the generic response of status 200.
– maison.m
Nov 30 '18 at 2:53
Could you check your
User.findByIdAndUpdate(..)
, or, at least, log the err
and user
values after the call– Vasyl Moskalov
Nov 30 '18 at 3:30
Could you check your
User.findByIdAndUpdate(..)
, or, at least, log the err
and user
values after the call– Vasyl Moskalov
Nov 30 '18 at 3:30
add a comment |
You are sending the status
field inside your payload, which is not parsed by fetch
API.
To solve this you could something as below
exports.user_update = (req, res) => {
const { params, body } = req;
const { userid } = params;
User.findByIdAndUpdate({ _id: userid }, body, { new: true }, (err, user) =>
{
if (err)
res.status(404).send({
message: "There was an issue finding and updating the user on the server."
});
else
res.status(200).send({
user
});
});
};
add a comment |
You are sending the status
field inside your payload, which is not parsed by fetch
API.
To solve this you could something as below
exports.user_update = (req, res) => {
const { params, body } = req;
const { userid } = params;
User.findByIdAndUpdate({ _id: userid }, body, { new: true }, (err, user) =>
{
if (err)
res.status(404).send({
message: "There was an issue finding and updating the user on the server."
});
else
res.status(200).send({
user
});
});
};
add a comment |
You are sending the status
field inside your payload, which is not parsed by fetch
API.
To solve this you could something as below
exports.user_update = (req, res) => {
const { params, body } = req;
const { userid } = params;
User.findByIdAndUpdate({ _id: userid }, body, { new: true }, (err, user) =>
{
if (err)
res.status(404).send({
message: "There was an issue finding and updating the user on the server."
});
else
res.status(200).send({
user
});
});
};
You are sending the status
field inside your payload, which is not parsed by fetch
API.
To solve this you could something as below
exports.user_update = (req, res) => {
const { params, body } = req;
const { userid } = params;
User.findByIdAndUpdate({ _id: userid }, body, { new: true }, (err, user) =>
{
if (err)
res.status(404).send({
message: "There was an issue finding and updating the user on the server."
});
else
res.status(200).send({
user
});
});
};
answered Nov 29 '18 at 4:35


Revanth MRevanth M
1296
1296
add a comment |
add a comment |
I figured out the solution.
The fetch request was wrong, here is the update:
fetch(`http://localhost:3000/users/${userId}`, {
method: "put",
headers: {
Accept: "application/json",
"Content-Type": "application/json"
},
body: JSON.stringify(userData)
}).then(response => response.json())
.then(response => console.log(response))
.catch(err => console.log(err));
I had to call response.json()
to parse the response as a JSON object.
add a comment |
I figured out the solution.
The fetch request was wrong, here is the update:
fetch(`http://localhost:3000/users/${userId}`, {
method: "put",
headers: {
Accept: "application/json",
"Content-Type": "application/json"
},
body: JSON.stringify(userData)
}).then(response => response.json())
.then(response => console.log(response))
.catch(err => console.log(err));
I had to call response.json()
to parse the response as a JSON object.
add a comment |
I figured out the solution.
The fetch request was wrong, here is the update:
fetch(`http://localhost:3000/users/${userId}`, {
method: "put",
headers: {
Accept: "application/json",
"Content-Type": "application/json"
},
body: JSON.stringify(userData)
}).then(response => response.json())
.then(response => console.log(response))
.catch(err => console.log(err));
I had to call response.json()
to parse the response as a JSON object.
I figured out the solution.
The fetch request was wrong, here is the update:
fetch(`http://localhost:3000/users/${userId}`, {
method: "put",
headers: {
Accept: "application/json",
"Content-Type": "application/json"
},
body: JSON.stringify(userData)
}).then(response => response.json())
.then(response => console.log(response))
.catch(err => console.log(err));
I had to call response.json()
to parse the response as a JSON object.
answered Nov 30 '18 at 6:01
maison.mmaison.m
11610
11610
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53531014%2fnodejs-fetch-request-from-client-always-returns-a-200-status-response-from-the%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JEW9Ex8K7AQAJor3n3H9P