What is the difference between super().__repr__() and repr(super())?
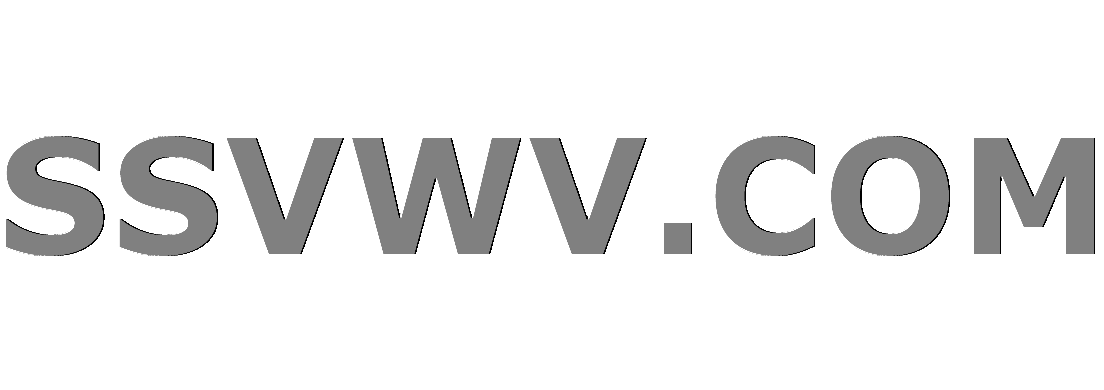
Multi tool use
In python (3.5.2), I was expecting the repr(obj)
function to call the magic method __repr__()
of obj
's class.
However calling both of them do not seem to yield the same result. Can anyone explain why ?
Sample code :
class parent:
def __init__(self):
self.a = "haha"
def __repr__(self):
return repr(self.a)
class child(parent):
def __init__(self):
super().__init__()
self.b="bebe"
def __repr__(self):
return "("+super().__repr__()+", "+repr(super())+", "+self.b+")"
def print1(self):
print("super().__repr__() returns:", super().__repr__())
print("repr(super()) returns:", repr(super()))
print("plom(super()).__repr__() returns:", plom(super()).__repr__())
print("repr(plom(super())) returns:", repr(plom(super())))
def plom(var):
return var
t=child()
print(t.__repr__())
print(repr(t))
print('-----')
t.print1()
print('-----')
print(plom(t).__repr__())
print(repr(plom(t)))
result :
>>>
RESTART: test super.py
('haha', <super: <class 'child'>, <child object>>, bebe)
('haha', <super: <class 'child'>, <child object>>, bebe)
-----
super().__repr__() returns: 'haha'
repr(super()) returns: <super: <class 'child'>, <child object>>
plom(super()).__repr__() returns: 'haha'
repr(plom(super())) returns: <super: <class 'child'>, <child object>>
-----
('haha', <super: <class 'child'>, <child object>>, bebe)
('haha', <super: <class 'child'>, <child object>>, bebe)
>>>
python python-3.x class super repr
add a comment |
In python (3.5.2), I was expecting the repr(obj)
function to call the magic method __repr__()
of obj
's class.
However calling both of them do not seem to yield the same result. Can anyone explain why ?
Sample code :
class parent:
def __init__(self):
self.a = "haha"
def __repr__(self):
return repr(self.a)
class child(parent):
def __init__(self):
super().__init__()
self.b="bebe"
def __repr__(self):
return "("+super().__repr__()+", "+repr(super())+", "+self.b+")"
def print1(self):
print("super().__repr__() returns:", super().__repr__())
print("repr(super()) returns:", repr(super()))
print("plom(super()).__repr__() returns:", plom(super()).__repr__())
print("repr(plom(super())) returns:", repr(plom(super())))
def plom(var):
return var
t=child()
print(t.__repr__())
print(repr(t))
print('-----')
t.print1()
print('-----')
print(plom(t).__repr__())
print(repr(plom(t)))
result :
>>>
RESTART: test super.py
('haha', <super: <class 'child'>, <child object>>, bebe)
('haha', <super: <class 'child'>, <child object>>, bebe)
-----
super().__repr__() returns: 'haha'
repr(super()) returns: <super: <class 'child'>, <child object>>
plom(super()).__repr__() returns: 'haha'
repr(plom(super())) returns: <super: <class 'child'>, <child object>>
-----
('haha', <super: <class 'child'>, <child object>>, bebe)
('haha', <super: <class 'child'>, <child object>>, bebe)
>>>
python python-3.x class super repr
Becausesuper
returns a proxy object, not the class itself.
– juanpa.arrivillaga
Nov 24 '18 at 2:31
How can I get the superclass from my object itself from it, then ? and why does it work with__repr__()
? In what way isrepr
different from__repr__
?
– Camion
Nov 24 '18 at 2:32
add a comment |
In python (3.5.2), I was expecting the repr(obj)
function to call the magic method __repr__()
of obj
's class.
However calling both of them do not seem to yield the same result. Can anyone explain why ?
Sample code :
class parent:
def __init__(self):
self.a = "haha"
def __repr__(self):
return repr(self.a)
class child(parent):
def __init__(self):
super().__init__()
self.b="bebe"
def __repr__(self):
return "("+super().__repr__()+", "+repr(super())+", "+self.b+")"
def print1(self):
print("super().__repr__() returns:", super().__repr__())
print("repr(super()) returns:", repr(super()))
print("plom(super()).__repr__() returns:", plom(super()).__repr__())
print("repr(plom(super())) returns:", repr(plom(super())))
def plom(var):
return var
t=child()
print(t.__repr__())
print(repr(t))
print('-----')
t.print1()
print('-----')
print(plom(t).__repr__())
print(repr(plom(t)))
result :
>>>
RESTART: test super.py
('haha', <super: <class 'child'>, <child object>>, bebe)
('haha', <super: <class 'child'>, <child object>>, bebe)
-----
super().__repr__() returns: 'haha'
repr(super()) returns: <super: <class 'child'>, <child object>>
plom(super()).__repr__() returns: 'haha'
repr(plom(super())) returns: <super: <class 'child'>, <child object>>
-----
('haha', <super: <class 'child'>, <child object>>, bebe)
('haha', <super: <class 'child'>, <child object>>, bebe)
>>>
python python-3.x class super repr
In python (3.5.2), I was expecting the repr(obj)
function to call the magic method __repr__()
of obj
's class.
However calling both of them do not seem to yield the same result. Can anyone explain why ?
Sample code :
class parent:
def __init__(self):
self.a = "haha"
def __repr__(self):
return repr(self.a)
class child(parent):
def __init__(self):
super().__init__()
self.b="bebe"
def __repr__(self):
return "("+super().__repr__()+", "+repr(super())+", "+self.b+")"
def print1(self):
print("super().__repr__() returns:", super().__repr__())
print("repr(super()) returns:", repr(super()))
print("plom(super()).__repr__() returns:", plom(super()).__repr__())
print("repr(plom(super())) returns:", repr(plom(super())))
def plom(var):
return var
t=child()
print(t.__repr__())
print(repr(t))
print('-----')
t.print1()
print('-----')
print(plom(t).__repr__())
print(repr(plom(t)))
result :
>>>
RESTART: test super.py
('haha', <super: <class 'child'>, <child object>>, bebe)
('haha', <super: <class 'child'>, <child object>>, bebe)
-----
super().__repr__() returns: 'haha'
repr(super()) returns: <super: <class 'child'>, <child object>>
plom(super()).__repr__() returns: 'haha'
repr(plom(super())) returns: <super: <class 'child'>, <child object>>
-----
('haha', <super: <class 'child'>, <child object>>, bebe)
('haha', <super: <class 'child'>, <child object>>, bebe)
>>>
python python-3.x class super repr
python python-3.x class super repr
edited Nov 24 '18 at 2:32
Camion
asked Nov 24 '18 at 1:37
CamionCamion
17410
17410
Becausesuper
returns a proxy object, not the class itself.
– juanpa.arrivillaga
Nov 24 '18 at 2:31
How can I get the superclass from my object itself from it, then ? and why does it work with__repr__()
? In what way isrepr
different from__repr__
?
– Camion
Nov 24 '18 at 2:32
add a comment |
Becausesuper
returns a proxy object, not the class itself.
– juanpa.arrivillaga
Nov 24 '18 at 2:31
How can I get the superclass from my object itself from it, then ? and why does it work with__repr__()
? In what way isrepr
different from__repr__
?
– Camion
Nov 24 '18 at 2:32
Because
super
returns a proxy object, not the class itself.– juanpa.arrivillaga
Nov 24 '18 at 2:31
Because
super
returns a proxy object, not the class itself.– juanpa.arrivillaga
Nov 24 '18 at 2:31
How can I get the superclass from my object itself from it, then ? and why does it work with
__repr__()
? In what way is repr
different from __repr__
?– Camion
Nov 24 '18 at 2:32
How can I get the superclass from my object itself from it, then ? and why does it work with
__repr__()
? In what way is repr
different from __repr__
?– Camion
Nov 24 '18 at 2:32
add a comment |
3 Answers
3
active
oldest
votes
Calling repr(super())
directly accesses the __repr__
on the super
class (technically, the tp_repr
of the C PyTypeObject
struct defining the super
type). Most special dunder methods behave this way when called implicitly (as opposed to explicitly calling them as methods). repr(x)
isn't equivalent to x.__repr__()
. You can think of repr
as being defined as:
def repr(obj):
return type(obj).__repr__(obj) # Call unbound function of class with instance as arg
while you were expecting it to be:
def repr(obj):
return obj.__repr__() # Call bound method of instance
This behavior is intentional; one, customizing dunder methods per-instance makes little sense, and two, prohibiting it allows for much more efficient code at the C level (it has much faster ways of doing what the illustrative methods above do).
By contrast, super().__repr__()
looks up the method on the super
instance, and super
defines a custom tp_getattro
(roughly equivalent to defining a custom __getattribute__
method), which means lookups on the instance are intercepted before they find the tp_repr
/__repr__
of the class, and instead are dispatched through the custom attribute getter (which performs the superclass delegation).
add a comment |
If you consult the docs, you'll see that super
returns a proxy object which delegates method calls to the appropriate class according to method resolution order.
So repr(super())
gets you the representation of the proxy object. Whereas the method call super().__repr__()
gives you the representation defined by the next class in the method resolution order.
If you want the superclass itself, try
my_object.__mro__[1]
add a comment |
In super().__repr__()
you're calling the repr class of the super object so you get 'haha'
In the second, you're calling repr of the super()
. what does super()
output? <super: <class 'child'>, <child object>>
so you're effectively calling repr on some class hierarchy
This doesn't make any sense to me : Why would super() output something different between super().__repr__() and repr(super()) ?
– Camion
Nov 24 '18 at 2:15
I added some cases and I don't understand whysuper()
would make that difference when myplom(t)
function doesn't. Isn't super a regular function ?
– Camion
Nov 24 '18 at 2:26
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454458%2fwhat-is-the-difference-between-super-repr-and-reprsuper%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Calling repr(super())
directly accesses the __repr__
on the super
class (technically, the tp_repr
of the C PyTypeObject
struct defining the super
type). Most special dunder methods behave this way when called implicitly (as opposed to explicitly calling them as methods). repr(x)
isn't equivalent to x.__repr__()
. You can think of repr
as being defined as:
def repr(obj):
return type(obj).__repr__(obj) # Call unbound function of class with instance as arg
while you were expecting it to be:
def repr(obj):
return obj.__repr__() # Call bound method of instance
This behavior is intentional; one, customizing dunder methods per-instance makes little sense, and two, prohibiting it allows for much more efficient code at the C level (it has much faster ways of doing what the illustrative methods above do).
By contrast, super().__repr__()
looks up the method on the super
instance, and super
defines a custom tp_getattro
(roughly equivalent to defining a custom __getattribute__
method), which means lookups on the instance are intercepted before they find the tp_repr
/__repr__
of the class, and instead are dispatched through the custom attribute getter (which performs the superclass delegation).
add a comment |
Calling repr(super())
directly accesses the __repr__
on the super
class (technically, the tp_repr
of the C PyTypeObject
struct defining the super
type). Most special dunder methods behave this way when called implicitly (as opposed to explicitly calling them as methods). repr(x)
isn't equivalent to x.__repr__()
. You can think of repr
as being defined as:
def repr(obj):
return type(obj).__repr__(obj) # Call unbound function of class with instance as arg
while you were expecting it to be:
def repr(obj):
return obj.__repr__() # Call bound method of instance
This behavior is intentional; one, customizing dunder methods per-instance makes little sense, and two, prohibiting it allows for much more efficient code at the C level (it has much faster ways of doing what the illustrative methods above do).
By contrast, super().__repr__()
looks up the method on the super
instance, and super
defines a custom tp_getattro
(roughly equivalent to defining a custom __getattribute__
method), which means lookups on the instance are intercepted before they find the tp_repr
/__repr__
of the class, and instead are dispatched through the custom attribute getter (which performs the superclass delegation).
add a comment |
Calling repr(super())
directly accesses the __repr__
on the super
class (technically, the tp_repr
of the C PyTypeObject
struct defining the super
type). Most special dunder methods behave this way when called implicitly (as opposed to explicitly calling them as methods). repr(x)
isn't equivalent to x.__repr__()
. You can think of repr
as being defined as:
def repr(obj):
return type(obj).__repr__(obj) # Call unbound function of class with instance as arg
while you were expecting it to be:
def repr(obj):
return obj.__repr__() # Call bound method of instance
This behavior is intentional; one, customizing dunder methods per-instance makes little sense, and two, prohibiting it allows for much more efficient code at the C level (it has much faster ways of doing what the illustrative methods above do).
By contrast, super().__repr__()
looks up the method on the super
instance, and super
defines a custom tp_getattro
(roughly equivalent to defining a custom __getattribute__
method), which means lookups on the instance are intercepted before they find the tp_repr
/__repr__
of the class, and instead are dispatched through the custom attribute getter (which performs the superclass delegation).
Calling repr(super())
directly accesses the __repr__
on the super
class (technically, the tp_repr
of the C PyTypeObject
struct defining the super
type). Most special dunder methods behave this way when called implicitly (as opposed to explicitly calling them as methods). repr(x)
isn't equivalent to x.__repr__()
. You can think of repr
as being defined as:
def repr(obj):
return type(obj).__repr__(obj) # Call unbound function of class with instance as arg
while you were expecting it to be:
def repr(obj):
return obj.__repr__() # Call bound method of instance
This behavior is intentional; one, customizing dunder methods per-instance makes little sense, and two, prohibiting it allows for much more efficient code at the C level (it has much faster ways of doing what the illustrative methods above do).
By contrast, super().__repr__()
looks up the method on the super
instance, and super
defines a custom tp_getattro
(roughly equivalent to defining a custom __getattribute__
method), which means lookups on the instance are intercepted before they find the tp_repr
/__repr__
of the class, and instead are dispatched through the custom attribute getter (which performs the superclass delegation).
answered Nov 24 '18 at 2:36


ShadowRangerShadowRanger
58.7k45495
58.7k45495
add a comment |
add a comment |
If you consult the docs, you'll see that super
returns a proxy object which delegates method calls to the appropriate class according to method resolution order.
So repr(super())
gets you the representation of the proxy object. Whereas the method call super().__repr__()
gives you the representation defined by the next class in the method resolution order.
If you want the superclass itself, try
my_object.__mro__[1]
add a comment |
If you consult the docs, you'll see that super
returns a proxy object which delegates method calls to the appropriate class according to method resolution order.
So repr(super())
gets you the representation of the proxy object. Whereas the method call super().__repr__()
gives you the representation defined by the next class in the method resolution order.
If you want the superclass itself, try
my_object.__mro__[1]
add a comment |
If you consult the docs, you'll see that super
returns a proxy object which delegates method calls to the appropriate class according to method resolution order.
So repr(super())
gets you the representation of the proxy object. Whereas the method call super().__repr__()
gives you the representation defined by the next class in the method resolution order.
If you want the superclass itself, try
my_object.__mro__[1]
If you consult the docs, you'll see that super
returns a proxy object which delegates method calls to the appropriate class according to method resolution order.
So repr(super())
gets you the representation of the proxy object. Whereas the method call super().__repr__()
gives you the representation defined by the next class in the method resolution order.
If you want the superclass itself, try
my_object.__mro__[1]
edited Nov 24 '18 at 17:07
answered Nov 24 '18 at 2:37


juanpa.arrivillagajuanpa.arrivillaga
37.5k33572
37.5k33572
add a comment |
add a comment |
In super().__repr__()
you're calling the repr class of the super object so you get 'haha'
In the second, you're calling repr of the super()
. what does super()
output? <super: <class 'child'>, <child object>>
so you're effectively calling repr on some class hierarchy
This doesn't make any sense to me : Why would super() output something different between super().__repr__() and repr(super()) ?
– Camion
Nov 24 '18 at 2:15
I added some cases and I don't understand whysuper()
would make that difference when myplom(t)
function doesn't. Isn't super a regular function ?
– Camion
Nov 24 '18 at 2:26
add a comment |
In super().__repr__()
you're calling the repr class of the super object so you get 'haha'
In the second, you're calling repr of the super()
. what does super()
output? <super: <class 'child'>, <child object>>
so you're effectively calling repr on some class hierarchy
This doesn't make any sense to me : Why would super() output something different between super().__repr__() and repr(super()) ?
– Camion
Nov 24 '18 at 2:15
I added some cases and I don't understand whysuper()
would make that difference when myplom(t)
function doesn't. Isn't super a regular function ?
– Camion
Nov 24 '18 at 2:26
add a comment |
In super().__repr__()
you're calling the repr class of the super object so you get 'haha'
In the second, you're calling repr of the super()
. what does super()
output? <super: <class 'child'>, <child object>>
so you're effectively calling repr on some class hierarchy
In super().__repr__()
you're calling the repr class of the super object so you get 'haha'
In the second, you're calling repr of the super()
. what does super()
output? <super: <class 'child'>, <child object>>
so you're effectively calling repr on some class hierarchy
answered Nov 24 '18 at 2:09


Ian QuahIan Quah
625612
625612
This doesn't make any sense to me : Why would super() output something different between super().__repr__() and repr(super()) ?
– Camion
Nov 24 '18 at 2:15
I added some cases and I don't understand whysuper()
would make that difference when myplom(t)
function doesn't. Isn't super a regular function ?
– Camion
Nov 24 '18 at 2:26
add a comment |
This doesn't make any sense to me : Why would super() output something different between super().__repr__() and repr(super()) ?
– Camion
Nov 24 '18 at 2:15
I added some cases and I don't understand whysuper()
would make that difference when myplom(t)
function doesn't. Isn't super a regular function ?
– Camion
Nov 24 '18 at 2:26
This doesn't make any sense to me : Why would super() output something different between super().__repr__() and repr(super()) ?
– Camion
Nov 24 '18 at 2:15
This doesn't make any sense to me : Why would super() output something different between super().__repr__() and repr(super()) ?
– Camion
Nov 24 '18 at 2:15
I added some cases and I don't understand why
super()
would make that difference when my plom(t)
function doesn't. Isn't super a regular function ?– Camion
Nov 24 '18 at 2:26
I added some cases and I don't understand why
super()
would make that difference when my plom(t)
function doesn't. Isn't super a regular function ?– Camion
Nov 24 '18 at 2:26
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53454458%2fwhat-is-the-difference-between-super-repr-and-reprsuper%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9XmT8Y1eX e,GzIVene6eFZxsZbFrIC T0XEaB8ptiz g,O
Because
super
returns a proxy object, not the class itself.– juanpa.arrivillaga
Nov 24 '18 at 2:31
How can I get the superclass from my object itself from it, then ? and why does it work with
__repr__()
? In what way isrepr
different from__repr__
?– Camion
Nov 24 '18 at 2:32