Insertion sort algorithm using doubly-linked lists in c - inserting nodes
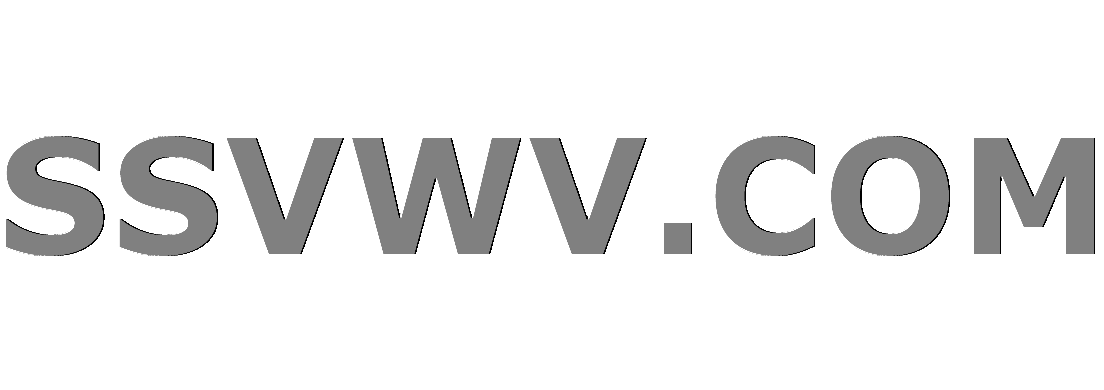
Multi tool use
I am very new to coding and I'm trying to write a function in C using the insertion sort algorithm on a doubly linked list. The function takes an element off the old list and inserts it in alphabetical order on a new list.
I'm having problems looping the function when an element is greater than (ie further along in the alphabet) the first element in the new/sorted list. Right now it is only adding elements that can be appended or prepended. Why is this?
Here is my function:
#include "sort.h"
#include <stdio.h>
#include <stdlib.h>
#include "linked_list.h"
/* sort linked list *list using merge insertion sort. */
/* upon success, the elements in *list will be sorted. */
/* return silently if *list is uninitialised or empty. */
/* the compare argument is a pointer to a function which returns */
/* less than 0, 0, or greater than 0 if first argument is */
/* less than, equal to, or greater than second argument respectively. */
void insertion_sort(LinkedList *list, int (*compare)(void *, void *))
{
void *d, *B_node_data;
LinkedList *B;
Node *B_node, *new_node;
if(!list)
return;
if(!list->head)
return;
B = initialise_linked_list();
d = list->head->data;
remove_head_linked_list(list);
prepend_linked_list(B, d);
while(list->head)
{
d = list->head->data;
B_node = B->head;
remove_head_linked_list(list);
while(B_node)
{
B_node_data = B_node->data;
if((*compare)(d, B_node_data) <= 0)
{
if(B_node->prev)
{
new_node = initialise_node();
new_node->next = B_node;
new_node->prev = B_node->prev;
new_node->data = d;
break;
}
if(B->head->data == B_node_data)
{
prepend_linked_list(B, d);
break;
}
}
if(!B_node->next)
append_linked_list(B, d);
else{
B_node = B_node->next;
}
}
}
list->head = B->head;
list->tail = B->tail;
B->head = NULL;
B->tail = NULL;
free_linked_list(B);
}
here is the definitions of the functions i called from another file:
#include "linked_list.h"
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
Node *initialise_node(void)
{
Node *node;
if(!(node = (Node *) malloc(sizeof(Node)))) {
fprintf(stderr, "error: unable to initialise node.n");
exit(EXIT_FAILURE);
}
node->next = node->prev = node->data = NULL;
return node;
}
void free_node(Node *node)
{
if(!node)
return;
free(node);
}
LinkedList *initialise_linked_list(void)
{
LinkedList *list;
if(!(list = (LinkedList *) malloc(sizeof(LinkedList)))) {
fprintf(stderr, "error: unable to initialise linked list.n");
exit(EXIT_FAILURE);
}
list->head = list->tail = NULL;
return list;
}
void free_linked_list(LinkedList *list)
{
Node *next;
while(list->head) {
next = list->head->next;
free_node(list->head);
list->head = next;
}
free(list);
}
void append_linked_list(LinkedList *list, void *data)
{
Node *node;
node = initialise_node();
node->data = data;
node->prev = list->tail;
if(list->tail) {
list->tail->next = node;
}
list->tail = node;
if(!list->head)
list->head = node;
}
void prepend_linked_list(LinkedList *list, void *data)
{
Node *node;
node = initialise_node();
node->data = data;
node->next = list->head;
if(list->head) {
list->head->prev = node;
}
list->head = node;
if(!list->tail)
list->tail = node;
}
void remove_head_linked_list(LinkedList *list)
{
Node *head;
if(!list->head)
return;
head = list->head->next;
free(list->head);
list->head = head;
if(list->head)
list->head->prev = NULL;
else
list->tail = NULL;
}
void remove_tail_linked_list(LinkedList *list)
{
Node *tail;
if(!list->tail)
return;
tail = list->tail->prev;
free_node(list->tail);
list->tail = tail;
if(list->tail)
list->tail->next = NULL;
else
list->head = NULL;
}
here is my output
==942== Command: ./task1_test harmonious pencil push naive jittery
greedy billowy practise lock star appear record precede pen lace
==942==
appear
billowy
greedy
harmonious
pencil
push
star
==942==
==942== HEAP SUMMARY:
==942== in use at exit: 264 bytes in 11 blocks
==942== total heap usage: 35 allocs, 24 frees, 824 bytes allocated
==942==
==942== 264 bytes in 11 blocks are definitely lost in loss record 1 of 1
==942== at 0x4C29C23: malloc (vg_replace_malloc.c:299)
==942== by 0x40084E: initialise_node (linked_list.c:11)
==942== by 0x400CF1: insertion_sort (sort.c:43)
==942== by 0x400815: main (task1_test.c:33)
==942==
==942== LEAK SUMMARY:
==942== definitely lost: 264 bytes in 11 blocks
==942== indirectly lost: 0 bytes in 0 blocks
==942== possibly lost: 0 bytes in 0 blocks
==942== still reachable: 0 bytes in 0 blocks
==942== suppressed: 0 bytes in 0 blocks
==942==
c linked-list doubly-linked-list insertion-sort
add a comment |
I am very new to coding and I'm trying to write a function in C using the insertion sort algorithm on a doubly linked list. The function takes an element off the old list and inserts it in alphabetical order on a new list.
I'm having problems looping the function when an element is greater than (ie further along in the alphabet) the first element in the new/sorted list. Right now it is only adding elements that can be appended or prepended. Why is this?
Here is my function:
#include "sort.h"
#include <stdio.h>
#include <stdlib.h>
#include "linked_list.h"
/* sort linked list *list using merge insertion sort. */
/* upon success, the elements in *list will be sorted. */
/* return silently if *list is uninitialised or empty. */
/* the compare argument is a pointer to a function which returns */
/* less than 0, 0, or greater than 0 if first argument is */
/* less than, equal to, or greater than second argument respectively. */
void insertion_sort(LinkedList *list, int (*compare)(void *, void *))
{
void *d, *B_node_data;
LinkedList *B;
Node *B_node, *new_node;
if(!list)
return;
if(!list->head)
return;
B = initialise_linked_list();
d = list->head->data;
remove_head_linked_list(list);
prepend_linked_list(B, d);
while(list->head)
{
d = list->head->data;
B_node = B->head;
remove_head_linked_list(list);
while(B_node)
{
B_node_data = B_node->data;
if((*compare)(d, B_node_data) <= 0)
{
if(B_node->prev)
{
new_node = initialise_node();
new_node->next = B_node;
new_node->prev = B_node->prev;
new_node->data = d;
break;
}
if(B->head->data == B_node_data)
{
prepend_linked_list(B, d);
break;
}
}
if(!B_node->next)
append_linked_list(B, d);
else{
B_node = B_node->next;
}
}
}
list->head = B->head;
list->tail = B->tail;
B->head = NULL;
B->tail = NULL;
free_linked_list(B);
}
here is the definitions of the functions i called from another file:
#include "linked_list.h"
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
Node *initialise_node(void)
{
Node *node;
if(!(node = (Node *) malloc(sizeof(Node)))) {
fprintf(stderr, "error: unable to initialise node.n");
exit(EXIT_FAILURE);
}
node->next = node->prev = node->data = NULL;
return node;
}
void free_node(Node *node)
{
if(!node)
return;
free(node);
}
LinkedList *initialise_linked_list(void)
{
LinkedList *list;
if(!(list = (LinkedList *) malloc(sizeof(LinkedList)))) {
fprintf(stderr, "error: unable to initialise linked list.n");
exit(EXIT_FAILURE);
}
list->head = list->tail = NULL;
return list;
}
void free_linked_list(LinkedList *list)
{
Node *next;
while(list->head) {
next = list->head->next;
free_node(list->head);
list->head = next;
}
free(list);
}
void append_linked_list(LinkedList *list, void *data)
{
Node *node;
node = initialise_node();
node->data = data;
node->prev = list->tail;
if(list->tail) {
list->tail->next = node;
}
list->tail = node;
if(!list->head)
list->head = node;
}
void prepend_linked_list(LinkedList *list, void *data)
{
Node *node;
node = initialise_node();
node->data = data;
node->next = list->head;
if(list->head) {
list->head->prev = node;
}
list->head = node;
if(!list->tail)
list->tail = node;
}
void remove_head_linked_list(LinkedList *list)
{
Node *head;
if(!list->head)
return;
head = list->head->next;
free(list->head);
list->head = head;
if(list->head)
list->head->prev = NULL;
else
list->tail = NULL;
}
void remove_tail_linked_list(LinkedList *list)
{
Node *tail;
if(!list->tail)
return;
tail = list->tail->prev;
free_node(list->tail);
list->tail = tail;
if(list->tail)
list->tail->next = NULL;
else
list->head = NULL;
}
here is my output
==942== Command: ./task1_test harmonious pencil push naive jittery
greedy billowy practise lock star appear record precede pen lace
==942==
appear
billowy
greedy
harmonious
pencil
push
star
==942==
==942== HEAP SUMMARY:
==942== in use at exit: 264 bytes in 11 blocks
==942== total heap usage: 35 allocs, 24 frees, 824 bytes allocated
==942==
==942== 264 bytes in 11 blocks are definitely lost in loss record 1 of 1
==942== at 0x4C29C23: malloc (vg_replace_malloc.c:299)
==942== by 0x40084E: initialise_node (linked_list.c:11)
==942== by 0x400CF1: insertion_sort (sort.c:43)
==942== by 0x400815: main (task1_test.c:33)
==942==
==942== LEAK SUMMARY:
==942== definitely lost: 264 bytes in 11 blocks
==942== indirectly lost: 0 bytes in 0 blocks
==942== possibly lost: 0 bytes in 0 blocks
==942== still reachable: 0 bytes in 0 blocks
==942== suppressed: 0 bytes in 0 blocks
==942==
c linked-list doubly-linked-list insertion-sort
4
We need to see all the functions related to list insertion/deletion. What you've shown doesn't illustrate the problem you're having. Please edit your question and post your entire program [in a single code block]. It should compile cleanly and we should be able to download it, compile it, and run it [possibly undergdb
] if we so choose. See: stackoverflow.com/help/mcve
– Craig Estey
Nov 28 '18 at 19:02
add a comment |
I am very new to coding and I'm trying to write a function in C using the insertion sort algorithm on a doubly linked list. The function takes an element off the old list and inserts it in alphabetical order on a new list.
I'm having problems looping the function when an element is greater than (ie further along in the alphabet) the first element in the new/sorted list. Right now it is only adding elements that can be appended or prepended. Why is this?
Here is my function:
#include "sort.h"
#include <stdio.h>
#include <stdlib.h>
#include "linked_list.h"
/* sort linked list *list using merge insertion sort. */
/* upon success, the elements in *list will be sorted. */
/* return silently if *list is uninitialised or empty. */
/* the compare argument is a pointer to a function which returns */
/* less than 0, 0, or greater than 0 if first argument is */
/* less than, equal to, or greater than second argument respectively. */
void insertion_sort(LinkedList *list, int (*compare)(void *, void *))
{
void *d, *B_node_data;
LinkedList *B;
Node *B_node, *new_node;
if(!list)
return;
if(!list->head)
return;
B = initialise_linked_list();
d = list->head->data;
remove_head_linked_list(list);
prepend_linked_list(B, d);
while(list->head)
{
d = list->head->data;
B_node = B->head;
remove_head_linked_list(list);
while(B_node)
{
B_node_data = B_node->data;
if((*compare)(d, B_node_data) <= 0)
{
if(B_node->prev)
{
new_node = initialise_node();
new_node->next = B_node;
new_node->prev = B_node->prev;
new_node->data = d;
break;
}
if(B->head->data == B_node_data)
{
prepend_linked_list(B, d);
break;
}
}
if(!B_node->next)
append_linked_list(B, d);
else{
B_node = B_node->next;
}
}
}
list->head = B->head;
list->tail = B->tail;
B->head = NULL;
B->tail = NULL;
free_linked_list(B);
}
here is the definitions of the functions i called from another file:
#include "linked_list.h"
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
Node *initialise_node(void)
{
Node *node;
if(!(node = (Node *) malloc(sizeof(Node)))) {
fprintf(stderr, "error: unable to initialise node.n");
exit(EXIT_FAILURE);
}
node->next = node->prev = node->data = NULL;
return node;
}
void free_node(Node *node)
{
if(!node)
return;
free(node);
}
LinkedList *initialise_linked_list(void)
{
LinkedList *list;
if(!(list = (LinkedList *) malloc(sizeof(LinkedList)))) {
fprintf(stderr, "error: unable to initialise linked list.n");
exit(EXIT_FAILURE);
}
list->head = list->tail = NULL;
return list;
}
void free_linked_list(LinkedList *list)
{
Node *next;
while(list->head) {
next = list->head->next;
free_node(list->head);
list->head = next;
}
free(list);
}
void append_linked_list(LinkedList *list, void *data)
{
Node *node;
node = initialise_node();
node->data = data;
node->prev = list->tail;
if(list->tail) {
list->tail->next = node;
}
list->tail = node;
if(!list->head)
list->head = node;
}
void prepend_linked_list(LinkedList *list, void *data)
{
Node *node;
node = initialise_node();
node->data = data;
node->next = list->head;
if(list->head) {
list->head->prev = node;
}
list->head = node;
if(!list->tail)
list->tail = node;
}
void remove_head_linked_list(LinkedList *list)
{
Node *head;
if(!list->head)
return;
head = list->head->next;
free(list->head);
list->head = head;
if(list->head)
list->head->prev = NULL;
else
list->tail = NULL;
}
void remove_tail_linked_list(LinkedList *list)
{
Node *tail;
if(!list->tail)
return;
tail = list->tail->prev;
free_node(list->tail);
list->tail = tail;
if(list->tail)
list->tail->next = NULL;
else
list->head = NULL;
}
here is my output
==942== Command: ./task1_test harmonious pencil push naive jittery
greedy billowy practise lock star appear record precede pen lace
==942==
appear
billowy
greedy
harmonious
pencil
push
star
==942==
==942== HEAP SUMMARY:
==942== in use at exit: 264 bytes in 11 blocks
==942== total heap usage: 35 allocs, 24 frees, 824 bytes allocated
==942==
==942== 264 bytes in 11 blocks are definitely lost in loss record 1 of 1
==942== at 0x4C29C23: malloc (vg_replace_malloc.c:299)
==942== by 0x40084E: initialise_node (linked_list.c:11)
==942== by 0x400CF1: insertion_sort (sort.c:43)
==942== by 0x400815: main (task1_test.c:33)
==942==
==942== LEAK SUMMARY:
==942== definitely lost: 264 bytes in 11 blocks
==942== indirectly lost: 0 bytes in 0 blocks
==942== possibly lost: 0 bytes in 0 blocks
==942== still reachable: 0 bytes in 0 blocks
==942== suppressed: 0 bytes in 0 blocks
==942==
c linked-list doubly-linked-list insertion-sort
I am very new to coding and I'm trying to write a function in C using the insertion sort algorithm on a doubly linked list. The function takes an element off the old list and inserts it in alphabetical order on a new list.
I'm having problems looping the function when an element is greater than (ie further along in the alphabet) the first element in the new/sorted list. Right now it is only adding elements that can be appended or prepended. Why is this?
Here is my function:
#include "sort.h"
#include <stdio.h>
#include <stdlib.h>
#include "linked_list.h"
/* sort linked list *list using merge insertion sort. */
/* upon success, the elements in *list will be sorted. */
/* return silently if *list is uninitialised or empty. */
/* the compare argument is a pointer to a function which returns */
/* less than 0, 0, or greater than 0 if first argument is */
/* less than, equal to, or greater than second argument respectively. */
void insertion_sort(LinkedList *list, int (*compare)(void *, void *))
{
void *d, *B_node_data;
LinkedList *B;
Node *B_node, *new_node;
if(!list)
return;
if(!list->head)
return;
B = initialise_linked_list();
d = list->head->data;
remove_head_linked_list(list);
prepend_linked_list(B, d);
while(list->head)
{
d = list->head->data;
B_node = B->head;
remove_head_linked_list(list);
while(B_node)
{
B_node_data = B_node->data;
if((*compare)(d, B_node_data) <= 0)
{
if(B_node->prev)
{
new_node = initialise_node();
new_node->next = B_node;
new_node->prev = B_node->prev;
new_node->data = d;
break;
}
if(B->head->data == B_node_data)
{
prepend_linked_list(B, d);
break;
}
}
if(!B_node->next)
append_linked_list(B, d);
else{
B_node = B_node->next;
}
}
}
list->head = B->head;
list->tail = B->tail;
B->head = NULL;
B->tail = NULL;
free_linked_list(B);
}
here is the definitions of the functions i called from another file:
#include "linked_list.h"
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
Node *initialise_node(void)
{
Node *node;
if(!(node = (Node *) malloc(sizeof(Node)))) {
fprintf(stderr, "error: unable to initialise node.n");
exit(EXIT_FAILURE);
}
node->next = node->prev = node->data = NULL;
return node;
}
void free_node(Node *node)
{
if(!node)
return;
free(node);
}
LinkedList *initialise_linked_list(void)
{
LinkedList *list;
if(!(list = (LinkedList *) malloc(sizeof(LinkedList)))) {
fprintf(stderr, "error: unable to initialise linked list.n");
exit(EXIT_FAILURE);
}
list->head = list->tail = NULL;
return list;
}
void free_linked_list(LinkedList *list)
{
Node *next;
while(list->head) {
next = list->head->next;
free_node(list->head);
list->head = next;
}
free(list);
}
void append_linked_list(LinkedList *list, void *data)
{
Node *node;
node = initialise_node();
node->data = data;
node->prev = list->tail;
if(list->tail) {
list->tail->next = node;
}
list->tail = node;
if(!list->head)
list->head = node;
}
void prepend_linked_list(LinkedList *list, void *data)
{
Node *node;
node = initialise_node();
node->data = data;
node->next = list->head;
if(list->head) {
list->head->prev = node;
}
list->head = node;
if(!list->tail)
list->tail = node;
}
void remove_head_linked_list(LinkedList *list)
{
Node *head;
if(!list->head)
return;
head = list->head->next;
free(list->head);
list->head = head;
if(list->head)
list->head->prev = NULL;
else
list->tail = NULL;
}
void remove_tail_linked_list(LinkedList *list)
{
Node *tail;
if(!list->tail)
return;
tail = list->tail->prev;
free_node(list->tail);
list->tail = tail;
if(list->tail)
list->tail->next = NULL;
else
list->head = NULL;
}
here is my output
==942== Command: ./task1_test harmonious pencil push naive jittery
greedy billowy practise lock star appear record precede pen lace
==942==
appear
billowy
greedy
harmonious
pencil
push
star
==942==
==942== HEAP SUMMARY:
==942== in use at exit: 264 bytes in 11 blocks
==942== total heap usage: 35 allocs, 24 frees, 824 bytes allocated
==942==
==942== 264 bytes in 11 blocks are definitely lost in loss record 1 of 1
==942== at 0x4C29C23: malloc (vg_replace_malloc.c:299)
==942== by 0x40084E: initialise_node (linked_list.c:11)
==942== by 0x400CF1: insertion_sort (sort.c:43)
==942== by 0x400815: main (task1_test.c:33)
==942==
==942== LEAK SUMMARY:
==942== definitely lost: 264 bytes in 11 blocks
==942== indirectly lost: 0 bytes in 0 blocks
==942== possibly lost: 0 bytes in 0 blocks
==942== still reachable: 0 bytes in 0 blocks
==942== suppressed: 0 bytes in 0 blocks
==942==
c linked-list doubly-linked-list insertion-sort
c linked-list doubly-linked-list insertion-sort
edited Nov 28 '18 at 19:25
Quar
asked Nov 28 '18 at 18:50
QuarQuar
62
62
4
We need to see all the functions related to list insertion/deletion. What you've shown doesn't illustrate the problem you're having. Please edit your question and post your entire program [in a single code block]. It should compile cleanly and we should be able to download it, compile it, and run it [possibly undergdb
] if we so choose. See: stackoverflow.com/help/mcve
– Craig Estey
Nov 28 '18 at 19:02
add a comment |
4
We need to see all the functions related to list insertion/deletion. What you've shown doesn't illustrate the problem you're having. Please edit your question and post your entire program [in a single code block]. It should compile cleanly and we should be able to download it, compile it, and run it [possibly undergdb
] if we so choose. See: stackoverflow.com/help/mcve
– Craig Estey
Nov 28 '18 at 19:02
4
4
We need to see all the functions related to list insertion/deletion. What you've shown doesn't illustrate the problem you're having. Please edit your question and post your entire program [in a single code block]. It should compile cleanly and we should be able to download it, compile it, and run it [possibly under
gdb
] if we so choose. See: stackoverflow.com/help/mcve– Craig Estey
Nov 28 '18 at 19:02
We need to see all the functions related to list insertion/deletion. What you've shown doesn't illustrate the problem you're having. Please edit your question and post your entire program [in a single code block]. It should compile cleanly and we should be able to download it, compile it, and run it [possibly under
gdb
] if we so choose. See: stackoverflow.com/help/mcve– Craig Estey
Nov 28 '18 at 19:02
add a comment |
1 Answer
1
active
oldest
votes
I think you're doing a lot of unnecessary alloc/free.
When taking an item off the original list, it doesn't need to be deallocated, just adjust the head
pointer.
When the insertion point is found, this node can be inserted directly into the destination list.
This simplifies the code quite a bit. But, your base list primitives aren't suitable for this type of node transfer from list to list.
I've coded up a few functions that can work directly on insertion of existing node pointers into a list.
I also refactored the sort function to use these.
NOTE: Because you didn't post all of your code, I didn't have a convenient way to test this. It will compile, but otherwise, no guarantees. Hopefully, it will help you get further.
void
insert_list_tail(LinkedList *list,Node *new)
{
Node *prev;
prev = list->tail;
if (prev != NULL)
prev->next = new;
new->next = NULL;
new->prev = prev;
list->tail = new;
if (list->head == prev)
list->head = new;
}
void
insert_list_head(LinkedList *list,Node *new)
{
Node *next;
next = list->head;
if (next != NULL)
next->prev = new;
new->prev = NULL;
new->next = next;
list->head = new;
if (list->tail == next)
list->tail = new;
}
void
insert_list_before(LinkedList *list,Node *cur,Node *new)
{
Node *next;
Node *prev;
do {
// we got to the end of the list without an insert before point, so
// append to tail of list
if (cur == NULL) {
insert_list_tail(list,new);
break;
}
// we do insert after [internally here] so get the previous node
cur = cur->prev;
// with no previous node insert at head of list
if (cur == NULL) {
insert_list_head(list,new);
break;
}
prev = cur->prev;
next = cur->next;
if (prev != NULL)
prev->next = new;
else
list->head = new;
if (next != NULL)
next->prev = new;
else
list->tail = new;
new->prev = prev;
new->next = next;
} while (0);
}
void
insertion_sort(LinkedList *slist, int (*compare)(void *,void *))
{
LinkedList list;
LinkedList *dlist = &list;
Node *src;
Node *dst;
if (slist == NULL)
return;
if (slist->head == NULL)
return;
dlist->head = NULL;
dlist->tail = NULL;
// grab first source node and insert it at destination list head
src = slist->head;
slist->head = src->next;
insert_list_head(dlist,src);
while (1) {
src = slist->head;
if (src == NULL)
break;
slist->head = src->next;
// find node to do an insertion before (i.e. find the first destination
// node that is higher than the source node)
for (dst = dlist->head; dst != NULL; dst = dst->next) {
if (compare(dst->data,src->data) > 0)
break;
}
insert_list_before(dlist,dst,src);
}
slist->head = dlist->head;
slist->tail = dlist->tail;
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53526190%2finsertion-sort-algorithm-using-doubly-linked-lists-in-c-inserting-nodes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think you're doing a lot of unnecessary alloc/free.
When taking an item off the original list, it doesn't need to be deallocated, just adjust the head
pointer.
When the insertion point is found, this node can be inserted directly into the destination list.
This simplifies the code quite a bit. But, your base list primitives aren't suitable for this type of node transfer from list to list.
I've coded up a few functions that can work directly on insertion of existing node pointers into a list.
I also refactored the sort function to use these.
NOTE: Because you didn't post all of your code, I didn't have a convenient way to test this. It will compile, but otherwise, no guarantees. Hopefully, it will help you get further.
void
insert_list_tail(LinkedList *list,Node *new)
{
Node *prev;
prev = list->tail;
if (prev != NULL)
prev->next = new;
new->next = NULL;
new->prev = prev;
list->tail = new;
if (list->head == prev)
list->head = new;
}
void
insert_list_head(LinkedList *list,Node *new)
{
Node *next;
next = list->head;
if (next != NULL)
next->prev = new;
new->prev = NULL;
new->next = next;
list->head = new;
if (list->tail == next)
list->tail = new;
}
void
insert_list_before(LinkedList *list,Node *cur,Node *new)
{
Node *next;
Node *prev;
do {
// we got to the end of the list without an insert before point, so
// append to tail of list
if (cur == NULL) {
insert_list_tail(list,new);
break;
}
// we do insert after [internally here] so get the previous node
cur = cur->prev;
// with no previous node insert at head of list
if (cur == NULL) {
insert_list_head(list,new);
break;
}
prev = cur->prev;
next = cur->next;
if (prev != NULL)
prev->next = new;
else
list->head = new;
if (next != NULL)
next->prev = new;
else
list->tail = new;
new->prev = prev;
new->next = next;
} while (0);
}
void
insertion_sort(LinkedList *slist, int (*compare)(void *,void *))
{
LinkedList list;
LinkedList *dlist = &list;
Node *src;
Node *dst;
if (slist == NULL)
return;
if (slist->head == NULL)
return;
dlist->head = NULL;
dlist->tail = NULL;
// grab first source node and insert it at destination list head
src = slist->head;
slist->head = src->next;
insert_list_head(dlist,src);
while (1) {
src = slist->head;
if (src == NULL)
break;
slist->head = src->next;
// find node to do an insertion before (i.e. find the first destination
// node that is higher than the source node)
for (dst = dlist->head; dst != NULL; dst = dst->next) {
if (compare(dst->data,src->data) > 0)
break;
}
insert_list_before(dlist,dst,src);
}
slist->head = dlist->head;
slist->tail = dlist->tail;
}
add a comment |
I think you're doing a lot of unnecessary alloc/free.
When taking an item off the original list, it doesn't need to be deallocated, just adjust the head
pointer.
When the insertion point is found, this node can be inserted directly into the destination list.
This simplifies the code quite a bit. But, your base list primitives aren't suitable for this type of node transfer from list to list.
I've coded up a few functions that can work directly on insertion of existing node pointers into a list.
I also refactored the sort function to use these.
NOTE: Because you didn't post all of your code, I didn't have a convenient way to test this. It will compile, but otherwise, no guarantees. Hopefully, it will help you get further.
void
insert_list_tail(LinkedList *list,Node *new)
{
Node *prev;
prev = list->tail;
if (prev != NULL)
prev->next = new;
new->next = NULL;
new->prev = prev;
list->tail = new;
if (list->head == prev)
list->head = new;
}
void
insert_list_head(LinkedList *list,Node *new)
{
Node *next;
next = list->head;
if (next != NULL)
next->prev = new;
new->prev = NULL;
new->next = next;
list->head = new;
if (list->tail == next)
list->tail = new;
}
void
insert_list_before(LinkedList *list,Node *cur,Node *new)
{
Node *next;
Node *prev;
do {
// we got to the end of the list without an insert before point, so
// append to tail of list
if (cur == NULL) {
insert_list_tail(list,new);
break;
}
// we do insert after [internally here] so get the previous node
cur = cur->prev;
// with no previous node insert at head of list
if (cur == NULL) {
insert_list_head(list,new);
break;
}
prev = cur->prev;
next = cur->next;
if (prev != NULL)
prev->next = new;
else
list->head = new;
if (next != NULL)
next->prev = new;
else
list->tail = new;
new->prev = prev;
new->next = next;
} while (0);
}
void
insertion_sort(LinkedList *slist, int (*compare)(void *,void *))
{
LinkedList list;
LinkedList *dlist = &list;
Node *src;
Node *dst;
if (slist == NULL)
return;
if (slist->head == NULL)
return;
dlist->head = NULL;
dlist->tail = NULL;
// grab first source node and insert it at destination list head
src = slist->head;
slist->head = src->next;
insert_list_head(dlist,src);
while (1) {
src = slist->head;
if (src == NULL)
break;
slist->head = src->next;
// find node to do an insertion before (i.e. find the first destination
// node that is higher than the source node)
for (dst = dlist->head; dst != NULL; dst = dst->next) {
if (compare(dst->data,src->data) > 0)
break;
}
insert_list_before(dlist,dst,src);
}
slist->head = dlist->head;
slist->tail = dlist->tail;
}
add a comment |
I think you're doing a lot of unnecessary alloc/free.
When taking an item off the original list, it doesn't need to be deallocated, just adjust the head
pointer.
When the insertion point is found, this node can be inserted directly into the destination list.
This simplifies the code quite a bit. But, your base list primitives aren't suitable for this type of node transfer from list to list.
I've coded up a few functions that can work directly on insertion of existing node pointers into a list.
I also refactored the sort function to use these.
NOTE: Because you didn't post all of your code, I didn't have a convenient way to test this. It will compile, but otherwise, no guarantees. Hopefully, it will help you get further.
void
insert_list_tail(LinkedList *list,Node *new)
{
Node *prev;
prev = list->tail;
if (prev != NULL)
prev->next = new;
new->next = NULL;
new->prev = prev;
list->tail = new;
if (list->head == prev)
list->head = new;
}
void
insert_list_head(LinkedList *list,Node *new)
{
Node *next;
next = list->head;
if (next != NULL)
next->prev = new;
new->prev = NULL;
new->next = next;
list->head = new;
if (list->tail == next)
list->tail = new;
}
void
insert_list_before(LinkedList *list,Node *cur,Node *new)
{
Node *next;
Node *prev;
do {
// we got to the end of the list without an insert before point, so
// append to tail of list
if (cur == NULL) {
insert_list_tail(list,new);
break;
}
// we do insert after [internally here] so get the previous node
cur = cur->prev;
// with no previous node insert at head of list
if (cur == NULL) {
insert_list_head(list,new);
break;
}
prev = cur->prev;
next = cur->next;
if (prev != NULL)
prev->next = new;
else
list->head = new;
if (next != NULL)
next->prev = new;
else
list->tail = new;
new->prev = prev;
new->next = next;
} while (0);
}
void
insertion_sort(LinkedList *slist, int (*compare)(void *,void *))
{
LinkedList list;
LinkedList *dlist = &list;
Node *src;
Node *dst;
if (slist == NULL)
return;
if (slist->head == NULL)
return;
dlist->head = NULL;
dlist->tail = NULL;
// grab first source node and insert it at destination list head
src = slist->head;
slist->head = src->next;
insert_list_head(dlist,src);
while (1) {
src = slist->head;
if (src == NULL)
break;
slist->head = src->next;
// find node to do an insertion before (i.e. find the first destination
// node that is higher than the source node)
for (dst = dlist->head; dst != NULL; dst = dst->next) {
if (compare(dst->data,src->data) > 0)
break;
}
insert_list_before(dlist,dst,src);
}
slist->head = dlist->head;
slist->tail = dlist->tail;
}
I think you're doing a lot of unnecessary alloc/free.
When taking an item off the original list, it doesn't need to be deallocated, just adjust the head
pointer.
When the insertion point is found, this node can be inserted directly into the destination list.
This simplifies the code quite a bit. But, your base list primitives aren't suitable for this type of node transfer from list to list.
I've coded up a few functions that can work directly on insertion of existing node pointers into a list.
I also refactored the sort function to use these.
NOTE: Because you didn't post all of your code, I didn't have a convenient way to test this. It will compile, but otherwise, no guarantees. Hopefully, it will help you get further.
void
insert_list_tail(LinkedList *list,Node *new)
{
Node *prev;
prev = list->tail;
if (prev != NULL)
prev->next = new;
new->next = NULL;
new->prev = prev;
list->tail = new;
if (list->head == prev)
list->head = new;
}
void
insert_list_head(LinkedList *list,Node *new)
{
Node *next;
next = list->head;
if (next != NULL)
next->prev = new;
new->prev = NULL;
new->next = next;
list->head = new;
if (list->tail == next)
list->tail = new;
}
void
insert_list_before(LinkedList *list,Node *cur,Node *new)
{
Node *next;
Node *prev;
do {
// we got to the end of the list without an insert before point, so
// append to tail of list
if (cur == NULL) {
insert_list_tail(list,new);
break;
}
// we do insert after [internally here] so get the previous node
cur = cur->prev;
// with no previous node insert at head of list
if (cur == NULL) {
insert_list_head(list,new);
break;
}
prev = cur->prev;
next = cur->next;
if (prev != NULL)
prev->next = new;
else
list->head = new;
if (next != NULL)
next->prev = new;
else
list->tail = new;
new->prev = prev;
new->next = next;
} while (0);
}
void
insertion_sort(LinkedList *slist, int (*compare)(void *,void *))
{
LinkedList list;
LinkedList *dlist = &list;
Node *src;
Node *dst;
if (slist == NULL)
return;
if (slist->head == NULL)
return;
dlist->head = NULL;
dlist->tail = NULL;
// grab first source node and insert it at destination list head
src = slist->head;
slist->head = src->next;
insert_list_head(dlist,src);
while (1) {
src = slist->head;
if (src == NULL)
break;
slist->head = src->next;
// find node to do an insertion before (i.e. find the first destination
// node that is higher than the source node)
for (dst = dlist->head; dst != NULL; dst = dst->next) {
if (compare(dst->data,src->data) > 0)
break;
}
insert_list_before(dlist,dst,src);
}
slist->head = dlist->head;
slist->tail = dlist->tail;
}
edited Nov 28 '18 at 23:50
answered Nov 28 '18 at 23:02
Craig EsteyCraig Estey
15.3k21131
15.3k21131
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53526190%2finsertion-sort-algorithm-using-doubly-linked-lists-in-c-inserting-nodes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
O4lUhYyqB Rx39T8A5GPfGLk,olVblQ,f
4
We need to see all the functions related to list insertion/deletion. What you've shown doesn't illustrate the problem you're having. Please edit your question and post your entire program [in a single code block]. It should compile cleanly and we should be able to download it, compile it, and run it [possibly under
gdb
] if we so choose. See: stackoverflow.com/help/mcve– Craig Estey
Nov 28 '18 at 19:02