How to “swap” two values in an array?
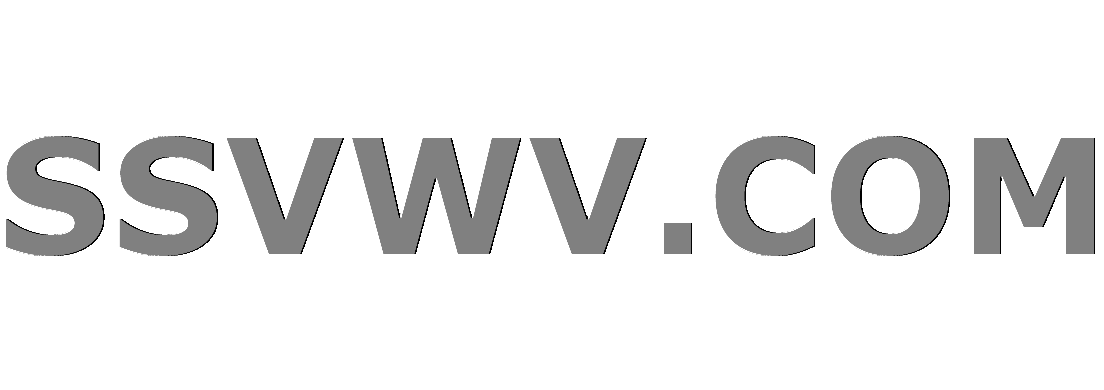
Multi tool use
I am trying to write a selection sort algorithom. As part of the algorithom I need to switch 2 values in the array, I tryed it as follows: array[min] = array[i]; array[i] = array[min];
But I belive this wont work because array[min]
will already be =
to array[i]
. So how do I do this swap? bellow is my code.
static int array = {3, 2, 1, 4, 5, 6};
static int n = 5;
static int temp;
for (int i = 0; i<=5; i++) {
int min = 0;
for (int j = i+1; j<=n; j++) {
//System.out.println(j);
if (array[j]<array[min]) {
min = j;
}
if (min != i) {
array[min] = array[i];
array[i] = array[min];
}
}
}
java selection
add a comment |
I am trying to write a selection sort algorithom. As part of the algorithom I need to switch 2 values in the array, I tryed it as follows: array[min] = array[i]; array[i] = array[min];
But I belive this wont work because array[min]
will already be =
to array[i]
. So how do I do this swap? bellow is my code.
static int array = {3, 2, 1, 4, 5, 6};
static int n = 5;
static int temp;
for (int i = 0; i<=5; i++) {
int min = 0;
for (int j = i+1; j<=n; j++) {
//System.out.println(j);
if (array[j]<array[min]) {
min = j;
}
if (min != i) {
array[min] = array[i];
array[i] = array[min];
}
}
}
java selection
add a comment |
I am trying to write a selection sort algorithom. As part of the algorithom I need to switch 2 values in the array, I tryed it as follows: array[min] = array[i]; array[i] = array[min];
But I belive this wont work because array[min]
will already be =
to array[i]
. So how do I do this swap? bellow is my code.
static int array = {3, 2, 1, 4, 5, 6};
static int n = 5;
static int temp;
for (int i = 0; i<=5; i++) {
int min = 0;
for (int j = i+1; j<=n; j++) {
//System.out.println(j);
if (array[j]<array[min]) {
min = j;
}
if (min != i) {
array[min] = array[i];
array[i] = array[min];
}
}
}
java selection
I am trying to write a selection sort algorithom. As part of the algorithom I need to switch 2 values in the array, I tryed it as follows: array[min] = array[i]; array[i] = array[min];
But I belive this wont work because array[min]
will already be =
to array[i]
. So how do I do this swap? bellow is my code.
static int array = {3, 2, 1, 4, 5, 6};
static int n = 5;
static int temp;
for (int i = 0; i<=5; i++) {
int min = 0;
for (int j = i+1; j<=n; j++) {
//System.out.println(j);
if (array[j]<array[min]) {
min = j;
}
if (min != i) {
array[min] = array[i];
array[i] = array[min];
}
}
}
java selection
java selection
edited Nov 25 '18 at 3:25
The Great Visionary
asked Nov 25 '18 at 3:20


The Great VisionaryThe Great Visionary
20117
20117
add a comment |
add a comment |
4 Answers
4
active
oldest
votes
Need a temp mem location to store a value.
i.e.
temp = array[min]
array[min] = array[i]
array[i] = temp
add a comment |
You can swap values without using temp variable too. Sample code goes like this:
For an example:
array[min] = 10;
array[i] = 5;
Now,
array[min] = array[min] + array[i]; // array[min] = 15
array[i] = array[min] - array[i]; // array[i] = 10
array[min] = array[min] - array[i]; // array[min] = 5
Here you can avoid the use of temp variable.
For the price of three operations and code readability :)
– iPirat
Nov 25 '18 at 3:45
add a comment |
There are numerous ways to swap:
With a third temporary variable
temp = array[min];
array[min] = array[i];
array[i] = temp;
Without a temporary variable (Using addition)
array[min] = array[min] + array[i];
array[i] = array[min] - array[i];
array[min] = array[min] - array[i];
Without a temporary variable (Using bit operations)
array[min] ^= array[i];
array[i] ^= array[min];
array[min] = array[i];
Here^
is the Bitwise XOR operator.
add a comment |
This looks like some fun!
Any Big 0 Constraints? Memory constraints? If not I would try this guy's simple bubble sort with the swap
public static void bubbleSort(int Database) {
for (int i = arraySize -1; i > 0; i--) {
for (int j = 0; j < i; j++) {
if(Database[j] > Database[j+1]){
Swap(Database,j,j+1);
//ArrayPartition.DisplayTheArray(i, j)
}
Main.PrintHorizontalArray(i, j);
}
// Main.PrintHorizontalArray(i, -1);
}
}
public static void Swap(int database, int j, int i) {
int temp = database[j];
database[j] = database[i];
database[i] = temp;
}
I take zero credit for this answer as sorting was not covered in my college Java classes and I googled this to learn it.
a great starting point to learn data structure and sorting.
There is also a great java 7 book for free that covers these
javanotes
I looked up my Java 7 class I used this code here
public int selectionSort(int inarray) {
//int data = Arrays.copyOf(inarray, inarray.length);
int temp;
int n=inarray.length;
for (int i=0; i<inarray.length; i++){
int k=i;
for (int j=i+1;j < n;j++)
if (inarray[j]<inarray[k])
k=j;
temp = inarray[i];
inarray[i] = inarray[k];
inarray[k] = temp;
}
return data;
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53464359%2fhow-to-swap-two-values-in-an-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Need a temp mem location to store a value.
i.e.
temp = array[min]
array[min] = array[i]
array[i] = temp
add a comment |
Need a temp mem location to store a value.
i.e.
temp = array[min]
array[min] = array[i]
array[i] = temp
add a comment |
Need a temp mem location to store a value.
i.e.
temp = array[min]
array[min] = array[i]
array[i] = temp
Need a temp mem location to store a value.
i.e.
temp = array[min]
array[min] = array[i]
array[i] = temp
edited Nov 25 '18 at 4:28
vinayawsm
678523
678523
answered Nov 25 '18 at 3:23
CodeFromthe510CodeFromthe510
1281212
1281212
add a comment |
add a comment |
You can swap values without using temp variable too. Sample code goes like this:
For an example:
array[min] = 10;
array[i] = 5;
Now,
array[min] = array[min] + array[i]; // array[min] = 15
array[i] = array[min] - array[i]; // array[i] = 10
array[min] = array[min] - array[i]; // array[min] = 5
Here you can avoid the use of temp variable.
For the price of three operations and code readability :)
– iPirat
Nov 25 '18 at 3:45
add a comment |
You can swap values without using temp variable too. Sample code goes like this:
For an example:
array[min] = 10;
array[i] = 5;
Now,
array[min] = array[min] + array[i]; // array[min] = 15
array[i] = array[min] - array[i]; // array[i] = 10
array[min] = array[min] - array[i]; // array[min] = 5
Here you can avoid the use of temp variable.
For the price of three operations and code readability :)
– iPirat
Nov 25 '18 at 3:45
add a comment |
You can swap values without using temp variable too. Sample code goes like this:
For an example:
array[min] = 10;
array[i] = 5;
Now,
array[min] = array[min] + array[i]; // array[min] = 15
array[i] = array[min] - array[i]; // array[i] = 10
array[min] = array[min] - array[i]; // array[min] = 5
Here you can avoid the use of temp variable.
You can swap values without using temp variable too. Sample code goes like this:
For an example:
array[min] = 10;
array[i] = 5;
Now,
array[min] = array[min] + array[i]; // array[min] = 15
array[i] = array[min] - array[i]; // array[i] = 10
array[min] = array[min] - array[i]; // array[min] = 5
Here you can avoid the use of temp variable.
answered Nov 25 '18 at 3:34


abj1305abj1305
18510
18510
For the price of three operations and code readability :)
– iPirat
Nov 25 '18 at 3:45
add a comment |
For the price of three operations and code readability :)
– iPirat
Nov 25 '18 at 3:45
For the price of three operations and code readability :)
– iPirat
Nov 25 '18 at 3:45
For the price of three operations and code readability :)
– iPirat
Nov 25 '18 at 3:45
add a comment |
There are numerous ways to swap:
With a third temporary variable
temp = array[min];
array[min] = array[i];
array[i] = temp;
Without a temporary variable (Using addition)
array[min] = array[min] + array[i];
array[i] = array[min] - array[i];
array[min] = array[min] - array[i];
Without a temporary variable (Using bit operations)
array[min] ^= array[i];
array[i] ^= array[min];
array[min] = array[i];
Here^
is the Bitwise XOR operator.
add a comment |
There are numerous ways to swap:
With a third temporary variable
temp = array[min];
array[min] = array[i];
array[i] = temp;
Without a temporary variable (Using addition)
array[min] = array[min] + array[i];
array[i] = array[min] - array[i];
array[min] = array[min] - array[i];
Without a temporary variable (Using bit operations)
array[min] ^= array[i];
array[i] ^= array[min];
array[min] = array[i];
Here^
is the Bitwise XOR operator.
add a comment |
There are numerous ways to swap:
With a third temporary variable
temp = array[min];
array[min] = array[i];
array[i] = temp;
Without a temporary variable (Using addition)
array[min] = array[min] + array[i];
array[i] = array[min] - array[i];
array[min] = array[min] - array[i];
Without a temporary variable (Using bit operations)
array[min] ^= array[i];
array[i] ^= array[min];
array[min] = array[i];
Here^
is the Bitwise XOR operator.
There are numerous ways to swap:
With a third temporary variable
temp = array[min];
array[min] = array[i];
array[i] = temp;
Without a temporary variable (Using addition)
array[min] = array[min] + array[i];
array[i] = array[min] - array[i];
array[min] = array[min] - array[i];
Without a temporary variable (Using bit operations)
array[min] ^= array[i];
array[i] ^= array[min];
array[min] = array[i];
Here^
is the Bitwise XOR operator.
answered Nov 25 '18 at 3:46


Lavish KothariLavish Kothari
726614
726614
add a comment |
add a comment |
This looks like some fun!
Any Big 0 Constraints? Memory constraints? If not I would try this guy's simple bubble sort with the swap
public static void bubbleSort(int Database) {
for (int i = arraySize -1; i > 0; i--) {
for (int j = 0; j < i; j++) {
if(Database[j] > Database[j+1]){
Swap(Database,j,j+1);
//ArrayPartition.DisplayTheArray(i, j)
}
Main.PrintHorizontalArray(i, j);
}
// Main.PrintHorizontalArray(i, -1);
}
}
public static void Swap(int database, int j, int i) {
int temp = database[j];
database[j] = database[i];
database[i] = temp;
}
I take zero credit for this answer as sorting was not covered in my college Java classes and I googled this to learn it.
a great starting point to learn data structure and sorting.
There is also a great java 7 book for free that covers these
javanotes
I looked up my Java 7 class I used this code here
public int selectionSort(int inarray) {
//int data = Arrays.copyOf(inarray, inarray.length);
int temp;
int n=inarray.length;
for (int i=0; i<inarray.length; i++){
int k=i;
for (int j=i+1;j < n;j++)
if (inarray[j]<inarray[k])
k=j;
temp = inarray[i];
inarray[i] = inarray[k];
inarray[k] = temp;
}
return data;
}
add a comment |
This looks like some fun!
Any Big 0 Constraints? Memory constraints? If not I would try this guy's simple bubble sort with the swap
public static void bubbleSort(int Database) {
for (int i = arraySize -1; i > 0; i--) {
for (int j = 0; j < i; j++) {
if(Database[j] > Database[j+1]){
Swap(Database,j,j+1);
//ArrayPartition.DisplayTheArray(i, j)
}
Main.PrintHorizontalArray(i, j);
}
// Main.PrintHorizontalArray(i, -1);
}
}
public static void Swap(int database, int j, int i) {
int temp = database[j];
database[j] = database[i];
database[i] = temp;
}
I take zero credit for this answer as sorting was not covered in my college Java classes and I googled this to learn it.
a great starting point to learn data structure and sorting.
There is also a great java 7 book for free that covers these
javanotes
I looked up my Java 7 class I used this code here
public int selectionSort(int inarray) {
//int data = Arrays.copyOf(inarray, inarray.length);
int temp;
int n=inarray.length;
for (int i=0; i<inarray.length; i++){
int k=i;
for (int j=i+1;j < n;j++)
if (inarray[j]<inarray[k])
k=j;
temp = inarray[i];
inarray[i] = inarray[k];
inarray[k] = temp;
}
return data;
}
add a comment |
This looks like some fun!
Any Big 0 Constraints? Memory constraints? If not I would try this guy's simple bubble sort with the swap
public static void bubbleSort(int Database) {
for (int i = arraySize -1; i > 0; i--) {
for (int j = 0; j < i; j++) {
if(Database[j] > Database[j+1]){
Swap(Database,j,j+1);
//ArrayPartition.DisplayTheArray(i, j)
}
Main.PrintHorizontalArray(i, j);
}
// Main.PrintHorizontalArray(i, -1);
}
}
public static void Swap(int database, int j, int i) {
int temp = database[j];
database[j] = database[i];
database[i] = temp;
}
I take zero credit for this answer as sorting was not covered in my college Java classes and I googled this to learn it.
a great starting point to learn data structure and sorting.
There is also a great java 7 book for free that covers these
javanotes
I looked up my Java 7 class I used this code here
public int selectionSort(int inarray) {
//int data = Arrays.copyOf(inarray, inarray.length);
int temp;
int n=inarray.length;
for (int i=0; i<inarray.length; i++){
int k=i;
for (int j=i+1;j < n;j++)
if (inarray[j]<inarray[k])
k=j;
temp = inarray[i];
inarray[i] = inarray[k];
inarray[k] = temp;
}
return data;
}
This looks like some fun!
Any Big 0 Constraints? Memory constraints? If not I would try this guy's simple bubble sort with the swap
public static void bubbleSort(int Database) {
for (int i = arraySize -1; i > 0; i--) {
for (int j = 0; j < i; j++) {
if(Database[j] > Database[j+1]){
Swap(Database,j,j+1);
//ArrayPartition.DisplayTheArray(i, j)
}
Main.PrintHorizontalArray(i, j);
}
// Main.PrintHorizontalArray(i, -1);
}
}
public static void Swap(int database, int j, int i) {
int temp = database[j];
database[j] = database[i];
database[i] = temp;
}
I take zero credit for this answer as sorting was not covered in my college Java classes and I googled this to learn it.
a great starting point to learn data structure and sorting.
There is also a great java 7 book for free that covers these
javanotes
I looked up my Java 7 class I used this code here
public int selectionSort(int inarray) {
//int data = Arrays.copyOf(inarray, inarray.length);
int temp;
int n=inarray.length;
for (int i=0; i<inarray.length; i++){
int k=i;
for (int j=i+1;j < n;j++)
if (inarray[j]<inarray[k])
k=j;
temp = inarray[i];
inarray[i] = inarray[k];
inarray[k] = temp;
}
return data;
}
edited Nov 25 '18 at 4:31
answered Nov 25 '18 at 3:48


Jeremiah StillingsJeremiah Stillings
4861315
4861315
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53464359%2fhow-to-swap-two-values-in-an-array%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
OKsXmhoBEQKn0BAYBZBspuUo